jQuery Plugin For Selecting Multiple Elements - Multiple Select
File Size: | 662 KB |
---|---|
Views Total: | 91405 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
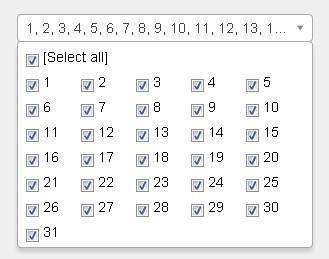
Multiple Select is a useful jQuery plugin that allows you to select multiple elements by clicking the checkboxes in a select drop down list.
More features:
- Live filtering.
- Supports the native select element.
- Supports both single and multiple selection.
- Supports both static and dynamic data.
- Multiple languages.
- Bootstrap 3/4 themes.
- Useful options, methods, and events.
See also:
- jQuery Multiple Select Box Plugin
- jQuery Multiple Select Element Replacement Plugin - selectlist
- 10 Best Multiple Select jQuery Plugins
How to use it:
1. Include jQuery library and jQuery Multiple Select on your web page
<script src="jquery.min.js"></script> <script src="jquery.multiple.select.js"></script>
2. Include jQuery Multiple Select CSS to style the select box
<link href="multiple-select.css" rel="stylesheet" type="text/css">
3. Create a stardard html select box.
<select id="demo"> <option value="1">1</option> <option value="2">2</option> <option value="3">3</option> ... </select>
4. Call the plugin on the select element and done.
$(function() { $("#demo").multipleSelect({ // options here }); });
5. You're also allowed to define the options in the JavaScript:
$(function() { $("#demo").multipleSelect({ data: [ { text: 'January', value: 1 }, { text: 'February', value: 2 }, { text: 'March', value: 3 } ] }) }); $(function() { $("#demo").multipleSelect({ data: [ { type: 'optgroup', label: 'Group 1', children: [ { text: 'January', value: 1 }, { text: 'February', value: 2 }, { text: 'March', value: 3 } ] }, { type: 'optgroup', label: 'Group 2', children: [ { text: 'July', value: 7 }, { text: 'August', value: 8 }, { text: 'September', value: 9 } ] } ] }) });
6. All possible options to customize the plugin.
$("#demo").multipleSelect({ // placeholder text placeholder: '', // an array of data data: [], // e.g. 'en-us' locale: undefined, // displays the Select All checkbox selectAll: true, // allows to select a single option single: false, // shows a radio button when single is set to true singleRadio: false, // allows multiple selection multiple: false, // hides checkboxes for Optgroup hideOptgroupCheckboxes: false, // width of the item multipleWidth: 80, // width width: undefined, // dropdown width dropWidth: undefined, // max height maxHeight: 250, maxHeightUnit: 'px', // or 'top' position: 'bottom', displayHtml: false, // displays selected values displayValues: false, // displays titles displayTitle: false, // custom delimiter displayDelimiter: ', ', // used to display how many items are selected minimumCountSelected: 3, // add ... after selected options ellipsis: false, // is opened on init isOpen: false, // keeps the select always be opened keepOpen: false, // opens the select on hover openOnHover: false, // container element container: null, // displays a search box to filter through the options filter: false, // allows to search through optgroups filterGroup: false, // placeholder text filterPlaceholder: '', // selects the option on enter filterAcceptOnEnter: false, // filter by data length filterByDataLength: undefined, // shows clear icon showClear: false, // animation type animate: 'none', // custom filter function customFilter: function customFilter(label, text) { // originalLabel, originalText return label.includes(text); }, formatSelectAll: function () { return '[Select all]'; }, formatAllSelected: function () { return 'All selected'; }, formatCountSelected: function () { return '# of % selected'; }, formatNoMatchesFound: function () { return 'No matches found'; }, styler: function () { return false; }, textTemplate: function ($elm) { return $elm.html(); }, labelTemplate: function ($elm) { return $elm.attr('label'); } });
7. Available callback functions.
$("#demo").multipleSelect({ onOpen: function () { return false; }, onClose: function () { return false; }, onCheckAll: function () { return false; }, onUncheckAll: function () { return false; }, onFocus: function () { return false; }, onBlur: function () { return false; }, onOptgroupClick: function (view) { return false; }, onClick: function (view) { return false; }, onFilter: function () { return false; }, onAfterCreate: function () { return false; } });
8 Events.
- onOpen: Fires when the dropdown list is open.
- onClose: Fires when the dropdown list is close.
- onCheckAll: Fires when all the options are checked by either clicking the “Select all” checkbox, or when the “checkall” method is programatically called.
- onUncheckAll:
- onFocus: Bind an event handler to the “focus”.
- onBlur: Bind an event handler to the “blur”
- onOptgroupClick: Fires when a an optgroup label is clicked on.
- onBeforeClick: Fires before a checkbox is clicked
- onClick: Fires when a checkbox is checked or unchecked.
$('#demo').multipleSelect({ onEventName: function (arg1, arg2, ...) { // ... } })
9. API methods.
// Gets the selected values. alert('Selected values: ' + $('select').multipleSelect('getSelects')); alert('Selected texts: ' + $('select').multipleSelect('getSelects', 'text')); // Sets the selected values. $('select').multipleSelect('setSelects', [1, 3]); // Enables Select. $('select').multipleSelect('enable'); // Disables Select. During this mode the user is not allowed to manipulate the selection. $('select').multipleSelect('disable'); // Check all checkboxes. $('select').multipleSelect('checkAll'); // Uncheck all checkboxes. $('select').multipleSelect('uncheckAll'); // Focus the multiple select. $('select').multipleSelect('focus'); // Blurs the multiple select. $('select').multipleSelect('blur'); // Reloads the Multiple Select. $('select').multipleSelect('refresh'); // Returns options object. $('select').multipleSelect('getOptions'); // Updates options $('select').multipleSelect('refreshOptions', Options); // Destroys the multiple select $('select').multipleSelect('destroy');
Changelog:
v1.7.0 (2023-11-23)
- New: Added onBeforeClick event.
- New: Added $.fn.multipleSelect.Constructor to support extension.
- New: Added keyboard actions.
- New: Added ja-JP locale.
- Update: Updated onFilter event parameter to original search.
v1.6.0 (2023-05-31)
- Added setSelects by text support.
- Added divider option support.
- Added classes, classPrefix and size options.
- Added dropdown support for bootstrap theme.
- Added class for ms-parent when open the select.
- Added getData method.
- Added fr-FR, vi-VN, ru-RU, hu-HU, da-DK, pt-BR and cz-CS locales.
- Added index.d.ts to support TypeScript.
- Fixed one item of group selected bug.
- Fixed not check group with no children bug.
- Fixed clear button display error.
- Fixed init multiple times bug in vue component.
- Updated font-size to use percent instead.
- Updated the default parameter of customFilter option.
- Updated the BLOCK_ROWS to 500.
v1.5.2 (2019-11-13)
- Update: Fixed virtual scroll bottom select bug.
- Update: Fixed element not destroyed bug using v-if.
v1.5.1 (2019-11-05)
- New: Added watch slot change supported.
- Update: Fixed v-model not check all bug.
- Update: Fixed document event trigger multiple times bug.
v1.5.0 (2019-10-28)
- New: Rewrote display and data logic code.
- New: Added auto single or multiple support.
- New: Added virtual scroll for large data.
- New: Added object value support.
- New: Added object for v-model.
- New: Added data attributes for optgroup and option.
- New: Added tabindex attribute.
- New: Added showClear option and onClear event.
- New: Added maxHeightUnit option.
- New: Added it-IT locale.
- Update: Improved events trigger logic.
- Update: Updated the single group style.
- Update: Updated parameters of onOptgroupClick and onClick events.
- Update: Fixed vue warning when data is Object.
- Update: Removed displayHtml option and display html by default.
v1.4.3 (2019-10-22)
- New: Added object support for data option.
- Update: Updated vue-es build filename.
- Update: Updated event name to lowercase hyphen format of vue component.
- Update: Fixed defaultValue error without v-model.
- Update: Fixed uncheck all cannot trigger change bug.
- Update: Fixed v-model not work bug when props changed.
- Update: Fixed min version throw cannot convert object to primitive value error.
- Update: Removed label spaces of customFilter params.
- Update: Removed text spaces of the option label.
v1.4.2 (2019-10-10)
- New: Added string/number array support for data option.
- New: Added required attribute support.
- New: Added bootstrap(v3, v4) themes support.
- Update: Improved onAfterCreate event.
- Update: Fixed destroyed bug of vue component.
- Update: Fixed display bug of only one member of group.
- Update: Fixed single group filter bug.
v1.4.1 (2019-09-24)
- Added data support.
v1.4.0 (2019-09-01)
- Added data support.
- Added vue component.
- Added locale support.
- Added getOptions method.
- Added singleRadio option to support hide radio buttons.
- Added new website.
- Added bower.json.
- Added vue-starter example.
- Improved the label element for select.
- Improved text display only one option.
- Fixed IE build error with core-js devDependencies.
- Fixed onCheckAll/onUncheckAll trigger bug.
- Fixed disabled options cannot show after filtering.
- Fixed single filter bug.
v1.3.1 (2019-08-21)
- Fixed optgroup selected item display bug
- Fixed filter li display blank space bug
- Fixed container outer width bug
- Updated addTitle option to displayTitle option
- Fixed filter with no select options display bug
- Fixed openOnHover bug
- Updated onOptgroupClick and onClick parameters
- Updated formatCountSelected parameters
- Added locale support
v1.3.0 (2019-05-15)
- New: Rewrote code to ES6.
- New: Added filterGroup option.
- New: Added babel and refined the code.
- New: Used dist to output the build files.
- New: Added full examples.
- Update: Fixed css escape bug
v1.2.3 (2019-04-27)
- New: Added onAfterCreate event
- New: Made option labels appear clickable
- New: Allowed placeholder from html
- Update: Fixed groups don't get checked by default
- Update: Fixed getSelects bug with single option
- Update: Removed duplicate destroy method
- Update: Fixed outdated CSS syntax in linear-gradient
- Update: Removed selectAll whitespace
- Update: Fixed selectAllText/allSelected doesn't work
- Update: Fixed IE 11 crashing bug
- Update: Fixed radio style bug
v1.2.2 (2018-12-23)
- Updated the default of textTemplate to .html().
- Added wrap span to label text.
- Added destroy method.
- Added openOnHover option.
- Rebuild docs and use gh-pages.
- Update multiple-select.css.
- Make setSelects Change Event Trigger Optional
v1.2.1 (2016-01-03)
- Fix: single select with Optgroups bug.
- Fix: special character problem.
- Fix: Added onFilter event.
- Fix: added animate option to support fade and slide animates.
- Fix: element detection.
- Fix jQuery dependency.
- Fixed disable issue.
- Add selected class to 'select all' option.
- Added logic to perform accent insensitive compare when filtering values.
- Added dropWidth option.
- Added open and close methods.
v1.2.0 (2015-11-24)
- [enh] Update jquery.multiple.select.js to multiple-select.js.
- [enh] Trigger change for select after set of new value.
- [bug] Prevents updateSelectAll() from calling options.onCheckAll() on init.
- [enh] Added labelTemplate option.
- [bug] Fix Automatically set Group when all child was selected.
- [bug] Fixed filter functionality with 'no-results' label behavior.
- [bug] Fix #184: prevented the dropdown handle error.
- [enh] INPUT tags should be nameless.
- [bug] Fix auto hide when the single option is set to true.
- [bug] Fix show selectAll and hide noResults when open.
- [bug] Fix update width option to support a percentage setting.
- [bug] Trigger the checkbox on the entire line.
- [bug] Added noMatchesFound option.
- [bug] Update seperator to separator.
- [enh] Allow object of options along with initial method.
- [enh] Add a filterAcceptOnEnter option.
- [enh] Put class on ms-parent div instead of ul.
- [bug] Fixed connect select back to its label.
- [enh] Added hideOptgroupCheckboxes option to hide optgroup checkboxes.
- [enh] Added displayValues and delimiter options.
- [enh] Added textTemplate option to custom the text.
- [enh] Added selectAllDelimiter option.
- [enh] Added ellipsis option.
- [enh] Get percentage width, if used.
- [bug] Fix spelling error.
- [bug] Fixed the error when element id's contain colons.
- [bug] Fix current selected element not displayed in newer jquery versions.
- [bug] Fix plain text stored to HTML.
- [bug] Update multiple-select.png.
- [enh] Added 'close' to allowedMethods.
- [bug] Prevent dropdown from closing occasionally when clicking on checkbox.
- [bug] Fixed dropdown not closing when focus was lost.
- [enh] Support for add title (tooltip) on the select list.
2014-09-23
- correct typo
2014-05-05
- update width option to support a percentage setting, and add responsive layout.
2014-04-29
- Fixed: show selectAll and hide noResults when open.
2014-04-26
- Fixed: auto hide when the single option is set to true.
2014-04-25
- Add keepOpen option.
- Fix isOpen and filter options are both true bug.
- Fire onCheckAll event when literally select.
- Add data attributes for support.
- Add name option.
2014-04-19
- Fixed: fire onCheckAll when literally select each element till all are selected.
- Fixed: call open function after init when isOpen and filter options are set to true.
v1.0.9 (2014-04-15)
- add onFocus and onBlur events.
- Fix countSelected display error when select has disabled options.
v1.0.8 (2014-03-12)
- add 'No matches found' message when there are no results found.
v1.0.7 (2014-03-06)
- add position option.
v1.0.6 (2014-03-06)
- fixed the filters not working bugs.
v1.0.6 (2014-03-05)
- add an to allow only single select.
v1.0.5 (2013-11-27)
- update the optgroups select text.
- fixed image is not shown in Firefox 25.0.1
This awesome jQuery plugin is developed by wenzhixin. For more Advanced Usages, please check the demo page or visit the official website.