User-friendly Multi Select Plugin For Bootstrap 5/4 - BsMultiSelect
File Size: | 1.27 MB |
---|---|
Views Total: | 146635 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
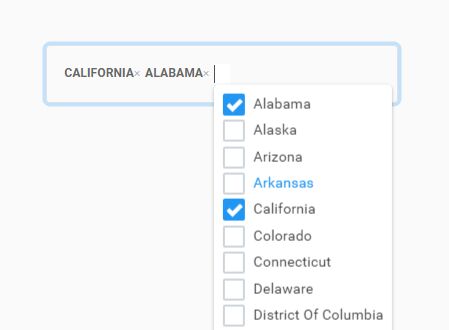
Just another multi-select plugin for Bootstrap 5 (and 4) framework that converts a multi-select list into an easy to use dropdown with checkboxes.
You users are able to select one or more options from the dropdown list by clicking the checkboxes.
To clear the selection, just click the x
icon inside the selected options just like the tags input.
More Features:
- RTL support.
- Allows to implement as an ES module (requires no jQuery).
- Form validation.
- Live filter.
- Licensed under APACHE 2.
How to use it:
1. To use the plugin, make sure you have jQuery library and Bootstrap 5 (or Bootstrap 4) framework installed.
<!-- Bootstrap Stylesheet --> <link rel="stylesheet" href="/path/to/cdn/bootstrap.min.css" /> <!-- jQuery Is Optional --> <script src="/path/to/cdn/jquery.slim.min.js"></script> <!-- Bootstrap JavaScript --> <script src="/path/to/cdn/bootstrap.bundle.min.js"></script>
2. Load the jQuery BsMultiSelect plugin's script after jQuery.
<!-- For Bootstrap 5 --> <script src="dist/js/BsMultiSelect.min.js"></script> <!-- For Bootstrap 4 --> <script src="dist/js/BsMultiSelect.bs4.min.js"></script>
3. Just call the function dashboardCodeBsMultiSelect
on the multi select list and the plugin will do the rest.
<select name="states" id="example" class="form-control" multiple="multiple" style="display: none;"> <option value="AL">Alabama</option> <option value="AK">Alaska</option> <option value="AZ">Arizona</option> <option value="AR">Arkansas</option> <option selected value="CA">California</option> ... </select>
$(function(){ $("select").bsMultiSelect(); });
4. You can also define the options in the JavaScript as follows.
<div class="container"> ... </div>
$('.container').bsMultiSelect({ options : [ {text:"Asia", value:"C0",hidden:false,disabled:false,selected:true}, {text:"Europe",value:"C1",hidden:false,disabled:false,selected:false}, {text:"Australia",value:"C2",hidden:false,disabled:false,selected:false} ], getDisabled : () => {}, getIsValid : () => {}, getIsInvalid : () => {} });
5. All default options to customize the plugin.
$("select").bsMultiSelect({ useCssPatch: true, containerClass: "dashboardcode-bsmultiselect", css: css, cssPatch: cssPatch, placeholder: '', staticContentGenerator: null, getLabelElement: null, pickContentGenerator: null, choiceContentGenerator: null, buildConfiguration: null, isRtl: null, setSelected: null, required: null, optionsAdapter: null, options: null, getDisabled: null, getSize: null, getValidity: null, labelElement: null, valueMissingMessage: '', getIsValueMissing: null });
6. Override the default styles & Bootstrap 4 classes to customize the plugin.
$("select").bsMultiSelect({ cssPatch: { choices: { listStyleType: 'none' }, picks: { listStyleType: 'none', display: 'flex', flexWrap: 'wrap', height: 'auto', marginBottom: '0' }, choice: 'px-md-2 px-1', choice_hover: 'text-primary bg-light', filterInput: { border: '0px', height: 'auto', boxShadow: 'none', padding: '0', margin: '0', outline: 'none', backgroundColor: 'transparent', backgroundImage: 'none' // otherwise BS .was-validated set its image }, filterInput_empty: 'form-control', // need for placeholder, TODO test form-control-plaintext // used in staticContentGenerator picks_disabled: { backgroundColor: '#e9ecef' }, picks_focus: { borderColor: '#80bdff', boxShadow: '0 0 0 0.2rem rgba(0, 123, 255, 0.25)' }, picks_focus_valid: { borderColor: '', boxShadow: '0 0 0 0.2rem rgba(40, 167, 69, 0.25)' }, picks_focus_invalid: { borderColor: '', boxShadow: '0 0 0 0.2rem rgba(220, 53, 69, 0.25)' }, // used in BsAppearance picks_def: { minHeight: 'calc(2.25rem + 2px)' }, picks_lg: { minHeight: 'calc(2.875rem + 2px)' }, picks_sm: { minHeight: 'calc(1.8125rem + 2px)' }, // used in pickContentGenerator pick: { paddingLeft: '0px', lineHeight: '1.5em' }, pickButton: { fontSize: '1.5em', lineHeight: '.9em', float: "none" }, pickContent_disabled: { opacity: '.65' }, // used in choiceContentGenerator choiceContent: { justifyContent: 'initial' }, // BS problem: without this on inline form menu items justified center choiceLabel: { color: 'inherit' }, // otherwise BS .was-validated set its color choiceCheckBox: { color: 'inherit' }, choiceLabel_disabled: { opacity: '.65' } } });
7. You're able to style the plugin using your own CSS without Bootstrap 4.
$("select").bsMultiSelect({ useCssPatch: false, css: { choices: 'dropdown-menu', // bs4, in bsmultiselect.scss as ul.dropdown-menu choice_hover: 'hover', // not bs4, in scss as 'ul.dropdown-menu li.hover' choice_selected: '', choice_disabled: '', picks: 'form-control', // bs4, in scss 'ul.form-control' picks_focus: 'focus', // not bs4, in scss 'ul.form-control.focus' picks_disabled: 'disabled', // not bs4, in scss 'ul.form-control.disabled' pick_disabled: '', pickFilter: '', filterInput: '', // used in BsPickContentStylingCorrector pick: 'badge', // bs4 pickContent: '', pickContent_disabled: 'disabled', // not bs4, in scss 'ul.form-control li span.disabled' pickButton: 'close', // bs4 // used in BsChoiceContentStylingCorrector // choice: 'dropdown-item', // it seems like hover should be managed manually since there should be keyboard support choiceCheckBox_disabled: 'disabled', // not bs4, in scss as 'ul.form-control li .custom-control-input.disabled ~ .custom-control-label' choiceContent: 'custom-control custom-checkbox d-flex', // bs4 d-flex required for rtl to align items choiceCheckBox: 'custom-control-input', // bs4 choiceLabel: 'custom-control-label justify-content-start', choiceLabel_disabled: '' } });
8. Implement it as an ES module.
import Popper from "https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.16.1/esm/popper.js" import {BsMultiSelect} from "./dist/js/BsMultiSelect.esm.min.js" var mySelect = document.querySelector('#select-id'); var environment = {window, Popper}; bsMultiSelect = BsMultiSelect(mySelect, environment, { // settings here });
9. Update changes on the component with the following API methods.
$('select').bsMultiSelect("UpdateIsValid"); $('select').bsMultiSelect("UpdateDisabled"); $('select').bsMultiSelect("UpdateSize"); $('select').bsMultiSelect("UpdateWasValidated"); $('select').bsMultiSelect("UpdateValidy"); $('select').bsMultiSelect("UpdateOptionsSelected", index); $('select').bsMultiSelect("UpdateOptionDisabled", index); $('select').bsMultiSelect("UpdateOptionHidden", index); // or all-in-one $('select').bsMultiSelect("UpdateAppearance"); $('select').bsMultiSelect("UpdateData");
Changelog:
v2.0.0beta28 (2021-12-19)
- Update
v2.0.0beta27 (2021-12-08)
- Update
v2.0.0beta26 (2021-12-05)
- Update NPM package.
v2.0.0beta25 (2021-12-01)
- Update NPM package.
- Bootstrap 4 version updated
v1.1.18 (2021-09-06)
- Bugfix
v1.1.17 (2021-09-02)
- Bugfix
v1.1.16 (2021-08-25)
- CSS update
v1.1.15 (2021-07-04)
- optimizations
v1.1.14 (2021-07-04)
- scrollIntoView improved
v1.1.13 (2021-06-29)
- scrollIntoView for ChoicesDynamicStylingPlugin
v1.1.13 (2021-06-28)
- scrollIntoView for ChoicesDynamicStylingPlugin
v1.1.12 (2021-06-26)
- Bugfixes.
v1.1.11 (2021-06-25)
- Bugfixes.
v1.1.8 (2021-06-24)
- HighlightPlugin, CustomChoiceStylingPlugin, CustomPickStylingPlugin
v1.1.7 (2021-06-20)
- ChoicesDynamicStylingPlugin
v1.1.6 (2021-06-17)
- Bugfix
- Improved 'no result' message.
v1.1.1 (2021-06-08)
- IE11 set placeholder problem solved
v1.1.0 (2021-06-08)
- breaking change in DOM generation
v1.0.4 (2021-06-05)
- breaking change in DOM generation: previous dropdown ul become wrapped to div
v1.0.3 (2021-06-04)
- problem with padding between picks in rtl solved
v1.0.1/2 (2021-06-03)
- UMD bundle without jquery support
- Floating labels (Bootstrap 5 version)
v1.0.0 (2021-05-27)
- Supports Bootstrap 5
v0.6.27 (2020-08-19)
- updated
v0.6.27 (2020-08-05)
- restore compatability with old code
v0.6.25 (2020-07-02)
- optimizations
v0.6.23 (2020-06-27)
- optimization
v0.6.22 (2020-06-26)
- Bugfixed
v0.6.21 (2020-06-25)
- Bugfixed
v0.6.20 (2020-06-24)
- Optimization.
v0.6.19 (2020-06-23)
- Optimization.
v0.6.18 (2020-06-22)
- Bugfix
v0.6.15 (2020-06-20)
- Optimized layout api
v0.6.14 (2020-06-19)
- FilterAspect redesign
v0.6.13 (2020-06-18)
- Layout redesign
v0.6.12 (2020-06-17)
- API changes
v0.6.11 (2020-06-16)
- Bugfix
v0.6.10 (2020-06-15)
- Bugfix
v0.6.9 (2020-06-13)
- Optimized
v0.6.8 (2020-06-13)
- Optimized API
v0.6.7 (2020-06-11)
- Bugfix
v0.6.5 (2020-06-11)
- Optimized.
v0.6.4 (2020-06-10)
- Optimized.
v0.6.3 (2020-06-09)
- Fixed ReferenceError: adjustLegacySettings is not defined
v0.6.2 (2020-06-08)
- Updated API
v0.6.0 (2020-06-02)
- changed API names
v0.5.68 (2020-06-01)
- optimizations
v0.5.67 (2020-05-31)
- optimizations
v0.5.66 (2020-05-30)
- optimizations
v0.5.65 (2020-05-29)
- Fixed IE11 flex start.
v0.5.64 (2020-05-29)
- optimizations
v0.5.63 (2020-05-21)
- optimizations
v0.5.62 (2020-05-20)
- optimizations
v0.5.61 (2020-05-19)
- Bug fixed
v0.5.60 (2020-05-18)
- Bug fixed
v0.5.59 (2020-05-17)
- Bug fixed
v0.5.58 (2020-05-05)
- FormRestoreOnBackwardPlugin
v0.5.57 (2020-05-05)
- optimizations
v0.5.56 (2020-05-04)
- added Placeholder plugin
v0.5.55 (2020-05-01)
- optimizations
v0.5.53 (2020-04-30)
- optimizations
v0.5.52 (2020-04-29)
- optimizations
v0.5.51 (2020-04-28)
- optimizations
v0.5.50 (2020-04-26)
- plugin api available for BsMultiSelect esm module
v0.5.49 (2020-04-25)
- Added preventOverflow option to popover.
v0.5.48 (2020-04-24)
- API changed
v0.5.47 (2020-04-22)
- Bugfix
v0.5.46 (2020-04-21)
- API changed.
v0.5.45 (2020-04-19)
- Improvement
v0.5.44 (2020-04-18)
- alignToFilterInputItemLocation optimized
v0.5.43 (2020-04-17)
- Fixed flex start on IE11.
v0.5.42 (2020-04-16)
- optimizations
v0.5.41 (2020-04-15)
- Changed API - UpdateOptionHidden
- Bugfixed
v0.5.40 (2020-03-18)
- Optimization.
v0.5.39 (2020-03-17)
- Better User Experience.
v0.5.38 (2020-03-16)
- Bug fixed
v0.5.37 (2020-03-15)
- Methods updated
v0.5.36 (2020-03-12)
- Added UpdateOptionInserted method
v0.5.35 (2020-03-11)
- optimizations
v0.5.34 (2020-03-10)
- optimizations
v0.5.33 (2020-03-09)
- optimizations
v0.5.32 (2020-03-08)
- Added new method UpdateOptionsDisabled
- Renamed UpdateSelected to UpdateOptionsSelected
v0.5.31 (2020-03-07)
- Bugfixed
v0.5.30 (2020-03-06)
- Fixed memory leak bug
v0.5.29 (2020-03-05)
- Press Enter in the input will open the dropdown
v0.5.28 (2020-03-04)
- Optimized for filter
v0.5.27 (2020-03-03)
- Fixed full match issue
v0.5.26 (2020-03-02)
- optimizations
v0.5.25 (2020-03-01)
- optimizations
v0.5.24 (2020-02-29)
- added UpdateSelected API
v0.5.23 (2020-02-28)
- configs optimized
v0.5.22 (2020-02-27)
- optimizations
v0.5.21 (2020-02-26)
- Styling update
v0.5.20 (2020-02-25)
- Bugfix
v0.5.19 (2020-02-24)
- optimizations
v0.5.18 (2020-02-24)
- API updated
v0.5.16 (2020-02-22)
- Optimization
v0.5.15 (2020-02-21)
- setSelected will not remove selected attribute
v0.5.14 (2020-02-20)
- new method UpdateAppearance
v0.5.13 (2020-02-19)
- BsMultiSelect jQuery prototype can create instance (was only return)
v0.5.12 (2020-02-18)
- Bugfix
v0.5.11 (2020-02-17)
- Added GetChoices() method that returns 'dropdown' menu
v0.5.10 (2020-02-12)
- ES6 module
v0.5.9 (2020-02-12)
- optimizations
v0.5.8 (2020-02-10)
- optimizations
v0.5.6/7 (2020-02-09)
- optimizations
v0.5.5 (2020-02-08)
- Fixed the styling of dropdown
v0.5.4 (2020-02-06)
- configuring css/cssPatch, assuming null/undefined value to styles property - the property will be removed from default styles dictionary
v0.5.3 (2020-02-06)
- justification in dropdown menu for inline form solved
v0.5.2 (2020-02-05)
- Bugs fixed
v0.5.0 (2020-02-04)
- updated
v0.4.34beta (2020-01-24)
- normalized options
- mem leak solved
- rtl support
v0.4.33 (2019-12-21)
- better suport of group-input
v0.4.32 (2019-12-20)
- getSize, getDisabled, getIsValid, getIsInValid (actual for js-object initiated) are available to setup from configuration
v0.4.31 (2019-12-20)
- Better padding for sm lg sizes
v0.4.30 (2019-12-19)
- configuration.setSelected as hook for pre and post selection events
v0.4.29 (2019-12-17)
- supports form-control-lg/sm
- supports input-group-lg and input-group-sm (on container)
v0.4.27 (2019-12-17)
- better look on phone devices
v0.4.26 (2019-12-16)
- fixed placeholder issues.
v0.4.20 (2019-12-14)
- placeholder on IE11 looks better
v0.4.19 (2019-12-14)
- Added placeholder support
v0.4.18 (2019-12-13)
- "no flick" dom elements instantioning in html; bootstrap prepend, append; select all and deselect all methods
v0.4.17 (2019-12-11)
- Supports fieldset
v0.4.10/11/12 (2019-12-08)
- form reset support
- removed console output
- now "GetContainer" returns container div
- fixed bugs
v0.4.10/11/12 (2019-12-07)
- uncheck selected error has been solved
- esc first close the dropdown then modal
v0.4.9 (2019-12-06)
- small optimizations & bug fix
v0.4.6 (2019-12-03)
- Bugfix
v0.4.5 (2019-11-28)
- ESC keydown now processed with preventDefault (to do not duplicate clear text custom functionality)
v0.4.4 (2019-11-27)
- click inside selected panel doesn't clear the filter input (we have bootrap x for this and ESC button)
v0.4.3 (2019-11-26)
- Fixed: "two dropdown items can be hovered for a moment" (one by mouse second from keyboard)
v0.4.2 (2019-11-13)
- configuration API changed; "selected" happens when full text entered
v0.4.1 (2019-11-01)
- added "buildConfiguration" method
v0.4.0 (2019-10-25)
- added "buildConfiguration" method
- id generation for label-input was redesigned once more time (now id is used first, if id is absent then name is used)
- checkbox without ids from now (js events are used to reference label and checbox, not "for-id" functionality).
v0.3.0 (2019-10-21)
- configuration object is now named "configuration" (was options)
- id generation - new ids (btw, id generation can be customized assigning configuration.createCheckBoxId and configuration.createInputId methods)
v0.2.24 (2019-07-28)
- adding (hardcoded) css foat:none to "cancel" button span (x) because BS team have added float:rigth to it (why?) in one of newest BS versions; this was the reason that sometimes badge's "x" button moved to new line separately.
v0.2.23 (2019-07-27)
- support bsMultiSelect on dropdown: click to remove/unselect the item is now processed in setTimout(..,0) this helps filter out close clicks in dropdown's hide event handler (investigate that clicks target was bsMultiSelect and ignore it)
v0.2.22 (2019-04-12)
- better UX, when only one left in aotosuggestion list - tab and enter select it
2018-10-11
- height initial -> auto since IE11 doesn't support initial (and node updates)
2018-07-25
- support BS 4.1.3 fixed height form-control
2018-06-25
- fix "can select disable"
2018-06-23
- v0.2.9: Bugfix
2018-06-22
- v0.2.7
2018-06-20
- Bugfix
2018-06-13
- renamed readonly to disabled
2018-06-11
- dispose for label click return last for value
2018-06-06
- fixe auto close after selection
- fix options bug
2018-06-05
- trigger change on original select
2018-06-02
- dynamic disabled support
2018-05-31
- adoptFilterInputLength in clearFilterInput
2018-05-24
- adoptFilterInputLength in clearFilterInput
2018-05-23
- v0.1.3: code adjusted by eslint; fixed bug with visible menu selector
2018-05-21
- v0.1.0
This awesome jQuery plugin is developed by DashboardCode. For more Advanced Usages, please check the demo page or visit the official website.