Validate Bootstrap Forms In A Snap With jQuery
File Size: | 8.07 MB |
---|---|
Views Total: | 234 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
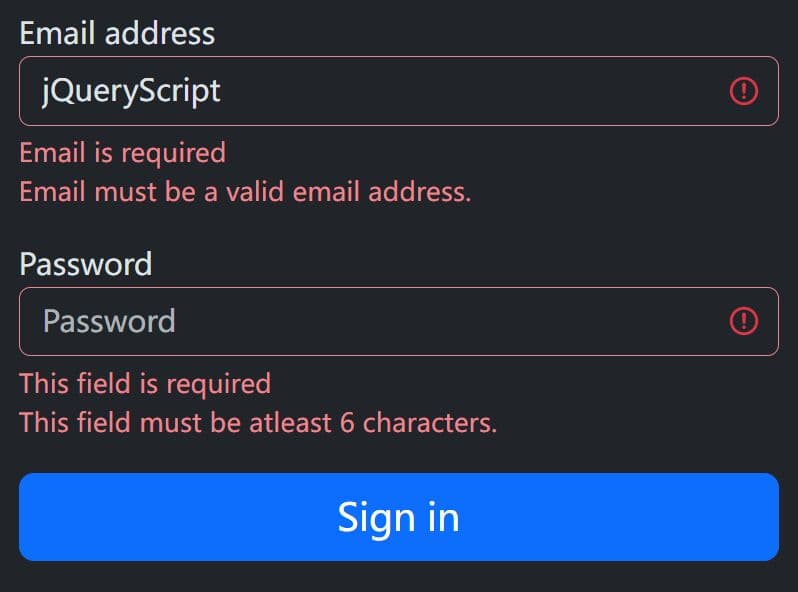
A lightweight jQuery plugin provides an easy way to implement client-side form validation in your latest Bootstrap projects.
The plugin allows setting validation rules like required, email, min/max length directly on form fields by leveraging HTML5 data
attributes. When a field doesn't meet the validation criteria, it's immediately highlighted, and a helpful error message appears, guiding users to provide the correct information.
How to use it:
1. Load the needed jQuery library and bootstrap-form-validation.js
script in your Bootstrap project.
<!-- jQuery is required --> <script src="/path/to/cdn/jquery.min.js"></script> <!-- Bootstrap 5 --> <link rel="stylesheet" href="/path/to/cdn/bootstrap.min.css" /> <script src="/path/to/cdn/bootstrap.bundle.min.js"></script> <!-- Bootstrap Form Validation Plugin --> <script src="/path/to/bootstrap-form-validation.min.js"></script>
2. Implement validation rules on form fields using the following data-validate-validation="*"
attributes.
- Trim: Trims the whitespace
- Required: Required field
- Email: Must be a valid email address
- Minimum length: Minimum length, e.g. min_length[2]
- Maximum length: Maximum length, e.g. max_length[14]
- Number: Must be a valid number
- Matches: Must match the value you typed in another field, e.g. matches[#password]
<!-- Email Address --> <input data-validate-validation="required|email" /> <!-- Password --> <input data-validate-validation="required|min_length[6]|max_length[12]" type="password" /> ...
3. Initialize the Bootstrap Form Validation plugin on your HTML form.
<!-- Validate form fields on submit --> <form data-validate-on-submit="true"> ... form fields here </form> <!-- Validate form fields on blur --> method="POST" action=""> ... form fields here </form>
4. Determine whether to scroll the page to the top of the form in cases where at least one form field is in valid.
<form data-validate-on-submit="true" data-validate-scroll-on-fail="true"> ... form fields here </form>
This awesome jQuery plugin is developed by RobertGrubb. For more Advanced Usages, please check the demo page or visit the official website.