Minimal Stopwatch & Timer Plugin For jQuery
File Size: | 54.3 KB |
---|---|
Views Total: | 35109 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
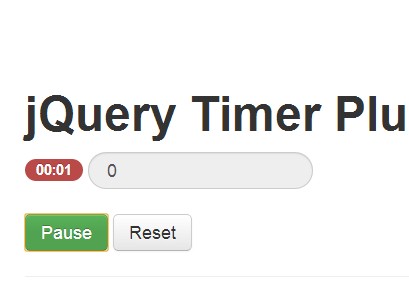
A clean and simple jQuery stopwatch/timer plugin which allows you to count up from zero or a certain time with start, pause and resume controls.
Basic Usage:
1. Create a container for the stopwatch/timer.
<div id="t" class="badge">00:00</div>
2. Create a control button which allows to start, pause and resume the timer in one button.
<button id="btn">Start</button>
3. Load the jQuery library and jQuery timer plugin at the end of your page.
<script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/cdn/timer.jquery.min.js"></script>
4. The JavaScript to enable the timer.
(function(){ $("#btn").click(function(){ switch($(this).html().toLowerCase()) { case "start": $("#t").timer({ action: 'start', seconds: 0 }); $(this).html("Pause"); $("input[name='s']").attr("disabled", "disabled"); $("#t").addClass("badge-important"); break; case "resume": $("#t").timer('resume'); $(this).html("Pause") $("#t").addClass("badge-important"); break; case "pause": //you can specify action via object $("#t").timer({action: 'pause'}); $(this).html("Resume") $("#t").removeClass("badge-important"); break; } }); })();
5. Style the timer and control button via CSS.
.badge-important { ... } ...
6. Available settings and callbacks
// Default seconds value to start timer from seconds: 0, // Allow making changes to the time by clicking on it editable: false, // Duration to run callback after duration: null, // Turn off the display of the timer hidden: false // Default callback to run after elapsed duration callback: function() { console.log('Time up!'); }, // This will repeat callback every n times duration is elapsed repeat: false, // If true, this will render the timer as a countdown (must have duration) countdown: false, // This sets the format in which the time will be printed format: null, // How often should timer display update updateFrequency: 500
7. API methods.
//pause an existing timer $("#div-id").timer('pause'); //resume a paused timer $("#div-id").timer('resume'); //remove an existing timer $("#div-id").timer('remove'); //leaves the display intact //get elapsed time in seconds $("#div-id").data('seconds');
8. Get the current state of your timer.
$('#div-id').data('state');
Changelog:
v0.9.1 (2019-12-23)
- Updated timer to support continuous run
v0.9.0 (2018-11-27)
- Adding new parameter
v0.8.0 (2018-09-27)
- Added data state 'removed
v0.7.0 (2017-04-17)
- Move to ES 5
v0.6.5 (2016-03-23)
- Incremental improvements
v0.6.3 (2016-07-05)
- Fixed redundant early return
v0.6.2 (2016-06-17)
- (fix) Object.assign issue on mobile
- Removed all Object.assign instances
v0.5.3 (2016-06-10)
- Add support for days
v0.5.2 (2016-06-08)
- (fix) countdown pause issue
v0.4.14 (2016-05-15)
- Fixed Timer problem Reset
v0.4.10 (2016-01-30)
- Fixed reset timer & added test for reset.
v0.4.9 (2016-01-16)
- Fixed issue where empty args did not translate to start
v0.4.4 (2015-12-27)
- Added AMD support for requirejs
v0.4.3 (2015-08-04)
- FIXED: Pausing, editing the time, and resuming could update the duration to reflect new time
v0.4.2 (2015-06-17)
- Improvement added: Customize timer display update frequency
v0.4.1 (2015-04-25)
- Added functionality to get the current state of the timer.
- Fixed a callback issue when seconds parameter is also passed along with duration parameter
2014-12-07
- Added functionality to edit timer
- Revamped timer.start and added testing ground
2014-11-01
- Switched to nullifying data object from element instead of $.removeData on clearing timer
2014-07-25
- Added repeat callback notification functionality
2014-07-23
- Added function to convert pretty time to seconds
2014-02-19
- Added a check to see if timer is running. Tweaked CSS for demo.
2014-02-06
- added feature to display time in a verbose fashion
- added timer value in seconds to a data attr on the element itself
This awesome jQuery plugin is developed by walmik. For more Advanced Usages, please check the demo page or visit the official website.