jQuery Date and Time Picker Plugin - Simple Datetimepicker
File Size: | 74.5 KB |
---|---|
Views Total: | 109496 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
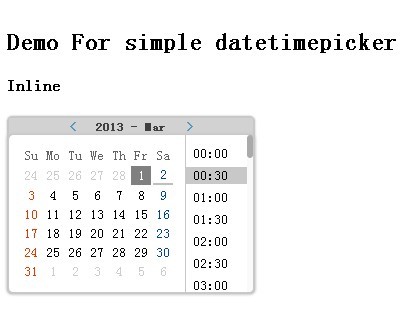
Simple Datetimepicker is a jQuery plugin that makes it easy to create Date and Time Picker in different styles for your project.
View more
Basic Usage:
1. Include jQuery library in the head section of your html page
<script src="/path/to/jquery.min.js"></script>
2. Include jquery.simple-dtpicker.js and jquery.simple-dtpicker.css after jQuery Library
<script src="jquery.simple-dtpicker.js"></script> <link type="text/css" href="jquery.simple-dtpicker.css" rel="stylesheet" />
3. Create a container element in which the plugin render the date/time picker.
<div id="date_picker"></div>
4. Call the plugin on the placeholder element to generate an inline date/time picker.
$(function(){ $('#date_picker').dtpicker(); });
5. Or attach the date/time picker to an input field.
<input type="text" name="date" value="">
$(function(){ $('*[name=date]').appendDtpicker(); });
6. Available plugin options to customize the date/time picker.
$('*[name=date]').appendDtpicker({ // current date/time "current": null, // e.g. DD.MM.YY H:mmTT "dateFormat": "default", /* Available languages: "en" "et" "br" "cn" "cz" "da" "de" "es" "fr" "gr" "hu" "id" "it" "ja" "ko" "nl" "no" "pt" "pl" "ro" "ru" "sv" "tr" "ua" */ "locale": "en", // enable/disable animation "animation": true, // minute interval "minuteInterval": 30, // 0 = Sunday "firstDayOfWeek": 0, // closes the calendar after selection "closeOnSelected": false, // enable/disable auto scroll "timelistScroll": true, // enables mouse scroll on the calendar "calendarMouseScroll": true, // shows today button "todayButton": true, // shows close button "closeButton": true, // date picker only "dateOnly": false, // time picker only "timeOnly": false, // only allows future datetimes "futureOnly": false, // min/max dates "minDate": null, "maxDate": null, // auto sets date on start "autodateOnStart": true, // min/max times "minTime": "00:00", "maxTime": "23:59", // allowed days "allowWdays": null, // shows AM/PM in the time list "amPmInTimeList": false, // external local "externalLocale": null });
7. Callback functions.
$('*[name=date]').appendDtpicker({ "onShow": function(){ // on show }, "onHide": function(){ // on hide }, "onSelect": function(){ // on select } });
8. API methods.
// shows the date picker $('*[name=date]').handleDtpicker('show'); // hides the date picker $('*[name=date]').handleDtpicker('hide'); // gets the selection $('*[name=date]').handleDtpicker('getDate'); // set the date $('*[name=date]').handleDtpicker('setDate', new Date(2019, 06, 11, 0, 0, 0)); // destroys the date picker $('*[name=date]').handleDtpicker('destroy');
Changelog:
v1.14.0 (2019-06-11)
- Locales: icelandic, finnish.
- Added Close button option.
- CSS-Compatibility with JQuery 3.x
- Replace deprecated .bind() calls with .on()
- Correction and completion of the PT local, option to specify time range available in the list.
- Fix double rendering when navigating to previousMonth
v1.13.0 (2016-11-21)
- [Add] Locales: Ukrainian, Hungarian, Danish, Estonian.
- [Add] Close button option.
- [Add] minDate option and setMinDate function.
- [Add] timeOnly option
- [Fix] isPast logic when calendar is in next month display.
- [Fix] Locales: Spanish, Korean.
- futureOnly option and current option can be mixed
v1.12.0 (2014-04-26)
- [Add] Automatically relocate a position of a picker when picker is float-mode.
- [Add] Automatically destroy a picker, when an input-field has lose.
- [Add] The getDate and setDate method is available on PickerHandler Object.
- [Change] Changed a parent element to root, when picker is float-mode
v1.11.0 (2014-04-25)
- [Add] Support for allowWdays option.
- [Add] Greek locale.
- [Fix] Issue at option.current - Setting the custom date and time as default.
- [Fix] Process for drawing the time cell.
- [Fix] Past date is selected when clicking on previous (futureOnly).
- [Fix] Bug when switching to next month.
- [Fix] If not available a console on this environment.
- [Fix] Position / outerHeight() gives wrong value.
v1.10.1 (2014-02-16)
- [Fix] Issue with month jumping
v1.10.0 (2013-12-27)
- [Add] Polish locale (Thanks to: viszman).
- [Add] Support for minDate and maxDate options (Thanks to: droidmunkey).
- [Add] Support for minTime and maxTime options (Thanks to: CedricLevasseur).
- [Fix] Use isNaN instead of Number.isNaN (Thanks to: vbatoufflet).
v1.9.0 (2013-11-06)
- [Add] Support for Bower (Thanks to: kmulvey).
- [Change] Improved localization mechanism (Thanks to: LucasTheCure).
- [Change] Refactor - Simplified some codes (Thanks to: LucasTheCure).
- [Fix] Close a picker when date is selected on dateOnly mode (Thanks to: Kwozzie).
v1.8.0 (2013-10-18)
- [Add] Portuguese locale (Thanks to: adc-tcarvalho).
- [Add] Dutch locale (Thanks to: Mondane).
- [Add] Czech locale (Thanks to: Jan Zatloukal).
- [Add] French locale (Thanks to: Jan matll42).
- [Add] Italian locale (Thanks to: Mauro Zuccato).
- [Fix] Fixing positioning when element is inside of nested relative parent (Thanks to: AddoSolutions).
v1.7.0 (2013-09-17)
- [Add] Korean locale (Thanks to: perhapsspy).
- [Add] "futureOnly" option and bugfix (Thanks to: camiel).
- [Add] "dateOnly" option (Thanks to: logitick).
- [Fix] Improved date parse (when using dateFormat option).
v1.6.0 (2013-09-04)
- [Add] "closeOnSelected" option for close the picker when selected date & time (Idea by: MacDknife).
- [Add] "timelistScroll" option for disable automatic scroll of timelist (Idea by: deviso).
- [Add] Change the months by scroll of mouse-wheel on the calendar (Idea by: deviso).
- [Add] "calendarMouseScroll" option for scroll calendar with mouse wheel (default: true).
- [Add] Today button for calendar (Idea by: Tanver186).
- [Add] "todayButton" option (default: true).
- [Change] Improve design (header, container).
- [Fix] Malfunction of "animation" option (Can't disable animation).
v1.1.3 (2013-05-22)
- Added Russian locale.
v1.1.2 (2013-05-09)
- Fixed IE8 compatibility.
v1.1.1 (2013-03-12)
- Fixed width of timelist for bug in ie9 (Thanks to: jjsgro).
This awesome jQuery plugin is developed by mugifly. For more Advanced Usages, please check the demo page or visit the official website.