Dynamic Pie Chart-style Progress Bar with jQuery and SVG - progresspieSVG
File Size: | 2.32 MB |
---|---|
Views Total: | 15996 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
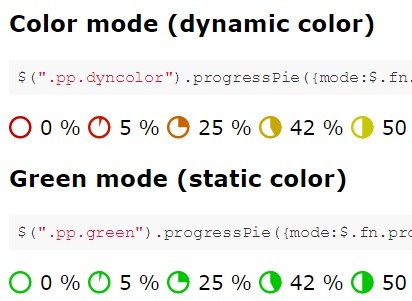
progresspieSVG is a simple yet robust jQuery plugin which renders pie or ring chart style progress bars or countdown timers using SVG
element.
It is designed to be an alternative to a progress bar, since it simply depicts a single value.
This plug-in may be used to draw a piechart with only one filled pie (rest empty).
It is designed to be an alternative to a progress bar, since it simply depicts a single value (in percent).
The plug-in assumes that values in percent are part of the document (either visible or as attribute value) and a small pie representing each value is to be dynamically inserted.
This may be either a static display or the pie may be updated upon data changes.
Features:
- Tons of customization options.
- Rotation animation.
- Dynamic auto updating.
Typical application:
This mode assumes by default, that a value (integer number between 0 and 100 inclusive, floating point numbers are supported, but truncated) is the only text content of an HTML element, e.g. a span element, and the pie is to be prepended (or appended) to the same element. The pie will usually be auto-sized to fit into the text line. A separator String to be placed between the pie and the number may be configured. Defaults are to prepend the pie and use a non-breaking space as separator. E.g. say you have HTML code like <p>You have achieved 25 points out of 50 (<span class="pie">50</span>%)</p>
, then you may insert a pie filled by 50% with $(.pie).progressPie();
, resulting in a line like: <p>You have achieved 25 points out of 50 (<span class="pie"><svg>the pie chart</svg> 50</span>%)</p>
.
Basic usage:
Select the elements holding the percent number and to insert the pie into by a jQuery selector. On the jQuery result set call "progressPie(options)", where options is an optional object with configuration options. The plugin is applied to any element in the result set, i.e. if the selector did not found any matching element, nothing will happen, while if the selector found several matching elements, the plugin will try to insert a corresponding pie into each of the found elements individually.
1. Load the latest version of jQuery library and the jQuery progresspieSVG plugin in your project.
<script src="//code.jquery.com/jquery-2.1.4.min.js"></script> <script src="js/min/jquery-progresspiesvg-min.js"></script>
2. The HTML.
<span class="demo">100</span> %
3. Render a default pie chart style progress bar.
$(".demo").progressPie();
Configuration options:
1. Options and defaults
// $.fn.progressPie.Mode.GREY // $.fn.progressPie.Mode.RED // $.fn.progressPie.Mode.GREEN // $.fn.progressPie.Mode.COLOR mode: $.fn.progressPie.Mode.GREY, // The stroke with of the outer circle strokeWidth: 2, // If undefined, the plug-in will try to draw the pie in the actual height of the parent element. // Beware: If the element is empty, the browser may have calculated a height of 0! In this case, a default size will be used. Defining this option disables auto-sizing: the provided number will be used as height and width of the svg. // It has to be a number (in pixels), not a string with a unit! // This is typically used on empty elements in combination with valueData, valueAttr or valueSelector. size: null, // Name of a value attribute: If defined, the function will look for an attribute of this name inside the opening tag of the found element, and if found, it will parse this attribute's value instead of the element's content as the percent value. // (If defined but not of type "string", the function will throw an exception.) For accessing data-* attributes, the next option valueData is usually preferred, use valueAttr only if you want to read other attributes (not beginning with data-) or if you really want to react to updates to the attribute in the DOM tree. valueAttr: null, // Mutually exclusive with valueAttr and valueSelector! Name of a jQuery data object. valueData: null, // Mutually exclusive with valueAttr and valueData! // If defined, the function will apply a jQuery search within the selected element to find a sub-element whose text content is to be used as a value. // Usually, the whole text content of the node previously selected (to which the progresspie-plugin is applied) is interpreted as the value. // If you want to have more content, maybe for CSS styling reasons, and the actual value is in a sub-element, but the pie should not be inserted into that sub-element but into the previously selected main element, then this option is for you. valueSelector: null, // If undefined, the color of the pie depends on the mode option, see above. // A valid string value of this option would be a color name like navy or color code like #888, #FF00BC, rgb(10,20,255). // If the value is a function, this function has to read one parameter of type number (0..100) and return a color code (string). // If the option is neiter undefined nor a string nor a function, the plugin will throw an exception. color: null, // Only evaluated if color is undefined. // Name of a color attribute: If defined, the function will look for an attribute of this name inside the opening tag of the found element, and if found, will try to use the attribute's content (string) to set the pie color. // The attribute must contain a color name or code (see color). colorAttr: null, // Only evaluated if no color has already been set with color or colorAttr. // Name of an attribute containing JavaScript code (as string literal) for calculating a color. colorFunctionAttr: null, // This object may contain a subset of the option properties described above {mode, color, valueAttr, valueAdapter, colorAttr, colorFunctionAttr, size, ringWidth}. // If inner is not undefined, then two piecharts will be drawn: An outer, larger chart with circle around it, described with all the other options, and a second, smaller, inner pie on top of the outer. // The inner circle's value might be taken from a second attribute (denoted by inner.valueAttr) or might be calculated from the same value string as the outer value, just by a different inner.valueAdapter mapping. // At least one of these two options should be defined. // Also, the inner pie should have a different color than the outer one, defined by inner.mode or inner.color. // If inner.size is specified, the outer size option should also be set manually and should be larger than innser.size. // If inner.size is left undefined, the inner pie is automatically slightly smaller than the outer one (approx. two thirds of the outer). inner: null, // If this option is ‘truthy’ (i.e. not undefined, not false, not 0 etc.), the (outer) pie or ring fragment will be animated by rotating around its center. rotation: null, // If true, the pie will be inserted at the beginning of the element's content, followed by the separator string. // If false, the separator string followed by the pie will be appended to the element's content. prepend: true, // Will separate the inserted pie from the rest of the content separator: " ", // Defines the CSS-property vertical-align of the inserted SVG element and thus the vertical alignment. verticalAlign: "bottom", // If false, the function will do nothing if the target element already contains an svg element. // Set to true if repeated calls are meant to update the graphic. update: false, // Executed when interpreting the value valueAdapter: function(value) { if (typeof value === "string") { return parseInt(value); } else if (typeof value === "number") { return value; } else { return 0; } }, // If undefined, a portion of the pie will be filled, cut out just to the center of the circle (like a partial sweep of a radar). // If ringWidth is a number, only the outer rim of this piece of the pie is drawn, leaving an empty circle in the middle with diameter ringWidth: null, // Only applicable if ringWidth is defined, ignored in pie mode. // If a ring is drawn, both ends of the ring are normally cut rectangularly. // Enabling this option draws a semicircle cap on each end. This might look prettier especially for very large ringEndsRounded: false
2. Possible values for the mode
option.
/** Default Mode: Pie is drawn in a shade of grey. The HTML color code is "#888" and may be changed by * overwriting <code>jQuery.fn.progressPie.Mode.GREY.color</code> (of type string). * @type {Object} */ GREY:{color:"#888"}, /** In mode RED the pie is drawn in red color regardless of the percentual value. * <code>jQuery.fn.progressPie.Mode.RED.value</code> is a variable of type "number" with the default value * of 200 and means the red color will be <code>rgb(200, 0, 0)</code>. * The variable RED.value is not only used in mode RED, but also in mode COLOR for calculating the * color of any value between 0 and 50. * @type {Object} */ RED:{value:200}, /** In mode GREEN the pie is drawn in green color regardless of the percentual value. * <code>jQuery.fn.progressPie.Mode.GREEN.value</code> is a variable of type "number" with the default value * of 200 and means the green color will be <code>rgb(0, 200, 0)</code>. * The variable GREEN.value is not only used in mode GREE, but also in mode COLOR for calculating the * color of any value between 50 and 100. * @type {Object} */ GREEN:{value:200}, /** In mode COLOR the color of the pie is depending on the percentual value. * The color is calculated via {@link "$.fn.progressPie".colorByPercent}. * It's the same green color as in mode GREEN for a value of 100 percent, the same red color * as in mode RED for a value of 0%. * The colors may be altered by overwriting progressPie.Mode.RED.value or progressPie.Mode.GREEN.value. */ COLOR:{}
Changelog:
2023-03-10
- v2.6.3: FIX CSS keyframes injection f. rotation animation
2018-10-25
- v2.6.2: FIX: CheckComplete-Plug-In in CSS Mode
2018-10-04
- v2.6.1
2018-10-02
- v2.6.0
2018-04-16
- v2.5.0
2017-07-10
- v2.3.0
2017-05-17
- Added replace argument to setupProgressPie()
2016-09-19
- v2.0: Fix: width/height of SVG now int
2016-04-24
- v1.5.0
2015-09-14
- Added strokeColor option
This awesome jQuery plugin is developed by isg-software. For more Advanced Usages, please check the demo page or visit the official website.