Drag'n'drop Flow Chart Plugin With jQuery And jQuery UI - flowchart.js
File Size: | 24.6 KB |
---|---|
Views Total: | 74371 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
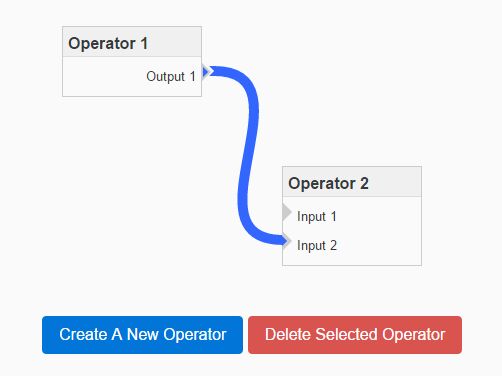
flowchart.js is a jQuery & jQuery UI based flowchart plugin that enables you to create drag'n'drop flowchart boxes and connect between them with a custom line.
Basic usage:
1. Put the jQuery flowchart.js plugin's stylesheet in the document's head section.
<link href="jquery.flowchart.css" rel="stylesheet">
2. Create an empty DIV container for the flowchart.
<div class="demo" id="example"></div>
3. The plugin requires jQuery and jQuery UI libraries loaded in the document.
<script src="jquery.min.js"></script> <script src="jquery-ui.min.js"></script> <script src="jquery.flowchart.js"></script>
4. Prepare your data for the flowchart. In this case we're going to draw 2 flowchart boxes on the webpage.
var data = { operators: { operator1: { top: 20, left: 20, properties: { title: 'Operator 1', inputs: {}, outputs: { output_1: { label: 'Output 1', } } } }, operator2: { top: 80, left: 300, properties: { title: 'Operator 2', inputs: { input_1: { label: 'Input 1', }, input_2: { label: 'Input 2', }, }, outputs: {} } }, } };
5. Apply the plugin to the target container.
$('#example').flowchart({ data: data });
6. Default options for the plugin.
$('#example').flowchart({ // allows to add links by clicking on lines canUserEditLinks: true, // enables drag and drop canUserMoveOperators: true, // custom data data: {}, // distance between the output line and the link distanceFromArrow: 3, // default operator class defaultOperatorClass: 'flowchart-default-operator', // default color defaultLinkColor: '#3366ff', // default link color defaultSelectedLinkColor: 'black', // width of the links linkWidth: 10, // grid of the operators when moved grid: 20, // allows multiple links on the same input line multipleLinksOnOutput: false, // allows multiple links on the same output line multipleLinksOnInput: false, // Allows to vertical decal the links (in case you override the CSS and links are not aligned with their connectors anymore). linkVerticalDecal: 0, // callbacks onOperatorSelect: function (operatorId) { return true; }, onOperatorUnselect: function () { return true; }, onOperatorMouseOver: function (operatorId) { return true; }, onOperatorMouseOut: function (operatorId) { return true; }, onLinkSelect: function (linkId) { return true; }, onLinkUnselect: function () { return true; }, onOperatorCreate: function (operatorId, operatorData, fullElement) { return true; }, onLinkCreate: function (linkId, linkData) { return true; }, onOperatorDelete: function (operatorId) { return true; }, onLinkDelete: function (linkId, forced) { return true; }, onOperatorMoved: function (operatorId, position) { }, onAfterChange: function (changeType) { } });
7. Public methods.
// Operators: createOperator(operatorId, operatorData) addOperator(operatorData) deleteOperator(operatorId) getSelectedOperatorId() selectOperator(operatorId) unselectOperator() addClassOperator(operatorId, className) removeClassOperator(operatorId, className) removeClassOperators(className) setOperatorTitle(operatorId, title) getOperatorTitle(operatorId) doesOperatorExists(operatorId) setOperatorData(operatorId, operatorData) getOperatorData(operatorId) getConnectorPosition(operatorId, connectorId) getOperatorCompleteData(operatorData) getOperatorElement(operatorData) getOperatorFullProperties(operatorData) // links createLink(linkId, linkData) addLink(linkData) deleteLink(linkId) getSelectedLinkId(): selectLink(linkId): unselectLink() setLinkMainColor(linkId, color) getLinkMainColor(linkId): colorizeLink(linkId, color) uncolorizeLink(linkId) redrawLinksLayer() // misc getData() setData(data) setPositionRatio(positionRatio) getPositionRatio() deleteSelected()
Changelogs:
2020-05-08
- Added method to return client rect
2019-12-20
- Small formatting fixes
- Changed where the vertical class is added
- Removed useless variables
2017-02-06
- added events
2016-12-18
- added the method doesOperatorExists
2016-12-12
- small style changes and minimization
2016-10-11
- js update
2016-08-22
- removed useless duplicate variable
2016-08-03
- Added setOperatorTitle method.
2016-07-21
- bugfix
2016-05-15
- added a move cursor to the title of flowchart operator
- added multiple connectors
2016-04-18
- added link_change_main_color onAfterChange trigger
2016-04-17
- added the linkVerticalDecal option
2016-04-13
- JS update.
2016-04-03
- fixed bug when using multiple flowcharts
This awesome jQuery plugin is developed by sdrdis. For more Advanced Usages, please check the demo page or visit the official website.