Draw Customizable Polylines Using jQuery And Canvas - polyline.js
File Size: | 6.79 KB |
---|---|
Views Total: | 1925 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
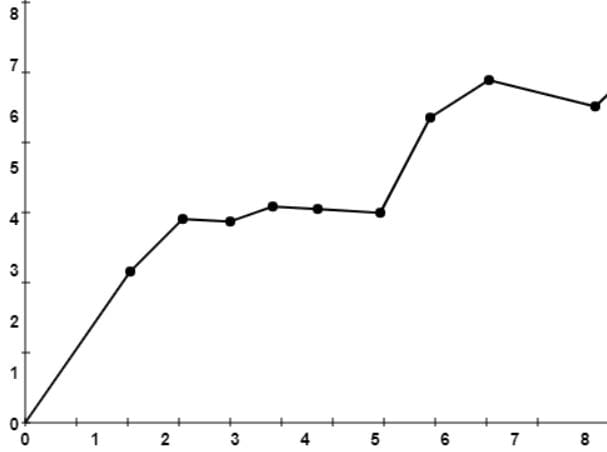
polyline.js is a jQuery plugin/jQuery UI widget that lets you draw polylines with dots on an HTML canvas to represent routes, paths, data series, etc.
How to use it:
1. Include the required jQuery and jQuery UI libraries from CDN.
<script src="/path/to/jquery.min.js"></script> <script src="/path/to/jquery-ui.min.js"></script>
2. Download and include the jquery.polyline.min.js
script after jQuery.
<script src="jquery.polyline.min.js"></script>
3. Create an HTML canvas element on which you want to draw the polylines and dots.
<canvas id="polyline"> Your browser does not support HTML5 Canvas. </canvas>
4. Initialize the plugin on the canvas element and done. Double-click the canvas to add a new dot. Double-click on a dot to remove it.
$('#polyline').polyline();
5. Customize the appearance of the polylines and dots.
$('#polyline').polyline({ max_x: 10, max_y: 10, min_dot_diff: 0.1, padding_top: 15, padding_right: 15, padding_bottom: 25, padding_left: 25, stroke_count: 10, stroke_size: 7, stroke_width: 1, stroke_shift: 4, stroke_text_font_style: "bold", stroke_text_font_name: "sans-serif", stroke_text_font_size: 14, stroke_text_hshift: 5, stroke_text_vshift: 18, stroke_text_precision: 2, line_width: 2, axis_width: 1, dot_radius: 4, dot_pick_radius_addition: 3, dots: [] // an array of predefined dots });
6. Execute a callback function each time the polyline changes.
$('#polyline').polyline({ 'change': function(event, isnew, affected) { var text = ((isnew === true) ? "Added" : (isnew === false) ? "Changed" : "Deleted") + " dot #"+affected.index+" at ( "+affected.dot[0]+" ; "+affected.dot[1]+" )"; }
This awesome jQuery plugin is developed by SmoothMouse. For more Advanced Usages, please check the demo page or visit the official website.