Adaptive Hamburger Menu With jQuery And CSS3
File Size: | 3.51 KB |
---|---|
Views Total: | 3451 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
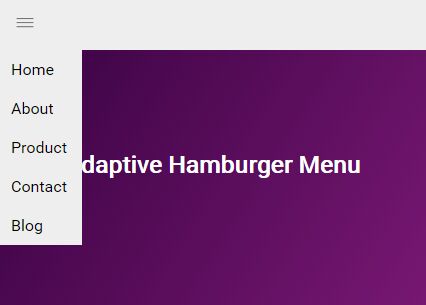
A responsive, adaptive hamburger dropdown navigation menu built using jQuery, HTML list, CSS and CSS media queries.
How to use it:
1. Create the html for the hamburger menu.
<nav> <div class="wrapper"> <div class="menuToggle"><img src="img/menu.png" alt="menu"></div> <ul class="menu clearfix"> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li><a href="#">Product</a></li> <li><a href="#">Contact</a></li> <li><a href="#">Blog</a></li> </ul> </div> </nav>
2. The primary CSS styles for the hamburger menu.
ul, li { margin: 0; padding: 0; list-style-type: none; } a { color: inherit; text-decoration: none; } .clearfix:after { content: ""; display: block; clear: both; } .wrapper { max-width: 1024px; padding: 0; margin: 0; } nav { background: #eee; } nav .wrapper { position: relative; } .menu li { float: left; } .menu li a { display: inline-block; padding: 10px 15px; } .menuToggle { padding: 10px 15px; display: none; cursor: pointer; }
3. Hide the normal menu on small screen using CSS media queries.
@media screen and (max-width: 600px) { .menu { display: none; position: absolute; background: #eee; } .menu li { float: none; } .menuToggle { display: inline-block; } }
4. Include the needed jQuery JavaScript library at the bottom of the webpage.
<script src="http://code.jquery.com/jquery-3.2.1.min.js" integrity="sha256-hwg4gsxgFZhOsEEamdOYGBf13FyQuiTwlAQgxVSNgt4=" crossorigin="anonymous"></script>
5. The core JavaScript to enable the hamburger menu toggle.
$(function(){ $('.menuToggle').on('click', function(){ $('.menu').slideToggle(300, function(){ if($(this).css('display') === 'none'){ $(this).removeAttr('style'); } }); }); });
This awesome jQuery plugin is developed by Alex5791. For more Advanced Usages, please check the demo page or visit the official website.