Responsive Hamburger Menu With jQuery And TailwindCSS
File Size: | 9.5 KB |
---|---|
Views Total: | 2368 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
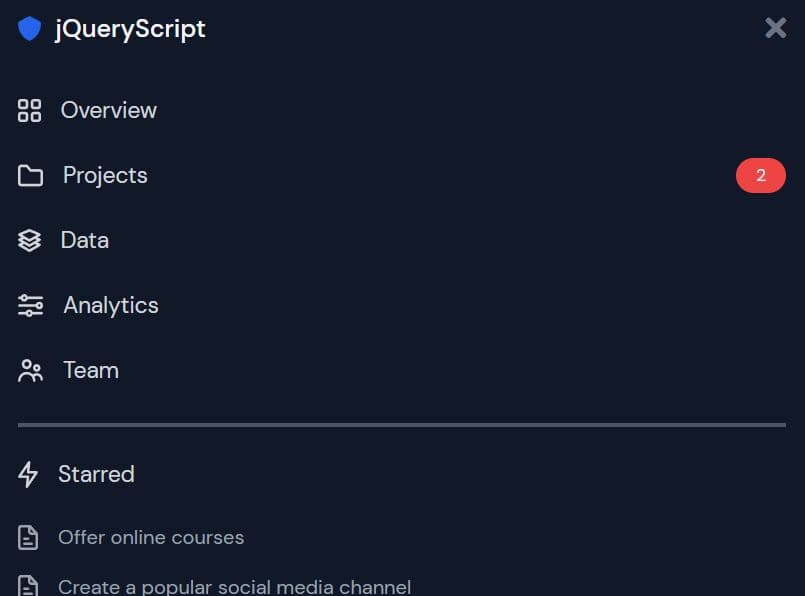
A jQuery-powered responsive menu that transforms a standard navbar into a fullscreen hamburger toggle menu on small screens.
It is built with jQuery, Tailwind.css, and Font Awesome. Easy to integrate it into your existing project in just a minute.
How to use it:
1. Load the necessary jQuery, Tailwind.css, and Font Awesome in the document.
<link rel="stylesheet" href="/path/to/font-awesome/all.min.css" /> <script src="/path/to/cdn/jquery.slim.min.js"></script> <script src="https://cdn.tailwindcss.com"></script>
2. Create the HTML for the responsive menu.
<header class="shadow-sm"> <div class="container mx-auto p-6"> <div class="flex justify-between items-center"> <a href="" class="text-gray-800 text-xl hover:text-indigo-500 font-medium inline-flex space-x-3 items-center" > <span><img src="./assets/Logo.svg" alt="" /></span> <span>jQueryScript</span> </a> <!-- Navbar --> <div id="nav" class="hidden md:inline-flex space-x-8 items-center text-gray-800" > <a href="#"><p class="hover:text-indigo-600">Overview</p></a> <a href="#"><p class="hover:text-indigo-600">Project</p></a> <a href="#"><p class="hover:text-indigo-600">Data</p></a> <a href="#"><p class="hover:text-indigo-600">Analytics</p></a> <a href="#"><p class="hover:text-indigo-600">Team</p></a> </div> <!-- Hamburger Button --> <div id="menu" class="block md:hidden"> <button class="focus:outline-none focus:outline-none" id="open"> <i class="fas fa-bars text-gray-800 text-xl items-center"></i> </button> </div> </div> <!-- Mobile Version --> <div id="mobile" class="hidden"> <div class="bg-gray-900 absolute top-0 right-0 w-full h-screen overflow-scroll p-6" > <div class="flex w-full justify-between items-center"> <a href="" class="text-gray-100 text-xl hover:text-indigo-500 font-medium space-x-3 inline-flex items-center" > <span><img src="./assets/Logo.svg" alt="" /></span> <span>Vencortex</span> </a> <div> <button class="float-right focus:outline-none focus:outline-none" id="close" > <i class="fas fa-times text-gray-500 text-2xl items-center" ></i> </button> </div> </div> <div id="mobileNav" class="pt-6 pb-6"> <div> <a href="" class="text-gray-300 text-lg py-3 hover:text-indigo-500 inline-flex items-center" > <span class="pr-4" ><img src="./assets/Overview.svg" alt="" /></span> <span>Overview</span> </a> </div> <div> <a href="" class="text-gray-300 text-lg py-3 hover:text-indigo-500 flex justify-between items-center" > <div class="inline-flex space-x-4 items-center"> <span ><img src="./assets/Project.svg" alt="" /></span> <span class="float-left">Projects</p> </div> <div> <p class="float-right text-right bg-red-500 text-gray-100 px-4 py-1 rounded-full text-sm"> 2 </p> </div> </a> </div> <div> <a href="" class="text-gray-300 text-lg py-3 hover:text-indigo-500 inline-flex items-center" > <span class="pr-4" ><img src="./assets/Data.svg" alt="" /></span> <span>Data</span> </a> </div> <div> <a href="" class="text-gray-300 text-lg py-3 hover:text-indigo-500 inline-flex items-center" > <span class="pr-4" ><img src="./assets/Analytics.svg" alt="" /></span> <span>Analytics</span> </a> </div> <div> <a href="" class="text-gray-300 text-lg py-3 hover:text-indigo-500 inline-flex items-center" > <span class="pr-4" ><img src="./assets/Team.svg" alt="" /></span> <span>Team</span> </a> </div> <div class="border-2 border-gray-600 mt-4"></div> </div> <div> <div> <div class="text-gray-300 text-lg pb-4 hover:text-indigo-500 inline-flex items-center" > <span class="pr-4" ><img src="./assets/Starred.svg" alt="" /></span> <span>Starred</span> </div> </div> <div class="text-gray-500"> <div> <a href="" class="text-gray-400 text-base py-2 hover:text-indigo-500 inline-flex items-center" > <span class="pr-4" ><img src="./assets/Doc.svg" alt="" /></span> <span>Offer online courses</span> </a> </div> <div> <a href="" class="text-gray-400 text-base py-2 hover:text-indigo-500 inline-flex items-center" > <span class="pr-4" ><img src="./assets/Doc.svg" alt="" /></span> <span>Create a popular social media channel</span> </a> </div> <div> <a href="" class="text-gray-400 text-base py-2 hover:text-indigo-500 inline-flex items-center" > <span class="pr-4" ><img src="./assets/Doc.svg" alt="" /></span> <span>Flagship course for freelancer</span> </a> </div> </div> </div> </div> </div> </div> </header>
3. Enable the hamburger button to toggle the mobile menu.
$(document).ready(function () { $('#close').click(function () { $('#mobile').fadeOut('fast', function () {}); }); $('#open').click(function () { $('#mobile').fadeIn('fast', function () {}); }); });
This awesome jQuery plugin is developed by abhisek47. For more Advanced Usages, please check the demo page or visit the official website.