Fullscreen Menu With Automatic Background Switching
File Size: | 5.99 KB |
---|---|
Views Total: | 964 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
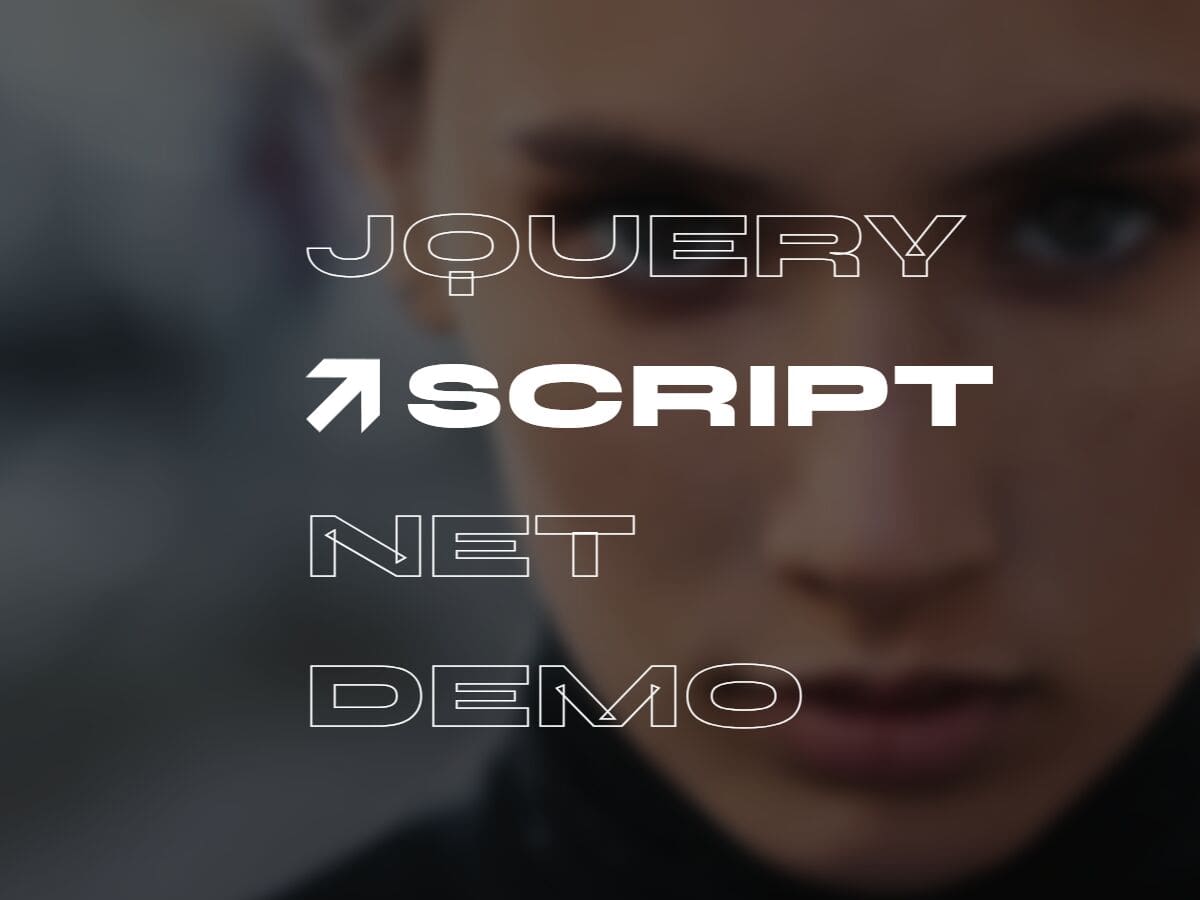
A modern fullscreen navigation with automatic background switching, written in jQuery and CSS/CSS3.
As the user hovers their cursor over each menu item, the background image will smoothly transition to a new image, providing a dynamic and engaging experience for the user.
How to use it:
1. Add a menu list and background images to the page.
<!-- Wrapper --> <div class="wrapp"> <!-- BG Images --> <div class="menu-img"> <img id="1-bg__img" src="1.jpg" alt="1"> <img id="2-bg__img" src="2.jpg" alt="2"> <img id="3-bg__img" src="3.jpg" alt="3"> ... </div> <!-- Menu Items --> <ul class="menu"> <li class="menu__item" data-id="1"> <a href="#" class="menu__link">jQuery</a> </li> <li class="menu__item" data-id="2"> <a href="#" class="menu__link">Script</a> </li> <li class="menu__item" data-id="3"> <a href="#" class="menu__link">Net</a> </li> ... </ul> </div>
2. The necessary CSS styles for the fullscreen navigation. Feel free to override the default CSS variables to create your own styles.
:root { --pr-color: #fff; --second-color: #0a0a0a; --cubicbz: cubic-bezier(.9, 0, .1, 1); } * { box-sizing: border-box; font-weight: 800; margin: 0; padding: 0; } body { background: var(--second-color); } .wrapp { position: relative; display: flex; align-items: center; justify-content: center; width: 100%; height: 100vh; overflow: hidden; z-index: 1; } .menu-img { position: absolute; width: 100%; height: 100%; top: 0; left: 0; opacity: .4; filter: blur(6px); overflow: hidden; transform: scale(1.1); } .menu-img img { position: absolute; width: 100%; height: 100%; object-fit: cover; top: 0; left: 0; transition: .8s var(--cubicbz); transform: scale(1.2); clip-path: polygon(0 0, 0 0, 0 100%, 0 100%); } .menu-img img.active { transform: scale(1); clip-path: polygon(0 0, 100% 0, 100% 100%, 0 100%); } .menu__item { position: relative; list-style: none; } .menu__item+.menu__item { margin-top: 60px; } .menu__item::before { position: absolute; content: ''; width: 60px; height: 60px; top: 50%; left: 0; transform: translate(-80px, -50%) rotate(225deg); opacity: 0; background: url(../img/arrow.svg); background-size: cover; background-repeat: no-repeat; background-position: center center; will-change: transform; transition: .8s var(--cubicbz); } .menu__link { position: relative; display: block; text-transform: uppercase; font-size: 75px; line-height: .8; text-decoration: none; color: transparent; -webkit-text-stroke: 1px var(--pr-color); z-index: 2; transition: .8s var(--cubicbz); } .menu__item:hover .menu__link { transform: translateX(80px); color: var(--pr-color); -webkit-text-stroke: 1px transparent; } .menu__item:hover::before { opacity: 1; transform: translate(0%, -50%) rotate(180deg); }
3. Insert the latest jQuery library (slim build) into the document.
<script src="/path/to/cdn/jquery.slim.min.js"></script>
4. Switch between background images when hovering over menu items.
$('.menu__item').on("mouseenter", function () { let id = $(this).data('id'); $('#' + id + '-bg__img').addClass('active'); }); $('.menu__item').on("mouseleave", function () { $('.menu-img img').removeClass('active'); });
This awesome jQuery plugin is developed by DmitriNaumov. For more Advanced Usages, please check the demo page or visit the official website.