Smooth Accordion Slider with jQuery and CSS/CSS3
File Size: | Unknown |
---|---|
Views Total: | 5145 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
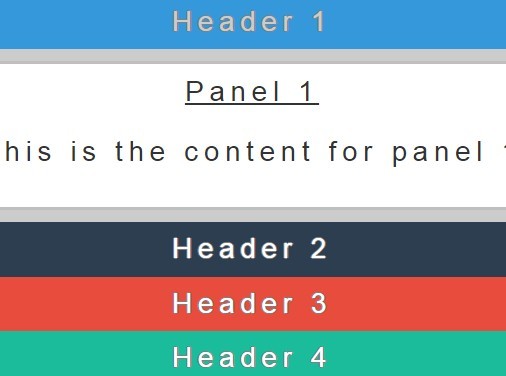
A HTML/CSS/jQuery accordion widget that uses CSS3 transitions to smoothly expand one content panel at a time.
How to use it:
1. The essential accordion structure.
<div id="accordion"> <ul class="panels"> <li data-panel-id="panel1">Header 1</li> <div class="panel panel1"> <a href="#">Panel 1</a> <p>This is the content for panel 1</p> </div> <li data-panel-id="panel2">Header 2</li> <div class="panel panel2"> <a href="#">Panel 2</a> <p>This is the content for panel 2</p> </div> <li data-panel-id="panel3">Header 3</li> <div class="panel panel3"> <a href="#">Panel 3</a> <p>This is the content for panel 3</p> </div> <li data-panel-id="panel4">Header 4</li> <div class="panel panel4"> <a href="#">Panel 4</a> <p>This is the content for panel 4</p> </div> </ul> </div>
2. The required CSS/CSS3 rules to style the accordion widget.
#accordion ul { text-align: center; margin: 0; } #accordion ul li { list-style-type: none; cursor: pointer; padding: 0.4em; font-size: 1.4em; color: white; letter-spacing: 0.2em; transition: 0.3s ease all; text-shadow: -1px 0 grey, 0 1px grey, 1px 0 grey, 0 -1px grey; } .panel { display: none; padding: 25px; border: 3px #bfbfbf solid; margin: 10px; font-family: "roboto", sans-serif; padding: 0.4em; font-size: 1.4em; color: white; letter-spacing: 0.2em; background-color: white; color: #333; }
3. Place the necessary jQuery library at the bottom of the webpage.
<script src="//code.jquery.com/jquery-2.1.3.min.js"></script>
4. The core JavaScript to enable the accordion.
$(function() { var $navLink = $('#accordion').find('li'); $navLink.on('click', function() { var panelToShow = $(this).data('panel-id'); var $activeLink = $('#accordion').find('.active'); // show new panel // .stop is used to prevent the animation from repeating // if you keep clicking the same link $('.' + panelToShow).stop().slideDown(); $('.' + panelToShow).addClass('active'); // hide the previous panel $activeLink.stop().slideUp() .removeClass('active'); }); });
This awesome jQuery plugin is developed by MarkShakespeare. For more Advanced Usages, please check the demo page or visit the official website.