Basic jQuery Photo Gallery with Image Lightbox
File Size: | 1.69 KB |
---|---|
Views Total: | 2718 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
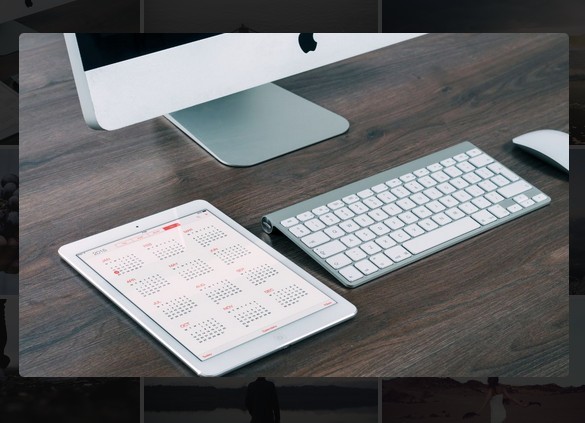
Uses plain Html and CSS to create a simple neat photo gallery from an unordered Html list. Click on a thumbnail to display the full sized image in the jQuery based lightbox popup.
How to use it:
1. Create a simple photo gallery like this:
<div id="photos"> <ul id="photo-gallery"> <li> <a href="https://unsplash.it/1280/800?image=668"> <img src="https://unsplash.it/1280/800?image=668"> </a> </li> <li> <a href="https://unsplash.it/1280/800?image=663"> <img src="https://unsplash.it/1280/800?image=663"> </a> </li> <li> <a href="https://unsplash.it/1280/800?image=675"> <img src="https://unsplash.it/1280/800?image=675"> </a> </li> <li> <a href="https://unsplash.it/1280/800?image=674"> <img src="https://unsplash.it/1280/800?image=674"> </a> </li> <li> <a href="https://unsplash.it/1280/800?image=667"> <img src="https://unsplash.it/1280/800?image=667"> </a> </li> <li> <a href="https://unsplash.it/1280/800?image=664"> <img src="https://unsplash.it/1280/800?image=664"> </a> </li> <li> <a href="https://unsplash.it/1280/800?image=662"> <img src="https://unsplash.it/1280/800?image=662"> </a> </li> <li> <a href="https://unsplash.it/1280/800?image=661"> <img src="https://unsplash.it/1280/800?image=661"> </a> </li> <li> <a href="https://unsplash.it/1280/800?image=656"> <img src="https://unsplash.it/1280/800?image=656"> </a> </li> </ul> </div>
2. The basic CSS styles for the photo gallery.
#photos img { width: 30%; float: left; display: block; margin: 2px; } #photos ul { list-style: none; margin: 0px auto; padding: 10px; display: block; max-width: 780px; text-align: center; } #photos { width: 100%; } #photo-gallery { width: 100%; }
3. The required CSS styles for the image lightbox & overlay.
#overlay { background: rgba(0,0,0, .8); width: 100%; height: 100%; position: absolute; top: 0; left: 0; display: none; text-align: center; } #overlay img { margin: 10% auto 0; width: 550px; border-radius: 5px; }
4. Enable the image lightbox. Add the following JS snippet after jQuery JavaScript library.
var $overlay = $('<div id="overlay"></div>'); var $image = $("<img>"); //An image to overlay $overlay.append($image); //Add overlay $("body").append($overlay); //click the image and a scaled version of the full size image will appear $("#photo-gallery a").click( function(event) { event.preventDefault(); var imageLocation = $(this).attr("href"); //update overlay with the image linked in the link $image.attr("src", imageLocation); //show the overlay $overlay.show(); } ); $("#overlay").click(function() { $( "#overlay" ).hide(); });
This awesome jQuery plugin is developed by mglissmann. For more Advanced Usages, please check the demo page or visit the official website.