Coverflow-like 3D Carousel Gallery with jQuery and CSS3
File Size: | 5.39 KB |
---|---|
Views Total: | 5545 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
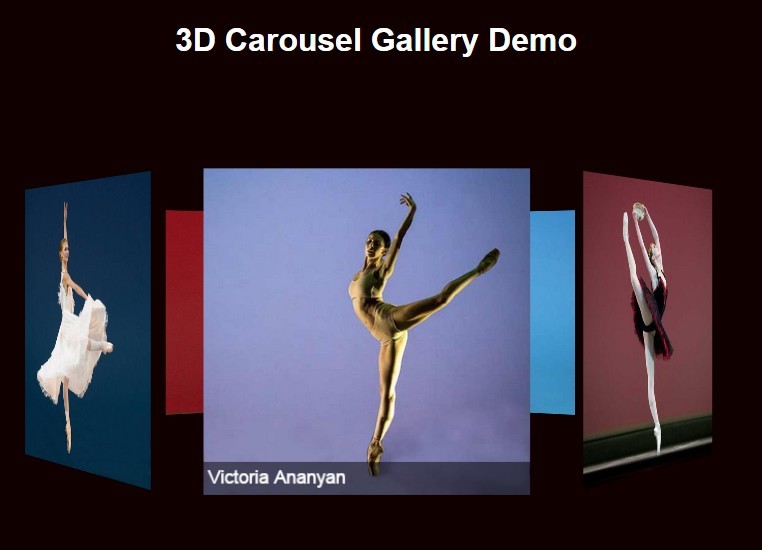
A jQuery, HTML5, CSS / CSS3 based carousel gallery which allows you to rotate infinitely through a series of images with keyboard interaction. A little similar to the Apple's coverflow design pattern.
Controls:
- Left / right keys or click on arrows to rotate
- Z or click to toggle zoom on foreground image
- C or hover to show/hide caption
How to use it:
1. Add images & captions into the 3D carousel gallery using Html5 figure
, figcaption
tags.
<div id="carousel"> <figure id="spinner"> <figure> <img src="1.jpg" alt="Alt 1"> <figcaption>Caption 1</figcaption> </figure> <figure> <img src="2.jpg" alt="Alt 2"> <figcaption>Caption 1</figcaption> </figure> <figure> <img src="3.jpg" alt="Alt 3"> <figcaption>Caption 3</figcaption> </figure> ... </div>
2. The core CSS / CSS3 styles.
* { box-sizing: border-box; } div#carousel { perspective: 1200px; background: #100000; padding-top: 5%; font-size: 0; margin-bottom: 3rem; } figure#spinner { transform-style: preserve-3d; height: 300px; transform-origin: 50% 50% -400px; transition: 1s; } figure#spinner figure { width: 20%; max-width: 500px; position: absolute; left: 40%; transform-origin: 50% 50% -400px; outline: 1px solid transparent; overflow: hidden; transition: 1s; } figure#spinner figure.focus { width: 26%; left: 37%; margin-top: -3rem; } figure#spinner figure img { width: 100%; } figcaption { position: absolute; top: 100%; width: 100%; padding: .3rem; z-index: 2; font-size: 1.1rem; background: rgba(0, 0, 0, 0); transition: .6s; } figure#spinner figure.current:hover figcaption, figure#spinner figure.current.caption figcaption { top: 90%; background: rgba(0, 0, 0, 0.5); bottom: 0; } div#carousel ~ span { color: #fff; margin: 5%; display: inline-block; text-decoration: none; font-size: 2rem; transition: 0.6s color; position: relative; margin-top: -6rem; border-bottom: none; line-height: 0; } div#carousel ~ span:hover { color: #888; cursor: pointer; }
3. Load the latest jQuery JavaScript library at the bottom of the web page.
<script src="//code.jquery.com/jquery-2.1.4.min.js"></script>
4. The JavaScript to enable the 3D carousel gallery.
var forEach = function (array, callback, scope) { for (var i = 0; i < array.length; i++) { callback.call(scope, i, array[i]); } }; var spinner = document.querySelector("#spinner"), angle = 0, images = document.querySelectorAll("#spinner figure"), numpics = images.length, degInt = 360 / numpics, start = 0, current = 1; forEach (images, function (index, value) { images[index].style.webkitTransform = "rotateY(-"+start+"deg)"; images[index].style.transform = "rotateY(-"+start+"deg)"; images[index].addEventListener("click", function() { if (this.classList.contains('current')) { this.classList.toggle("focus"); } }) start = start + degInt; }); function setCurrent(current) { document.querySelector('figure#spinner figure:nth-child('+current+')').classList.add('current'); } function galleryspin(sign) { forEach (images, function (index, value) { images[index].classList.remove('current'); images[index].classList.remove('focus'); images[index].classList.remove('caption'); }) if (!sign) { angle = angle + degInt; current = (current+1); if (current > numpics) { current = 1; } } else { angle = angle - degInt; current = current - 1; if (current == 0) { current = numpics; } } spinner.setAttribute("style","-webkit-transform: rotateY("+ angle +"deg); transform: rotateY("+ angle +"deg)"); setCurrent(current); } document.body.onkeydown = function(e){ switch(e.which) { case 37: // left cursor galleryspin('-'); break; case 39: // right cursor galleryspin(''); break; case 90: // Z - zoom image in forefront image document.querySelector('figure#spinner figure.current').classList.toggle('focus'); break; case 67: // C - show caption for forefront image document.querySelector('figure#spinner figure.current').classList.toggle('caption'); break; default: return; // exit this handler for other keys } e.preventDefault(); } setCurrent(1);
This awesome jQuery plugin is developed by dudleystorey. For more Advanced Usages, please check the demo page or visit the official website.