Gallery Content Filter With jQuery And CSS3
File Size: | 1.91 KB |
---|---|
Views Total: | 18319 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
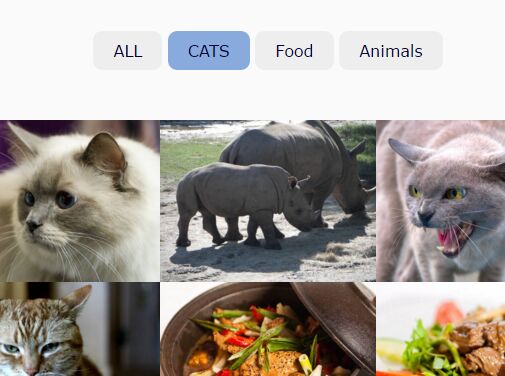
A jQuery based content filter which allows you to filter through a gallery of images with smooth CSS3 transition effects.
How to use it:
1. Insert a group of images with links to the gallery and categorize them using CSS classes like this:
<div class="gallery"> <a href="#" class="cats" ><img src="http://lorempixel.com/640/480/cats/1"></a> <a href="#" class="animals"><img src="http://lorempixel.com/640/480/animals/1"></a> <a href="#" class="cats" ><img src="http://lorempixel.com/640/480/cats/2"></a> <a href="#" class="cats" ><img src="http://lorempixel.com/640/480/cats/3"></a> <a href="#" class="food" ><img src="http://lorempixel.com/640/480/food/1"></a> <a href="#" class="food" ><img src="http://lorempixel.com/640/480/food/2"> <a href="#" class="animals" ><img src="http://lorempixel.com/640/480/animals/2"></a> <a href="#" class="food" ><img src="http://lorempixel.com/640/480/food/3"></a> <a href="#" class="animals" ><img src="http://lorempixel.com/640/480/animals/3"></a> </div>
2. Create filter controls.
<div class="filter"> <a href="#all">ALL</a> <a href="#cats">CATS</a> <a href="#food">Food</a> <a href="#animals">Animals</a> </div>
3. Style the gallery and filter controls.
.filter a { padding: 10px 20px; display: inline-block; color: #003; background: #eee; text-decoration: none; transition: all 0.2s; border-radius: 9px } .filter a:hover { background: #8ad } .filter { padding: 50px; text-align: center } .gallery a img { width: 100%; height: auto; float: left; } .gallery a { width: 33.33%; transition: all 0.2s; display: block; float: left; opacity: 1; height: auto; } .gallery .hide, .gallery .pophide { width: 0%; opacity: 0; transition: all 0.1s; } .gallery .pop { width: 100%; position: relative; z-index: 2; box-shadow: 0 0 0px 1000px rgba(0,0,0,0.5); } .pop:after { content: "\00D7"; position: absolute; top: 10px; right: 10px; color: #333; background: #fff; padding: 10px 15px; border-radius: 50%; opacity: 0.8; } .pop:hover:after { opacity: 1 }
4. Load the latest version of jQuery library at the end of the html document.
<script src="//code.jquery.com/jquery-2.2.1.min.js"></script>
5. The JavaScript to active the gallery content filter.
$('.filter a').click(function(e) { e.preventDefault(); var a = $(this).attr('href'); a = a.substr(1); $('.gallery a').each(function() { if (!$(this).hasClass(a) && a != 'all') $(this).addClass('hide'); else $(this).removeClass('hide'); }); }); $('.gallery a').click(function(e) { e.preventDefault(); var $i = $(this); $('.gallery a').not($i).toggleClass('pophide'); $i.toggleClass('pop'); });
This awesome jQuery plugin is developed by nodws. For more Advanced Usages, please check the demo page or visit the official website.