Responsive Hexagon Gallery with jQuery and CSS3
File Size: | 2.75 KB |
---|---|
Views Total: | 15311 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
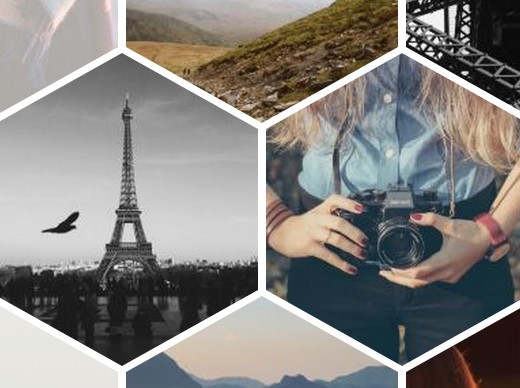
This is a jQuery & CSS3 based responsive hexagon grid layout for your image gallery that uses Geensock's TweenMax for fancy hierarchical timing animations.
See also:
- jQuery Plugin For Responsive Hexagon Grid Layout - Honeycombs
- Fancy jQuery & CSS3 Based Hexagon Image Gallery
- Fancy & Responsive jQuery Diamond Layout Plugin - diamonds.js
How to use it:
1. Create a hexagon grid layout from an html unordered list.
<div class="hex-container"> <ul class="hex-grid"> <li class="hex-grid__item"> Item 1 </li> <li class="hex-grid__item"> Item 2 </li> <li class="hex-grid__item"> Item 3 </li> ... <li class="hex-grid__item"> Item n </li> </ul> </div>
2. The CSS rules to style the hexagon grid.
.hex-container { overflow: hidden; } .hex-grid { list-style: none; margin: 0; padding: 0; width: 5000px; } .hex-grid__item { float: left; width: 200px; width: calc(100vw / 6); -webkit-clip-path: polygon(50% 0%, 100% 25%, 100% 75%, 50% 100%, 0% 75%, 0% 25%); clip-path: polygon(50% 0%, 100% 25%, 100% 75%, 50% 100%, 0% 75%, 0% 25%); border-left: 2px solid #fff; border-right: 2px solid #fff; -webkit-transform: translateZ(0); transform: translateZ(0); } .hex-grid__item:hover { opacity: 0.4; }
3. Make it responsive.
@media (max-width: 768px) { .hex-grid { width: 100%; } } @media (min-width: 1200px) { .hex-grid__item { border-left: 4px solid #fff; border-right: 4px solid #fff; } } @media (max-width: 768px) { .hex-grid__item { width: calc(100vw / 2); margin-bottom: calc(100vw / -7.5); } .hex-grid__item:nth-child(3n+1) { margin-left: calc(100vw / 4); clear: left; opacity: 0.4; } .hex-grid__item:nth-child(3n+1):last-child { margin-bottom: 0; } } @media (min-width: 768px) { .hex-grid__item:nth-child(7), .hex-grid__item:nth-child(14) { clear: left; } .hex-grid__item:nth-child(1), .hex-grid__item:nth-child(6), .hex-grid__item:nth-child(7), .hex-grid__item:nth-child(13), .hex-grid__item:nth-child(14), .hex-grid__item:nth-child(19) { opacity: 0.4; z-index: -1; } .hex-grid__item:nth-child(n+7):nth-child(-n+13) { position: relative; left: -105px; left: calc(100vw/-12); top: -58px; top: calc(100vw/-24); margin-bottom: -111px; margin-bottom: calc(100vw/-12); } }
4. Include jQuery library and TweenMax.js at the bottom of the html page.
<script src="/path/to/jquery-2.1.4.min.js"></script> <script src="/path/to/TweenMax.min.js"></script>
5. The core JavaScript for the hexagon grid layout.
var HexGrid = { $container: $('.hex-container'), $gridItems: $('.hex-grid__item:not(.no-refresh)'), animation: { duration: 0.5, visible: { autoAlpha: 1, delay: 0.05, scale: 1 }, hidden: { autoAlpha: 0, scale: 0.8 } }, init: function() { this.$refreshGrid; }, calculate: function() { var w = this.$container.width(), rowCount = 6, $newRow = $('.hex-grid__item:nth-child(n+7):nth-child(-n+13)'); $('.hex-grid__item').css('width', w / rowCount); $newRow.css({ 'left': w / -(rowCount * 2), 'top': w / -(rowCount * 4), 'margin-bottom': w / -(rowCount * 2) }); }, refreshGrid: function(e) { var _ = HexGrid, i = 0; e.preventDefault(); _.animation.visible.delay = 0.3; TweenLite.to(_.$gridItems, _.animation.duration, _.animation.hidden); for (i; i < _.$gridItems.length; i++) { _.animation.visible.delay += 0.05; TweenLite.to(_.$gridItems[i], _.animation.duration, _.animation.visible); } } };
6. Initialize the hexagon grid layout.
HexGrid.init();
This awesome jQuery plugin is developed by tjFogarty. For more Advanced Usages, please check the demo page or visit the official website.