Stylish Context Menu With jQuery And CSS
File Size: | 3.86 KB |
---|---|
Views Total: | 3011 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
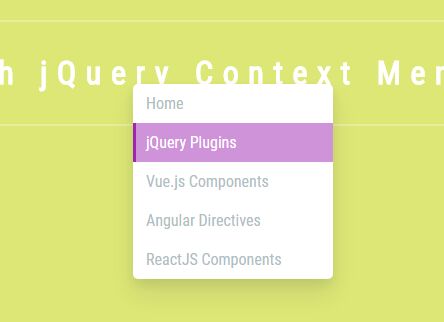
This is a lightweight jQuery snippet to create an easy-to-style context menu instead of the browser's default right click menu. Great for context menus, drop down lists, site navigations, and more.
How to use it:
1. Create the context menu from an unordered html list.
<ul class="contextmenu"> <li><a href="#">Home</a></li> <li><a href="#">jQuery Plugins</a></li> <li><a href="#">Vue.js Components</a></li> <li><a href="#">Angular Directives</a></li> <li><a href="#">ReactJS Components</a></li> </ul>
2. Style the context menu whatever you like:
.contextmenu { display: none; position: absolute; width: 200px; margin: 0; padding: 0; background: #FFFFFF; border-radius: 5px; list-style: none; box-shadow: 0 15px 35px rgba(50,50,90,0.1), 0 5px 15px rgba(0,0,0,0.07); overflow: hidden; z-index: 999999; } .contextmenu li { border-left: 3px solid transparent; transition: ease .2s; } .contextmenu li a { display: block; padding: 10px; color: #B0BEC5; text-decoration: none; transition: ease .2s; } .contextmenu li:hover { background: #CE93D8; border-left: 3px solid #9C27B0; } .contextmenu li:hover a { color: #FFFFFF; }
3. Insert the needed jQuery library in the document.
<script src="//code.jquery.com/jquery-3.2.1.slim.min.js"></script>
4. The jQuery script to enable the context menu within the whole document.
$(document).ready(function(){ //Show contextmenu: $(document).contextmenu(function(e){ //Get window size: var winWidth = $(document).width(); var winHeight = $(document).height(); //Get pointer position: var posX = e.pageX; var posY = e.pageY; //Get contextmenu size: var menuWidth = $(".contextmenu").width(); var menuHeight = $(".contextmenu").height(); //Security margin: var secMargin = 10; //Prevent page overflow: if(posX + menuWidth + secMargin >= winWidth && posY + menuHeight + secMargin >= winHeight){ //Case 1: right-bottom overflow: posLeft = posX - menuWidth - secMargin + "px"; posTop = posY - menuHeight - secMargin + "px"; } else if(posX + menuWidth + secMargin >= winWidth){ //Case 2: right overflow: posLeft = posX - menuWidth - secMargin + "px"; posTop = posY + secMargin + "px"; } else if(posY + menuHeight + secMargin >= winHeight){ //Case 3: bottom overflow: posLeft = posX + secMargin + "px"; posTop = posY - menuHeight - secMargin + "px"; } else { //Case 4: default values: posLeft = posX + secMargin + "px"; posTop = posY + secMargin + "px"; }; //Display contextmenu: $(".contextmenu").css({ "left": posLeft, "top": posTop }).show(); //Prevent browser default contextmenu. return false; }); //Hide contextmenu: $(document).click(function(){ $(".contextmenu").hide(); }); });
This awesome jQuery plugin is developed by tobiasdev. For more Advanced Usages, please check the demo page or visit the official website.