Highly Customizable Custom Overlay Scrollbar Plugin With jQuery
File Size: | 716 KB |
---|---|
Views Total: | 23641 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
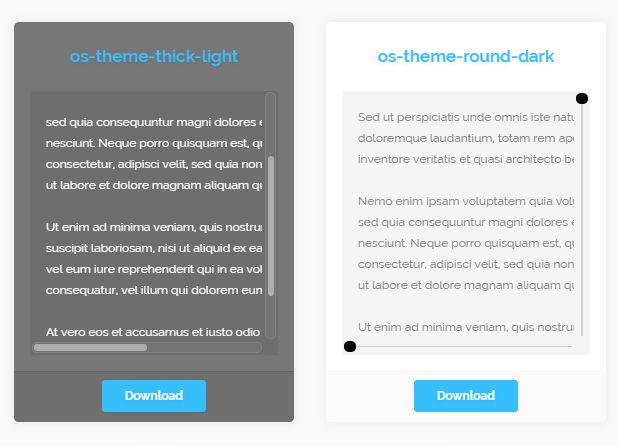
The jQuery OverlayScrollbars plugin provides a simple way to create customizable, themeable overlay scrollbars on any scrollable element while preserving the native scroll functionality.
The DOC of vanilla JS version is available here.
Features:
- Touch-enabled.
- RTL supported.
- CSS3 animated.
- Cross browser.
- High performance.
Basic usage:
1. Include the main stylesheet and a theme CSS of your choice on the head section of the webpage.
<!-- Main --> <link href="css/OverlayScrollbars.css" rel="stylesheet"> <!-- round dark --> <link href="css/os-theme-round-dark.css" rel="stylesheet"> <!-- round light --> <link href="css/os-theme-round-light.css" rel="stylesheet"> <!-- block dark --> <link href="css/os-theme-block-dark.css" rel="stylesheet"> <!-- block light --> <link href="css/os-theme-block-light.css" rel="stylesheet"> <!-- minimal dark --> <link href="css/os-theme-minimal-dark.css" rel="stylesheet"> <!-- minimal light --> <link href="css/os-theme-minimal-light.css" rel="stylesheet"> <!-- thick dark --> <link href="css/os-theme-thick-dark.css" rel="stylesheet"> <!-- thick light --> <link href="css/os-theme-thick-light.css" rel="stylesheet"> <!-- thin dark --> <link href="css/os-theme-thin-dark.css" rel="stylesheet"> <!-- thin light --> <link href="css/os-theme-thin-light.css" rel="stylesheet">
2. Include both jQuery JavaScript library and the jquery.overlayScrollbars.js
script on the page.
<script src="https://code.jquery.com/jquery-1.12.4.min.js" integrity="sha384-nvAa0+6Qg9clwYCGGPpDQLVpLNn0fRaROjHqs13t4Ggj3Ez50XnGQqc/r8MhnRDZ" crossorigin="anonymous"></script> <script src="js/jquery.overlayScrollbars.js"></script>
3. Call the function on the target scrollable element and specify the theme Class you want to use. Available themes:
- os-theme-dark: built-in
- os-theme-light: built-in
- os-theme-minimal-dark
- os-theme-minimal-light
- os-theme-thin-dark
- os-theme-thin-light
- os-theme-thick-dark
- os-theme-thick-light
- os-theme-round-dark
- os-theme-round-light
- os-theme-block-dark
- os-theme-block-light
$('body').overlayScrollbars({ className: "os-theme-dark" });
4. More configuration options to customize the scrollbars.
$('body').overlayScrollbars({ // none || both || horizontal || vertical || n || b || h || v resize : 'none', // true || false sizeAutoCapable : true, // true || false clipAlways : true, // true || false normalizeRTL : true, // true || false paddingAbsolute : false, // true || false || null autoUpdate : null, // number autoUpdateInterval : 33, // Control on which elements / selectors OverlayScrollbars shall update automatically after the emit of a load event. // You can set it to null to disable this auto updating entierly or add your own selectors to update only on special img elements or on for example loaded iframes. updateOnLoad: ['img'], // These options are only respected if the native scrollbars are overlaid. nativeScrollbarsOverlaid : { showNativeScrollbars : false, //true || false initialize : true //true || false }, // Defines how the overflow should be handled for each axis overflowBehavior : { // visible-hidden || visible-scroll || hidden || scroll || v-h || v-s || h || s x : 'scroll', // visible-hidden || visible-scroll || hidden || scroll || v-h || v-s || h || s y : 'scroll' }, // Defines the behavior of the custom scrollbars. scrollbars : { visibility : 'auto', //visible || hidden || auto || v || h || a autoHide : 'never', //never || scroll || leave || n || s || l autoHideDelay : 800, //number dragScrolling : true, //true || false clickScrolling : false, //true || false touchSupport : true, //true || false snapHandle: true //true || false }, // Defines special behavior of textarea elements. textarea : { dynWidth : false, //true || false dynHeight : false, //true || false inheritedAttrs : inheritedAttrsTemplate //string || array || null } });
5. Callback functions.
$('body').overlayScrollbars({ callbacks : { onInitialized : null, //null || function onInitializationWithdrawn : null, //null || function onDestroyed : null, //null || function onScrollStart : null, //null || function onScroll : null, //null || function onScrollStop: null, //null || function onOverflowChanged : null, //null || function onOverflowAmountChanged : null, //null || function onDirectionChanged : null, //null || function onContentSizeChanged : null, //null || function onHostSizeChanged : null, //null || function onUpdated : null //null || function } });
6. API methods.
var instance = $('body').overlayScrollbars({ // options here }); // adds new options instance.options(optionName, optionValue) // updates scrollbars instance.update([force]) // puts the instance to sleep instance.sleep() // returns the current scroll information. instance.scroll() // sets the scroll position. instance.scroll(coordinates [, duration] [, easing] [, complete]) // stops the current scroll-animation. instance.scrollStop() // returns all relevant elements. instance.getElements() // returns a object which describes the current state of this instance. instance.getState() // removes scrollbars from DOM instance.destroy() // checks whether a passed object is a non-destroyed OverlayScrollbars instance instance.valid()
Changelog:
v2.1.0 (2023-02-06)
- Major update. Click here to see details.
v1.13.0 (2020-08-03)
- If you drag the scrollbar handle the click event won't be propagated to the body to be closer to the native behavior.
- The .os-padding element has now default z-index.
- Clickscrolling amount & speed adjusts now to the scrollbar-handle size to be more accurate.
- The RTL(right to left) style won't be applied to the body element anymore to be closer to the native behavior.
v1.11.0 (2020-04-06)
- 'max-content' is now used to detect the possible size if width is not fixed. (only if supported by the browser else the old algo. is used)
- Updated all wrapper versions to better support frontend frameworks.
- Removed useless touchevents from the host element
- A new option called 'updateOnLoad' with which you can control on which elements / selectors OverlayScrollbars shall update automatically after the emit of a load event. Per default the value is set to ["img"] so the plugin will updated after any img emits a load event. You can set it to null to disable this auto updating entierly or add your own selectors to update only on special img elements or on for example loaded iframes.
- Bugfixes
v1.11.0 (2020-03-01)
- Changed RTL behavior detection to support the Chromium web interoperability effort
- Implemented a way to intuitively set the tabindex attribute of the viewport element
- Changed restrictedMeasuring workaround (works via CSS now).
- Removed unnecessary CSS
- If ResizeObserver is supported, it now detects changes in padding in Chrome again.
v1.10.3 (2020-02-03)
- Fixed: Reference Error: previousOverflow is not defined
- The cache of the scroll infos which can be get by the scroll method is now updated immediately after you use the scroll method to change the position.
v1.9.0 (2019-07-27)
- Fixed a bug where unexpected scroll jumps happened after you changed a option.
- Fixed a bug where a min-width change wasn't detected if width is auto
- Fixed a bug where the update function didn't update a textarea properly, when only its value changed and due to that its size.
- A new global method OverlayScrollbars.valid which checks whether a passed object is a non-destroyed OverlayScrollbars instance.
v1.8.0 (2019-07-09)
- The getState() methods returned object contains now a new property called destroyed which indicates that the instance has been destroyed.
- Changed the management of passive event listeners: touch events which call prefentDefault() are now added with passive : false, all other events which should be passive and don't call prefentDefault() are added with passive : true.
- Preparation for framework wrappers such as react, vue and angular: the option() method will now only cause a call to the update() method if at least one option has been truly changed.
- Fixed bugs.
- Cleaner handling of the internal update() method.
v1.7.3 (2019-06-24)
- Bugfixes.
v1.7.0 (2019-04-19)
- A new option scrollbars.snapHandle was added which helps you to control how the scrollbar-handle behaves if you use CSS Snap Scrolling.
- The scroll() methods returned object now has a new property called snappedHandleOffset.
- CSS Snap Scrolling has now deeper support: The handle dragging is now smooth (controlled with the new option scrollbars.snapHandle) and the jump-back if you let go midway has now a transition.
- If you set a handle-max-size and the overflow is way to small, the resulting jump-back if you let go midway has now a transition.
- If you drag the handle the next calculated scroll-position is now rounded instead of floored which results in better user-experience.
- Click scrolling is now more precise.
- Bugs fixed.
2019-01-17
- v1.6.2
This awesome jQuery plugin is developed by KingSora. For more Advanced Usages, please check the demo page or visit the official website.