Cross-browser Custom Scrollbar In JavaScript - jScrollPane
File Size: | 195 KB |
---|---|
Views Total: | 3542 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
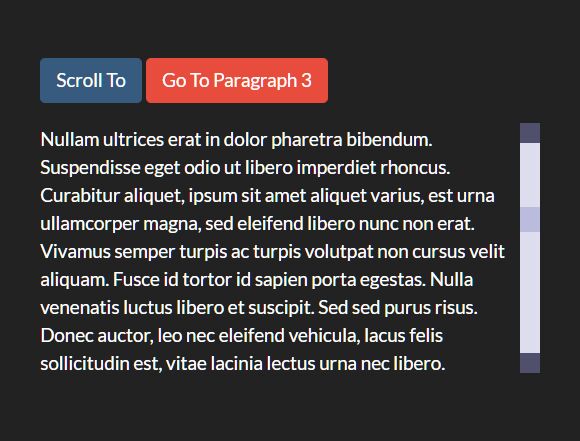
jScrollPane is a jQuery plugin to create cross-browser, fully configurable, highly customizable scrollbars on any container elements.
Supports any scrollable containers such as DIVs, iframes, and even the whole body.
Created for non-WebKit browsers that don't support custom browser scrollbars using pseudo elements.
More features:
- Supports both horizontal and vertical scrollbars.
- Supports mouse wheel event. Requires mousewheel plugin.
- Auto scrolls the content when hovering over scroll arrows.
- Auto maintains the position whenever it is reinitialised.
- Fully responsive. Auto reinit on window resize.
- Auto scrolls the content to the correct place on page load. Smooth scroll is supported as well.
Table Of Contents:
How to use it:
1. Include jQuery library and the jScrollPane plugin's files on the page.
<link rel="stylesheet" href="/path/to/style/jquery.jscrollpane.css" /> <script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/script/jquery.jscrollpane.js"></script>
2. Include the mousewheel plugin. OPTIONAL.
<script src="/path/to/cdn/mousewheel.min.js"></script>
3. Include the mwheelIntent.js plugin to detect the user intent. OPTIONAL.
<script src="/path/to/script/mwheelIntent.js"></script>
4. Create a scrollable container.
<div class="scroll-pane"> ... content here ... </div>
.scroll-pane { width: 300px; height: 200px; overflow: auto; }
5. Attach the jScrollPane plugin to the scrollable content and done.
var pane = $('.scroll-pane'); pane.jScrollPane( { // options here } );
6. All plugin options to customize the scrollbar.
// shows scroll arrows showArrows: false, // maintains the position whenever it is reinitialised maintainPosition: true, // scroll to the bottom even if new content is added to the pane stickToBottom: false, // scroll to the right even if new content is added to the pane stickToRight: false, // allows to scroll towards the point you click clickOnTrack: true, // auto re-init (e.g. new content is added to the pane) autoReinitialise: false, // time to wait before auto re-init autoReinitialiseDelay: 500, // the smallest height that the vertical drag can have verticalDragMinHeight: 0, // the max height that the vertical drag can have verticalDragMaxHeight: 99999, // the smallest width that the horizontal drag can have horizontalDragMinWidth: 0, // the max width that the horizontal drag can have horizontalDragMaxWidth: 99999, // content width contentWidth: undefined, // enables smooth scroll animateScroll: false, // duration of smooth scroll animateDuration: 300, // easing for smooth scroll animateEase: 'linear', // enables a link to scroll the pane to specific anchor hijackInternalLinks: false, // vertical/horizontal gutter verticalGutter: 4, horizontalGutter: 4, // mousewheel speed mouseWheelSpeed: 3, // the amount that the scrollpane scrolls each time on of the arrow buttons is pressed arrowButtonSpeed: 0, // the number of milliseconds between each repeated scroll event when the mouse is held down over one of the arrow keys arrowRepeatFreq: 50, // auto scrolls the content when hovering over the scroll arrows arrowScrollOnHover: false, // controls the amount that the scrollpane scrolls each trackClickRepeatFreq while the mouse button is held down over the track trackClickSpeed: 0, // the number of milliseconds between each repeated scroll event when the mouse is held down over the track trackClickRepeatFreq: 70, // split|before|after|os verticalArrowPositions: 'split', horizontalArrowPositions: 'split', // enables keyboard navigation enableKeyboardNavigation: true, keyboardSpeed: 0, // hides focus outline hideFocus: false, // Delay before starting repeating initialDelay: 300, // Default speed when others falsey speed: 30, // Percent of visible area scrolled when pageUp/Down or track area pressed scrollPagePercent: 0.8, // always shows vertical scrollbar alwaysShowVScroll: false, // always shows horizontal scrollbar alwaysShowHScroll: false, // auto re-init on content resize resizeSensor: false, // time to wait before re-init on resize resizeSensorDelay: 0,
6. API methods.
var api = pane.data('jsp'); // re-init the instance api.reinitialise(delay); // scrolls to a specific element api.scrollToElement(ele, stickToTop, animate); // scrolls to a specific point api.scrollTo(destX, destY, animate); api.scrollToX(destX, animate); api.scrollToY(destY, animate); api.scrollToPercentX(destPercentX, animate); api.scrollToPercentY(destPercentY, animate); // scrolls the pane by the specified amount of pixels api.scrollBy(deltaX, deltaY, animate); api.scrollByX(deltaX, animate); api.scrollByY(deltaY, animate); // Positions the horizontal drag at the specified x position api.positionDragX(x, animate); // Positions the vertical drag at the specified y position (and updates the viewport to reflect this) api.positionDragY(y, animate); // animates to a new position api.animate(ele, prop, value, stepCallback, completeCallback); // scrolls to the bottom api.scrollToBottom(animate); // hijacks the links on the page which link to content inside the scrollpane api.hijackInternalLinks(); // get data api.getContentPositionX(); api.getContentPositionY(); api.getContentWidth(); api.getContentHeight(); api.getIsScrollableH(); api.getPercentScrolledX(); api.getPercentScrolledY(); api.getIsScrollableV(); api.getContentPane(); // destroys the instance api.destroy();
This awesome jQuery plugin is developed by vitch. For more Advanced Usages, please check the demo page or visit the official website.