Customizable jQuery & Bootstrap Pagination Plugin - Bootstrap Paginator
File Size: | 329 KB |
---|---|
Views Total: | 7882 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
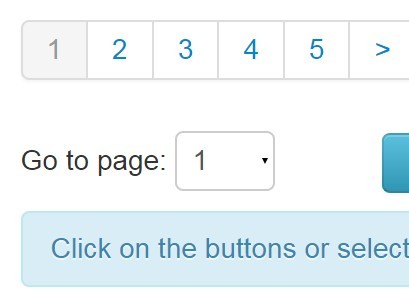
Bootstrap Paginator is a jQuery plugin which uses Bootstrap's pagination component to generate a fully customizable, client side pagination for your long web content. Bootstrap Paginator is licensed under the Apache Software Foundation License Version 2.0.
Basic usage:
1. To use this plugin you first need to include jQuery JavaScript library and Twitter's Bootstrap framework in your document.
<link href="bootstrap.min.css" rel="stylesheet"> <script src="jquery.min.js"></script> <script src="bootstrap.min.js"></script>
2. Make sure to include the bootstrap-paginator.min.js after jQuery library.
<script src="js/bootstrap-paginator.min.js"></script>
3. Generate a basic pagination in your web page. In Bootstrap version 2 the pagination must be a div element. If you are using Bootstrap pagination 3, the pagination's wrapper must be an ul element.
<div id="example"></div>
var options = { currentPage: 2, totalPages: 5 } $('#example').bootstrapPaginator(options);
3. Customization options.
// container css class containerClass: "", // Controls the size of output pagination component. // small, normal, large size: "normal", // Controls the alignment of the pagination component. // left, center and right. alignment: "left", // Specifies the major version number of bootstrap so that the plugin know how to render the pagination. bootstrapMajorVersion: 2, // list container class listContainerClass: "", // Returns a string class name when rendering a specific page item. itemContainerClass: function (type, page, current) { return (page === current) ? "active" : ""; }, itemContentClass: function (type, page, current) { return ""; }, // The current page currentPage: 1, // How many pages should be rendered numberOfPages: 5, // Defines the upper limit of the page range. totalPages: 1, // Fills the href attribute when rendering the page items. pageUrl: function (type, page, current) { return null; }, // for page-clicked event onPageClicked: null, // for page-changed event onPageChanged: null, // Uses Bootstrap tooltip component useBootstrapTooltip: false, // Defines whether a page item should be shown. shouldShowPage: function (type, page, current) { var result = true; switch (type) { case "first": result = (current !== 1); break; case "prev": result = (current !== 1); break; case "next": result = (current !== this.totalPages); break; case "last": result = (current !== this.totalPages); break; case "page": result = true; break; } return result; }, // Plain text or html itemTexts: function (type, page, current) { switch (type) { case "first": return "<<"; case "prev": return "<"; case "next": return ">"; case "last": return ">>"; case "page": return page; } }, // tooltip text tooltipTitles: function (type, page, current) { switch (type) { case "first": return "Go to first page"; case "prev": return "Go to previous page"; case "next": return "Go to next page"; case "last": return "Go to last page"; case "page": return (page === current) ? "Current page is " + page : "Go to page " + page; } }, // Bootstrap tooltip options bootstrapTooltipOptions: { animation: true, html: true, placement: 'top', selector: false, title: "", container: false }
4. Public methods.
// shows the specified page .bootstrapPaginator("show",page); // shows the first page .bootstrapPaginator("showFirst"); // shows the previous page .bootstrapPaginator("showPrevious"); // shows the next page .bootstrapPaginator("showNext"); // shows the last page .bootstrapPaginator("showLast"); // returns an array with extra attributes that indicate the current status of the page. .bootstrapPaginator("getPages"); // updates options .bootstrapPaginator("setOptions", OPTIONS);
This awesome jQuery plugin is developed by lyonlai. For more Advanced Usages, please check the demo page or visit the official website.