Simple Calculator App Styled With Bootstrap 5
File Size: | 4.34 KB |
---|---|
Views Total: | 4029 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
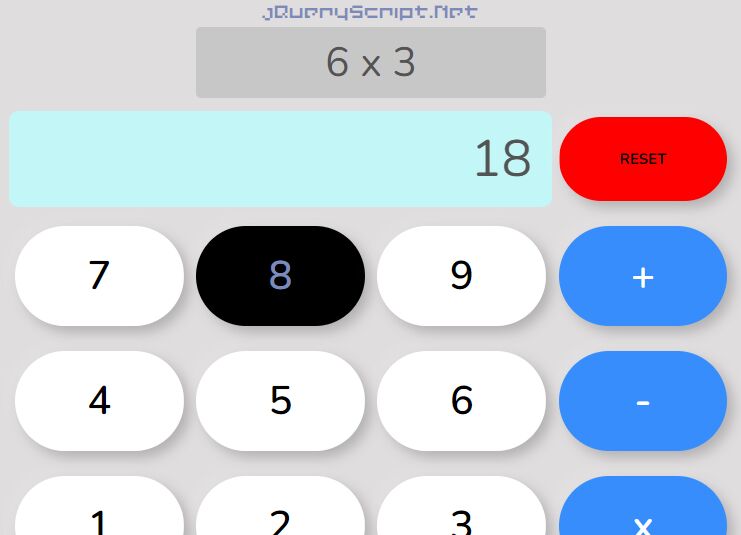
In this post, we're going to make a simple online calculator app styled with the latest Bootstrap 5 framework.
It is a calculator app that only accepts decimal digits. This will help you get started with Bootstrap 5 and understand how to style it into your own apps.
How to use it:
1. Load the required jQuery library and Bootstrap 5 stylesheet in the document.
<link rel="stylesheet" href="/path/to/cdn/bootstrap.min.css" /> <script src="/path/to/cdn/jquery.slim.min.js"></script>
2. Create the HTML for the calculator and style the buttons using the Bootstrap's button utility classes:
<div class="calculator-area mx-auto justify-content-center border border-primary text-center"> <div class="history col-12"> <div class="logo"> jQueryScript.Net </div> <div class="below-logo"> <input type="text" class="border border-primary form-control-sm text-center history-screen" disabled/> </div> </div> <div class="row"> <input type="text" class="input-screen border border-primary form-control-lg col-9 text-end" disabled/> <button type="button" class="btn btn-outline-danger reset col" value="reset">RESET</button> </div> <div class="row"> <button type="button" class="btn btn-outline-secondary col" value="7">7</button> <button type="button" class="btn btn-outline-secondary col" value="8">8</button> <button type="button" class="btn btn-outline-secondary col" value="9">9</button> <button type="button" class="btn btn-outline-primary col" value="+">+</button> </div> <div class="row"> <button type="button" class="btn btn-outline-secondary col" value="4">4</button> <button type="button" class="btn btn-outline-secondary col" value="5">5</button> <button type="button" class="btn btn-outline-secondary col" value="6">6</button> <button type="button" class="btn btn-outline-primary col" value="-">-</button> </div> <div class="row"> <button type="button" class="btn btn-outline-secondary col" value="1">1</button> <button type="button" class="btn btn-outline-secondary col" value="2">2</button> <button type="button" class="btn btn-outline-secondary col" value="3">3</button> <button type="button" class="btn btn-outline-primary col" value="x">x</button> </div> <div class="row"> <button type="button" class="btn btn-outline-secondary col mod" value="%">mod</button> <button type="button" class="btn btn-outline-secondary col" value="0">0</button> <button type="button" class="btn btn-outline-secondary col" value=".">.</button> <button type="button" class="btn btn-outline-primary col" value="/">÷</button> </div> <div class="row"> <button type="button" class="btn btn-outline-success col-8" value="=">=</button> <button type="button" class="btn btn-outline-primary col-3" value="+-">+/-</button> </div> </div>
3. Additional CSS styles for the calculator.
.input-screen { background-color: rgb(195, 247, 247); font-size: 2vw; } .calculator-area { width: 70%; height: 90%; margin: 6%; padding: 3%; background-color: #dfdddd; } .history-screen { background-color: #c7c7c7; font-size: 1.6vw; max-width: 50%; } .row { margin: 1%; padding: 1%; } .btn { margin: 1%; font-size: xx-large; } .reset { font-size: 15px; font-weight: bolder; } .mod { font-size: medium; font-weight: bolder; } .btn-outline-secondary { background: linear-gradient(to right, #000000 0%, #000000 50%, #ffffff 50%, #ffffff 100%); background-size: 200% 100%; background-position: 100% 0; transition: background-position 0.1s; -webkit-transition: all 1s; -moz-transition: all 1s; transition: all 1s; color: #000000; } .btn-outline-secondary:hover { background-position: 0 0; } .btn-outline-secondary:focus { color: #000000; } .btn-outline-danger { background: linear-gradient(to right, #f87171 0%, #f87171 50%, #ff0000 50%, #ff0000 100%); background-size: 200% 100%; background-position: 100% 0; transition: background-position 0.1s; -webkit-transition: all 1s; -moz-transition: all 1s; transition: all 1s; color: #000000; } .btn-outline-danger:hover { background-position: 0 0; } .btn-outline-danger:focus { color: white; } .outer { margin: 0; padding: 0; }
4. Enable the calculator.
<script src="js/app.js"></script>
Changelog:
2022-09-25
- JS & CSS updated
This awesome jQuery plugin is developed by boraxpr. For more Advanced Usages, please check the demo page or visit the official website.