Google App Launcher Style Dropdown Plugin with jQuery and CSS3
File Size: | 2.68 KB |
---|---|
Views Total: | 6359 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
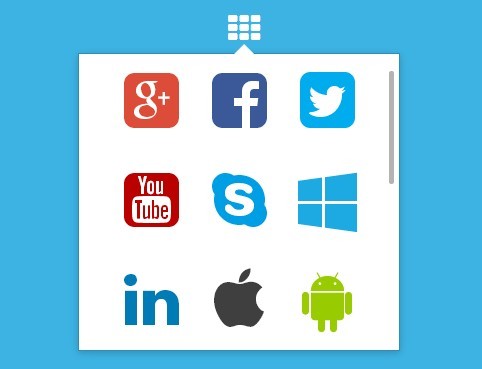
A toggleable and scrollable drop down interface which allows you to quickly launch your favorite apps, inspired by Google App launcher and built with jQuery & CSS3.
How to use it:
1. Load the Font Awesome 4 in the head section of your page (Optional. Just for App icons).
<link href="//netdna.bootstrapcdn.com/font-awesome/4.1.0/css/font-awesome.min.css" rel="stylesheet">
2. Create a scrollable dropdown with a toggle button that includes some popular App icons.
<div class="launcher"> <div class="button"> <i class="fa fa-th fa-2x"></i> </div> <div class="app-launcher"> <div class="apps"> <ul class="first-set"> <li><a href="#"><i class="fa fa-google-plus-square fa-4x"> </i></a></li> <li><a href="#"><i class="fa fa-facebook-square fa-4x"> </i></a> </li> <li><a href="#"><i class="fa fa-twitter-square fa-4x"> </i></a> </li> <li><a href="#"><i class="fa fa-youtube-square fa-4x"> </i></a> </li> <li><a href="#"><i class="fa fa-skype fa-4x"></i></a> </li> <li><a href="#"><i class="fa fa-windows fa-4x"></i></a> </li> <li><a href="#"><i class="fa fa-linkedin fa-4x"></i></a> </li> <li><a href="#"><i class="fa fa-apple fa-4x"></i></a> </li> <li><a href="#"><i class="fa fa-android fa-4x"></i></a> </li> </ul> <a href="#" class="more">More</a> <ul class="second-set hide"> <li><a href="#"><i class="fa fa-dribbble fa-4x"></i></a> </li> <li><a href="#"><i class="fa fa-html5 fa-4x"></i></a> </li> <li><a href="#"><i class="fa fa-linux fa-4x"></i></a> </li> <li><a href="#"><i class="fa fa-css3 fa-4x"></i></a> </li> <li><a href="#"><i class="fa fa-github fa-4x"></i></a> </li> <li><a href="#"><i class="fa fa-pinterest fa-4x"></i></a> </li> <li><a href="#"><i class="fa fa-tumblr-square fa-4x"></i></a> </li> <li><a href="#"><i class="fa fa-dropbox fa-4x"></i></a> </li> <li><a href="#"><i class="fa fa-instagram fa-4x"></i></a> </li> </ul> </div> </div> </div>
3. Style the dropdown in the your CSS.
.launcher { position: relative; text-align: center; color: white; } .app-launcher { position: absolute; left: 84px; top: 40px; } .app-launcher::before { content: ''; border-left: 10px solid transparent; border-right: 10px solid transparent; border-bottom: 10px solid white; position: absolute; top: -9px; left: 50%; margin-left: -5px; z-index: 1; } .apps { position: relative; border: 1px solid #ccc; border-color: rgba(0,0,0,.2); box-shadow: 0 2px 10px rgba(0,0,0,.2); -webkit-transition: height .2s ease-in-out; transition: height .2s ease-in-out; min-height: 196px; overflow-y: auto; overflow-x: hidden; width: 320px; height: 396px; margin-bottom: 30px; } .launcher .button { cursor: pointer; width: 32px; margin: 0 auto; } .hide { display: none; } .apps ul { background: #fff; margin: 0; padding: 28px; width: 264px; overflow: hidden; list-style: none; } .apps ul li { float: left; height: 64px; width: 88px; color: black; padding: 18px 0; text-align: center; } .apps .more { line-height: 40px; text-align: center; display: block; width: 320px; background: #f5f5f5; cursor: pointer; height: 40px; overflow: hidden; position: absolute; text-decoration: none; color: #282828; } .apps.overflow .more { border-bottom: 1px solid #ebebeb; left: 28px; width: 264px; height: 0; cursor: default; height: 0; outline: none; } .fa-facebook-square { color: #3b5998; } .fa-twitter-square { color: #00aced; } .fa-google-plus-square { color: #dd4b39; } .fa-youtube-square { color: #bb0000; } .fa-linkedin { color: #007bb6; } .fa-instagram { color: #517fa4; } .fa-pinterest { color: #cb2027; } .fa-tumblr-square { color: #32506d; } .fa-skype { color: #009EE5; } .fa-android { color: #99CC00; } .fa-dribbble { color: #C35D9C; } .fa-html5 { color: #F65B1F; } .fa-css3 { color: #0070BA; } .fa-dropbox { color: #65B1ED; } .fa-windows { color: #1DAAE2; } .fa-linux { color: #DD4814; } .fa-apple { color: #403F3F; } ::-webkit-scrollbar-thumb { background-clip: padding-box; background-color: rgba(0,0,0,.3); border: 5px solid transparent; border-radius: 10px; min-height: 20px; min-width: 20px; height: 5px; width: 5px; } ::-webkit-scrollbar { height: 15px; width: 15px; background: white; } ::-webkit-scrollbar-button { height: 0; width: 0; }
4. Include the latest version of jQuery library at the bottom of your webpage.
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
5. The Javascript to enable the droddown App launcher.
$(document).ready(function(){ var scroll = false; var launcherMaxHeight = 396; var launcherMinHeight = 296; // Mousewheel event handler to detect whether user has scrolled over the container $('.apps').bind('mousewheel', function(e){ if(e.originalEvent.wheelDelta /120 > 0) { // Scrolling up } else{ // Scrolling down if(!scroll){ $(".second-set").show(); $('.apps').css({height: launcherMinHeight}).addClass('overflow'); scroll =true; $(this).scrollTop(e.originalEvent.wheelDelta); } } }); // Scroll event to detect that scrollbar reached top of the container $('.apps').scroll(function(){ var pos=$(this).scrollTop(); if(pos == 0){ scroll =false; $('.apps').css({height: launcherMaxHeight}).removeClass('overflow'); $(".second-set").hide(); } }); // Click event handler to show more apps $('.apps .more').click(function(){ $(".second-set").show(); $(".apps").animate({ scrollTop: $('.apps')[0].scrollHeight}).css({height: 296}).addClass('overflow'); }); // Click event handler to toggle dropdown $(".button").click(function(event){ event.stopPropagation(); $(".app-launcher").toggle(); }); $(document).click(function() { //Hide the launcher if visible $('.app-launcher').hide(); }); // Prevent hiding on click inside app launcher $('.app-launcher').click(function(event){ event.stopPropagation(); }); }); // Resize event handler to maintain the max-height of the app launcher $(window).resize(function(){ $('.apps').css({maxHeight: $(window).height() - $('.apps').offset().top}); });
This awesome jQuery plugin is developed by izerox. For more Advanced Usages, please check the demo page or visit the official website.