CSS3 Animated Tabs Plugin - jQuery Tabify
File Size: | 7.44 MB |
---|---|
Views Total: | 613 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
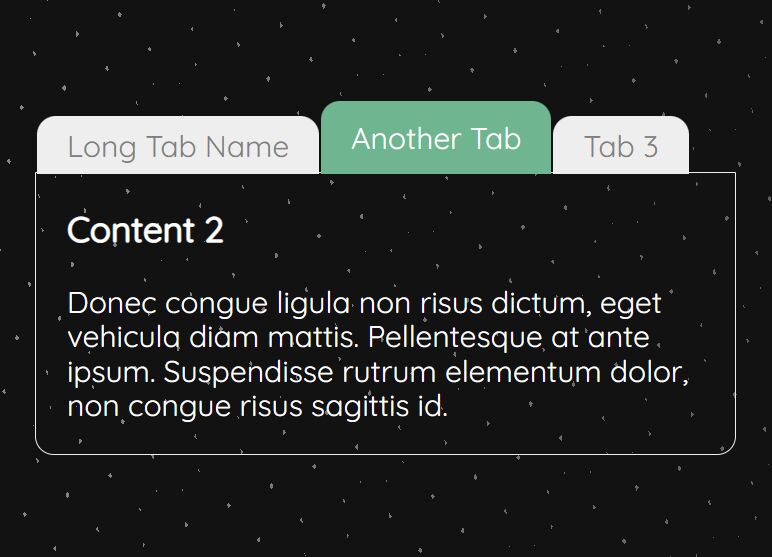
The Tabify jQuery plugin is a simple implementation of tabs with slick CSS3 animations. The plugin is pretty much the bare minimum you need to get a nice looking tabs system working. It has all the features you need, easy styling, and it's fully responsive.
How to use it:
1. The required HTML structure for the tabs system.
<div class="tab-group"> <section id="tab1" title="Long Tab Name"> <h3> Content 1 </h3> <p> Aliquam eleifend magna mauris, id egestas eros dictum ac. Vivamus ac turpis at nisi mattis aliquam. In hac habitasse platea dictumst. </p> </section> <section id="tab2" title="Another Tab"> <h3> Content 2 </h3> <p> Donec congue ligula non risus dictum, eget vehicula diam mattis. Pellentesque at ante ipsum. Suspendisse rutrum elementum dolor, non congue risus sagittis id. </p> </section> <section id="tab3" title="Tab 3"> <h3> Content 3 </h3> <p> Vivamus sem odio, mattis vel dui aliquet, iaculis lacinia nibh. Vestibulum tincidunt, lacus vel semper pretium, nulla sapien blandit massa, et tempor turpis urna eu mi. </p> </section> ... </div>
2. Basic tabs styling. Feel free to override the followng CSS snippets to create your own styles.
.tab-group { position: relative; border: 1px solid #eee; margin-top: 2.5em; border-radius: 0 0 10px 10px; } .tab-group section { opacity: 0; height: 0; padding: 0 1em; overflow: hidden; transition: opacity 0.4s ease, height 0.4s ease; } .tab-group section.active { opacity: 1; height: auto; overflow: visible; } .tab-nav { list-style: none; margin: -2.5em -1px 0 0; padding: 0; height: 2.5em; overflow: hidden; } .tab-nav li { display: inline; } .tab-nav li a { top: 1px; position: relative; display: block; float: left; border-radius: 10px 10px 0 0; background: #eee; line-height: 2em; padding: 0 1em; text-decoration: none; color: grey; margin-top: .5em; margin-right: 1px; transition: background .2s ease, line-height .2s ease, margin .2s ease; } .tab-nav li.active a { background: #6EB590; color: white; line-height: 2.5em; margin-top: 0; }
3. Load the requried jQuery library right before the closing body tag.
<script src="/path/to/cdn/jquery.slim.min.js"></script>
4. The main Tabify() function.
;(function(defaults, $, window, document, undefined) { 'use strict'; $.extend({ // Function to change the default properties of the plugin // Usage: // jQuery.tabifySetup({property:'Custom value'}); tabifySetup : function(options) { return $.extend(defaults, options); } }).fn.extend({ // Usage: // jQuery(selector).tabify({property:'value'}); tabify : function(options) { options = $.extend({}, defaults, options); return $(this).each(function() { var $element, tabHTML, $tabs, $sections; $element = $(this); $sections = $element.children(); // Build tabHTML tabHTML = '<ul class="tab-nav">'; $sections.each(function() { if ($(this).attr("title") && $(this).attr("id")) { tabHTML += '<li><a href="#' + $(this).attr("id") + '">' + $(this).attr("title") + '</a></li>'; } }); tabHTML += '</ul>'; // Prepend navigation $element.prepend(tabHTML); // Load tabs $tabs = $element.find('.tab-nav li'); // Functions var activateTab = function(id) { $tabs.filter('.active').removeClass('active'); $sections.filter('.active').removeClass('active'); $tabs.has('a[href="' + id + '"]').addClass('active'); $sections.filter(id).addClass('active'); } // Setup events $tabs.on('click', function(e){ activateTab($(this).find('a').attr('href')); e.preventDefault(); }); // Activate first tab activateTab($tabs.first().find('a').attr('href')); }); } }); })({ property : "value", otherProperty : "value" }, jQuery, window, document);
5. Initialize the tabs system. That's it.
$('.tab-group').tabify();
This awesome jQuery plugin is developed by altitudems. For more Advanced Usages, please check the demo page or visit the official website.