Minimal Toast Message Script Built Using jQuery
File Size: | 6.17 KB |
---|---|
Views Total: | 407 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
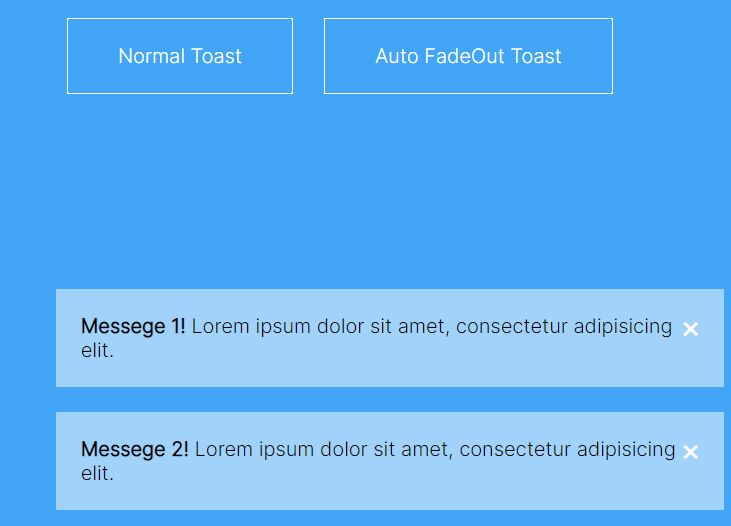
A small jQuery toast notification script that shows a quick message to the user below their current view, lasting only for a few seconds, or until the users manually close it.
How to use it:
1. Add toast messages to the page.
<div class="toast-container toast-pos-right toast-pos-bottom"> <!-- Toast --> <div class="toast" id="toast-name-1"> <a href="#" class="close-toast">✖</a> <b>Messege 1!</b> Lorem ipsum dolor sit amet, consectetur adipisicing elit. </div> <!-- Toast --> <div class="toast" id="toast-name-2"> <a href="#" class="close-toast">✖</a> <b>Messege 2!</b> Lorem ipsum dolor sit amet, consectetur adipisicing elit. </div> </div>
2. Create trigggers to toggle the toast messages.
- Use "toast-auto" class to force automatically notification fade out.
- Use "toast-pos-xxxxx" classes to place the 'toasts' container wherever you want.
<a href="#" class="toast-trigger" data-toast="toast-name-1"> Normal Toast </a> <a href="#" class="toast-trigger toast-auto" data-toast="toast-name-2"> Auto FadeOut Toast </a>
3. The necessary CSS styles for the toast messages.
.toast-container { width: 90%; max-width: 580px; margin: 0 auto; } [class*="toast-pos-"] { position: absolute; padding: 10px; } .toast-pos-top { top: 0; } .toast-pos-right { right: 0; } .toast-pos-bottom { bottom: 0; } .toast-pos-left { left: 0; } .toast { display: none; padding: 20px; margin: 20px 0; background: #eeeeee; color: #333333; } .close-toast { float: right; text-decoration: none; color: #ffffff; vertical-align: middle; }
4. The main script to enable the toast messages. Copy and insert the following snippets after the latest jQuery library. That's it.
<script src="/path/to/cdn/jquery.min.js"></script>
5. The main script to enable the toast messages. Copy and insert the following snippets after the latest jQuery library. That's it.
$(".toast-trigger").click(function(e){ e.preventDefault(); datatoast = $(this).attr("data-toast"); if ( $( this ).hasClass( "toast-auto" ) && !$("#" + datatoast).is(":visible") ){ $("#" + datatoast).fadeIn(400).delay(2000).fadeOut(400); } else if ( !$("#" + datatoast).is(":visible") ){ $("#" + datatoast).fadeIn(400); }; }); $(".close-toast").click(function(e){ e.preventDefault(); closetoast = $(this).parent().attr("id"); $("#" + closetoast).fadeOut(400); });
This awesome jQuery plugin is developed by tobiasdev. For more Advanced Usages, please check the demo page or visit the official website.