Windows File Explorer Like Folder Tree In jQuery
File Size: | 33.1 KB |
---|---|
Views Total: | 9784 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
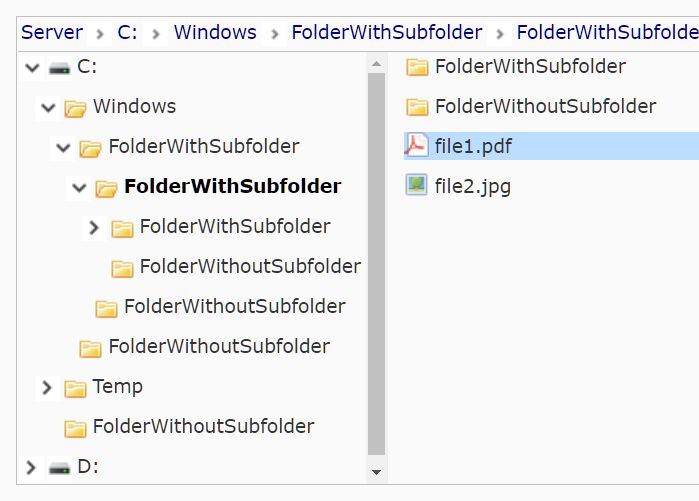
A jQuery plugin to generate a multi-level folder tree that represents the folder hierarchy of your file system and allows the user to browse folders and files in a Windows File Explorer like interface.
Features:
- Fetch file/folder information via AJAX.
- Custom connector scripts.
- Draggable/resizable column view.
See Also:
How to use it:
1. Load the needed jQuery and Split.js (for split view) in the document.
<script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/cdn/split.min.js"></script>
2. Create a container in which you want to generate the folder tree.
<div id="fileexplorer-example"></div>
3. Initialize the plugin and specify the path/label of the root.
$("#fileexplorer-example").jQueryFileExplorer({ root: "/", rootLabel:"Server", });
4. Specify the URL (or a function) that responds with folder/file information.
$("#fileexplorer-example").jQueryFileExplorer({ script: getPath }); $("#fileexplorer-example").jQueryFileExplorer({ script: "https://yoursite.com/FileExplorer/GetPath" });
5. The data structure should look as follow.
function getPath(data) { if(data.path=='/') { return [ { label: 'C:', path: 'C:', isDrive: true, isFolder: true, hasSubfolder: true, subitems:['Total: 300,000', 'Free: 100,000'] }, { label: 'D:', path: 'D:', isDrive: true, isFolder: true, hasSubfolder: true, subitems:['Total: 100,000', 'Free: 80,000'] } ]; } else if(data.path=='C:') { return [ { label: 'Windows', path: 'C:/Windows', hasSubfolder: true, isFolder: true, subitems:['1/2/2021'] }, { label: 'Temp', path: 'C:/Temp', hasSubfolder: true, isFolder: true, lastModified: '1/1/2021' }, { label: 'FolderWithoutSubfolder', path: 'C:/FolderWithoutSubfolder', hasSubfolder: false, isFolder: true, subitems: ['1/3/2021'] }, { label: 'File1', path: 'C:/File1.pdf', isFolder: false, ext: 'pdf', subitems: ['2/2/2021', '123,433'] } ]; } else if(data.path.endsWith("/FolderWithoutSubfolder")) { return [ { label: 'file1.pdf', path: data.path+"/file1.pdf", ext: 'pdf', isFolder: false, subitems: ['2/2/2021', '123,234'] }, { label: 'file2.jpg', path: data.path+"/file2.jpg", ext: 'jpg', isFolder: false, subitems: ['2/2/2021', '123,234'] } ]; } else { return [ { label: 'FolderWithSubfolder', path: data.path+"/FolderWithSubfolder", hasSubfolder: true, isFolder: true, subitems: ['1/1/2021'] }, { label: 'FolderWithoutSubfolder', path: data.path+"/FolderWithoutSubfolder", hasSubfolder: false, isFolder: true, subitems: ['1/1/2021'] }, { label: 'file1.pdf', path: data.path+"/file1.pdf", ext: 'pdf', isFolder: false, subitems: ['2/2/2021', '123,234'] }, { label: 'file2.jpg', path: data.path+"/file2.jpg", ext: 'jpg', isFolder: false, subitems: ['2/2/2021','123,234'] } ]; } }
6. Specify a function or a URL to handle the file when getting clicked.
$("#fileexplorer-example").jQueryFileExplorer({ fileScript: function(file){ window.open("https://yoursite.com/FileExplorer/GetPath?path="+file.path); } });
This awesome jQuery plugin is developed by edmlin. For more Advanced Usages, please check the demo page or visit the official website.