Customizable jQuery Modal Dialog Plugin with CSS3 Animations - jAlert
File Size: | 2.6 MB |
---|---|
Views Total: | 14868 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
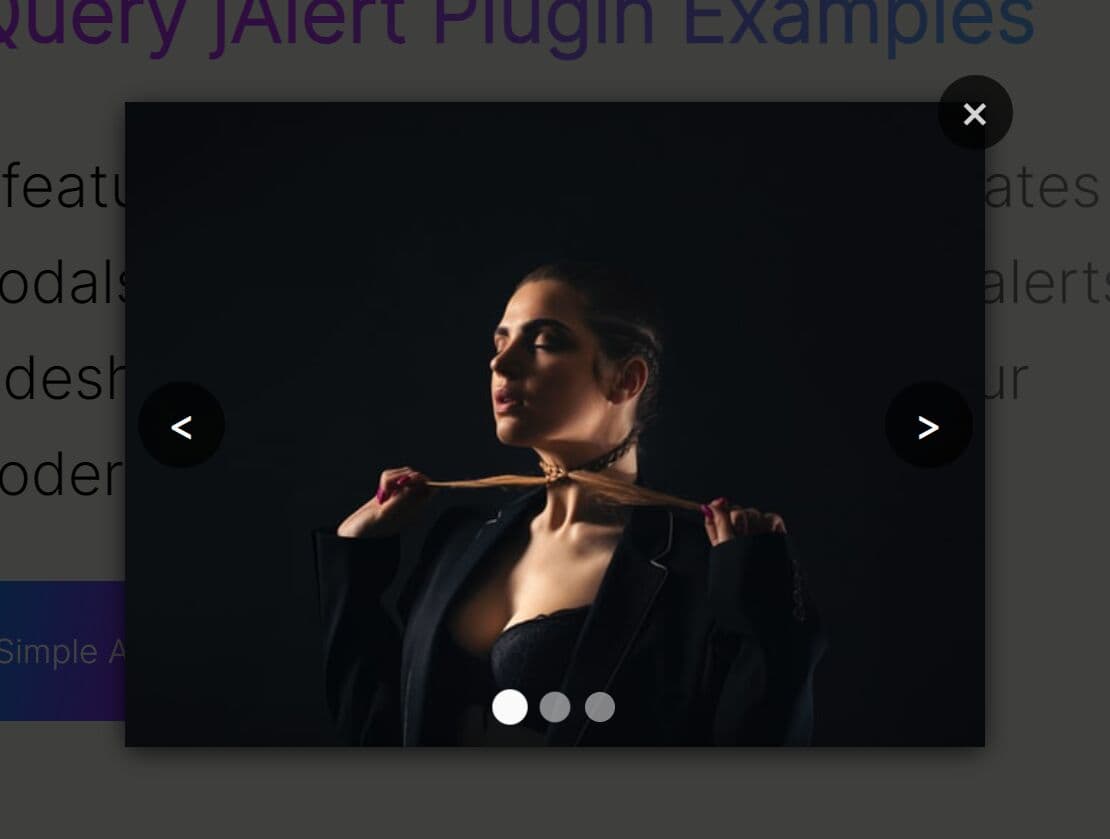
jAlert is a simple yet feature-rich jQuery plugin used to create fully configurable modals, dialogs and popups with cool CSS3 animations powered by animate.css.
Features:
- Responsive design.
- Replaces the default alert / prompt / confirm dialog boxes.
- 6 predefined sizes & themes.
- AJAX, images, iframes and videos supported.
Basic usage:
1. Load the required stylesheet jAlert.css
in the head section of the document.
<link href="src/jAlert.css" rel="stylesheet">
2. Load the animate.css for amazing CSS3 animations.
<link rel="stylesheet" href="//cdnjs.cloudflare.com/ajax/libs/animate.css/3.2.0/animate.min.css">
3. Load jQuery library and other required JS files at the bottom of the document.
<script src="//code.jquery.com/jquery.min.js"></script> <script src="src/jAlert.js"></script> <script src="src/jAlert-functions.js"></script>
4. Basic usage.
// default alert popup alert('Hello World!'); // success successAlert('Success', 'Hello World!'); // error errorAlert('Error', 'Hello World!'); // info infoAlert('Info', 'Hello World!'); // warning warningAlert('Warning', 'Hello World!'); // black warning blackAlert('Warning', 'Hello World!'); // image lightbox imageAlert('1.jpg'); // video lightbox videoAlert('http://youtube.com/...'); // ajax lightbox ajaxAlert('external.html'); // iframe lightbox iframeAlert('https://www.jqueryscript.net'); // confirm dialog confirm(function(){ console.log('confirmed!'); }, function(){ console.log('denied'); });
5. Default usage (Doesn't require jAlert-functions.js).
$.jAlert({ // options }); // or $('.btn').alertOnClick({ // triggered by a button });
6. Full options with default values.
//title for the popup (false = don't show) 'title': false, //html for the popup (replaced if you use image, ajax, or iframe) 'content': false, //remove padding from the body 'noPadContent': false, //make the jAlert completely fullscreen 'fullscreen': false, //adds a centered img tag 'image': false, //defaults to max-width: 100%; width: auto; 'imageWidth': 'auto', //adds a responsive iframe video - value is the "src" of the iframe 'video': false, //uses ajax call to get contents 'ajax': false, //callback for when ajax fails 'onAjaxFail': function(alert, errorThrown){ alert.closeAlert(); errorAlert(errorThrown); }, //uses iframe as content 'iframe': false, //string. height of the iframe within the popup (false = 90% of viewport height) 'iframeHeight': false, //adds a class to the jAlert (add as many as you want space delimited) 'class': '', //add classes to the jAlert (space delimited) 'classes': '', //adds an ID to the jAlert 'id': false, // see animate.css 'showAnimation': 'bounceIn', 'hideAnimation': 'bounceOut', // approx duration of animation to wait until onClose 'animationTimeout': 600, // red, green, blue, black, default 'theme': 'default', //white, black 'backgroundColor': 'black', //blurs background elements 'blurBackground': false, //false = css default, xsm, sm, md, lg, xlg, full, { height: 200, width: 200 } 'size': false, //if there's already an open jAlert, remove it first 'replaceOtherAlerts': false, //close the alert when you click anywhere 'closeOnClick': false, //close the alert when you click the escape key 'closeOnEsc': true, //adds a button to the top right of the alert that allows you to close it 'closeBtn': true, //alternative close button 'closeBtnAlt': false, //alternative round close button 'closeBtnRound': true, //alternative round close button (in white) 'closeBtnRoundWhite': false, //adds buttons to the popup at the bottom. Pass an object for a single button, or an object of objects for many 'btns': false, //By default we specify as false. You can add secound 'autoClose' : false, /* Variety of buttons you could create (also, an example of how to pass the object 'btns': [ {'text':'Open in new window', 'closeAlert':false, 'href': 'http://google.com', 'target':'_new'}, {'text':'Cool, close this alert', 'theme': 'blue', 'closeAlert':true}, {'text':'Buy Now', 'closeAlert':true, 'theme': 'green', 'onClick': function(){ console.log('You bought it!'); } }, {'text':'I do not want it', 'closeAlert': true, 'theme': 'red', 'onClick': function(){ console.log('Did not want it'); } }, {'text':'DOA', 'closeAlert': true, 'theme': 'black', 'onClick': function(){ console.log('Dead on arrival'); } } ] */ //adds optional background to btns 'btnBackground': true, //pass a selector to autofocus on it 'autofocus': false, //on open call back. Fires just after the alert has finished rendering 'onOpen': function(alert){ return false; }, //fires when you close the alert 'onClose': function(alert){ return false; }, //modal, confirm, tooltip 'type': 'modal', /* The following only applies when type == 'confirm' */ 'confirmQuestion': 'Are you sure?', 'confirmBtnText': 'Yes', 'denyBtnText': 'No', 'confirmAutofocus': '.confirmBtn', //confirmBtn or denyBtn 'onConfirm': function(e, btn){ e.preventDefault(); console.log('confirmed'); return false; }, 'onDeny': function(e, btn){ e.preventDefault(); //console.log('denied'); return false; }
Changelog:
2020-09-10
- Fix toggleFocusTrap() call locations
v4.9.1 (2019-07-26)
- Cleanup some CSS stuff, improve iframe full height, improve image fullscreen
v4.8.0 & v4.7.0 (2019-07-10)
- Added confirm question as the third param of the confirm() method
- Accepts dom element for content (it'll grab the html automatically)
- Button onClick method now receives 3 params e, btn, alert (so you can quickly alert.closeAlert())
- When you open an image alert using the image param, it will now shrink the popup to fit the image size if it's smaller and disables alert padding
v4.6.5 (2018-08-23)
- fix inability to scroll
2018-08-13
- Bugfix
2016-08-26
- add fullscreen: true/false + tiny adjustment to red alternative close button padding
2016-08-25
- fix dupe button callbacks, add dist folder, autocompile hook instructions, bump version to 4.5
2016-07-20
- added box-shadow back to improve look of white background
2016-05-12
- fix for height
2016-05-11
- v4
2016-05-07
- added dark colors and adjusted each shade
2016-05-06
- adjust round close button
2016-05-04
- added new round button as option, remove borders
2015-11-03
- Fixed or IE8
2015-09-13
- fixed alert variable overriding function.
2015-09-11
- fixed alert variable overriding function.
2015-09-10
- fix for alert.each
2015-09-04
- enhancement: remove focus from the element that initiated the alert
- options were being overriden by each new alert
2015-09-01
- Compatibility of Date.now() for IE8 and 7, opacity of backgrounds in IE8 and 7
2015-07-15
- fix for ie10 iframe
2015-07-13
- Changed default animation to fade.
2015-07-09
- .off fix
2015-07-03
- Fixed on scrolling tall alert, background wasn't position: fixed
2015-06-29
- Fixed body overflow hidden bug
This awesome jQuery plugin is developed by HTMLGuyLLC. For more Advanced Usages, please check the demo page or visit the official website.