3D Interactive Fullscreen Navigation With jQuery And CSS3
File Size: | 3.06 KB |
---|---|
Views Total: | 3231 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
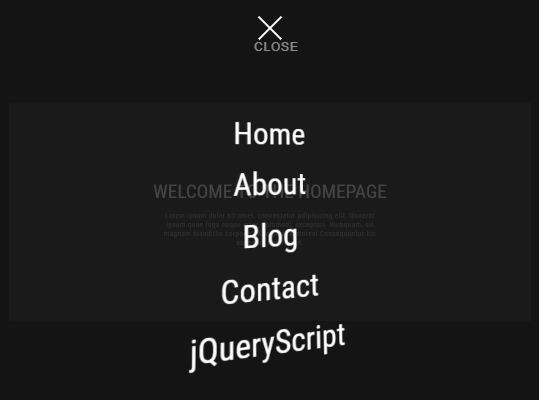
A 3D interactive fullscreen hamburger navigation concept that automatically rotates & flips menu on mouse move using CSS3 3D transforms. Clicking on the menu links will automatically switch between page sections without refreshing the current page.
How to use it:
1. Create the navigation menu.
<div class="wrapper"> <nav role="navigation"> <div class="elem" style="transform: perspective(1000px) rotateY(0deg) rotateX(0deg);"> <ul> <li name="home"><a href="#">Home</a></li> <li name="about"><a href="#">About</a></li> <li name="blog"><a href="#">Blog</a></li> <li name="contact"><a href="#">Contact</a></li> <li name="contact"><a href="#">jQueryScript</a></li> </ul> </div> </nav> </div>
2. Create a button to toggle the fullscreen navigation.
<button class="menu"> <span></span> </button>
3. Create your own page sections you want to switch between.
<div class="section-wrap"> <section class="page home active"> <div class="vertical-align"> <div class="wrap"> <h2>Home Page</h2> </div> </div> </section> <section class="page about"> <div class="vertical-align"> <div class="wrap"> <h2>About Page</h2> </div> </div> </section> ... </div>
4. The main CSS styles for the fullscreen navigation.
nav { position: fixed; width: 100%; top: 0; left: 0; right: 0; margin: 0 auto; width: 100%; text-align: center; height: 100vh; z-index: 2; display: none; perspective: 1000px; transform-style: preserve-3d; -webkit-transform-style: preserve-3d; cursor: pointer; } nav ul { position: absolute; top: 0; right: 0; left: 0; margin: auto; width: 300px; height: 360px; transform: rotateX(180deg) scale(0); -webkit-transform: rotateX(180deg) scale(0); transform-origin: top; opacity: 0; transform-style: preserve-3d; -webkit-transform-style: preserve-3d; transition: 1s all ease-in-out 0s; -webkit-transition: 1s all ease-in-out 0s; } nav ul.active { transform: rotateX(0deg) scale(1.6); -webkit-transform: rotateX(0deg) scale(1.6); opacity: 1; transform-origin: top; transform-style: preserve-3d; -webkit-transform-style: preserve-3d; transition: 1s all ease-in-out 0.5s; -webkit-transition: 1s all ease-in-out 0.5s; } nav ul li { margin: 20px 0; } nav ul li a { color: #fff; font-size: 48px; } .menu { display: block; position: absolute; top: 30px; left: 0; right: 0; margin: 0 auto; height: 25px; width: 40px; background: transparent; border: none; outline: none; cursor: pointer; z-index: 3; } .menu:before { content: "MENU"; position: absolute; bottom: -20px; left: 0; right: 0; margin: 0 auto; color: rgba(255,255,255,0.5); font-size: 10px; letter-spacing: 1px; } .menu.active:before { content: "CLOSE"; position: absolute; bottom: -20px; left: 0; right: 0; margin: 0 auto; color: rgba(255,255,255,0.5); font-size: 10px; letter-spacing: 1px; } .menu span { height: 2px; background: #fff; display: block; width: 100%; position: absolute; top: 0; bottom: 0; margin: auto; transition: 0.2s all ease-in-out; -webkit-transition: 0.2s all ease-in-out; } .menu span:after, .menu span:before { content: ""; height: 2px; background: #fff; display: block; width: 100%; position: absolute; transition: 0.2s top ease-in-out 0.2s, transform 0.2s ease-in-out; -webkit-transition: 0.2s top ease-in-out 0.2s, transform 0.2s ease-in-out; } .menu span:after { top: -10px; } .menu span:before { top: 10px; } .menu.active span { background: transparent; } .menu.active span:before, .menu.active span:after { transition: 0.2s top ease-in-out, transform 0.2s ease-in-out 0.2s; -webkit-transition: 0.2s top ease-in-out, transform 0.2s ease-in-out 0.2s; } .menu.active span:after { top: 0; transform: rotate(45deg); } .menu.active span:before { top: 0; transform: rotate(-45deg); }
5. The other required CSS styles.
.wrapper { position: absolute; top: 0; bottom: 0; left: 0; right: 0; margin: auto; transition: 0.8s transform ease-in-out; -webkit-transition: 0.8s transform ease-in-out; transform: scale(0.5); -webkit-transform: scale(0.5); z-index: 2; } .page { height: 100vh; width: 100vw; background: #323232; opacity: 0; position: absolute; top: 0; left: 0; right: 0; bottom: 0; margin: auto; } .page.active { opacity: 1; } .vertical-align { position: relative; height: 160px; top: 50%; transform: translateY(-50%); transition: 0.2s all ease-in-out; -webkit-transition: 0.2s all ease-in-out; } .wrap { width: 60%; margin: 0 auto; text-align: center; transition: 0.2s all ease-in-out; -webkit-transition: 0.2s all ease-in-out; } .page .wrap p { font-size: 18px; margin: 20px auto; width: 70%; opacity: .5; letter-spacing: 1px; } .section-wrap { height: 100vh; width: 100vw; position: relative; transform-style: preserve-3d; perspective: 1000px; transition: 0.8s all ease-in-out; -webkit-transition: 0.8s all ease-in-out; overflow: hidden; opacity: 1; } .section-wrap.active { opacity: 0.2; transform: scale(0.5); -webkit-transform: scale(0.5); } section.active { transform: rotateX(0deg); }
6. Insert the necessary jQuery plugin into the document.
<script src="https://code.jquery.com/jquery-3.2.1.min.js"></script>
7. The main JavaScript to activate the fullscreen navigation.
$('.menu, nav').click(function(){ if ($('.menu').hasClass('active')) { $('nav ul').removeClass('active'); $('nav').delay(800).fadeOut('slow'); setTimeout(function() { $('.menu').removeClass('active'); $('.section-wrap').removeClass('active'); }, 800); } else { $('.menu').addClass('active'); $('nav').fadeIn('slow'); $('nav').addClass('active'); $('nav ul').addClass('active'); $('.section-wrap').addClass('active'); } });
8. Add a 3D interactive effect to the navigation.
!(function ($doc, $win) { var screenWidth = $win.screen.width / 2, screenHeight = $win.screen.height / 2, $elems = $doc.getElementsByClassName("elem"), validPropertyPrefix = '', otherProperty = 'perspective(1000px)', elemStyle = $elems[0].style; if(typeof elemStyle.webkitTransform == 'string') { validPropertyPrefix = 'webkitTransform'; } else if (typeof elemStyle.MozTransform == 'string') { validPropertyPrefix = 'MozTransform'; } $doc.addEventListener('mousemove', function (e) { var centroX = e.clientX - screenWidth, centroY = screenHeight - (e.clientY + 13), degX = centroX * 0.04, degY = centroY * 0.01, $elem for (var i = 0; i < $elems.length; i++) { $elem = $elems[i]; $elem.style[validPropertyPrefix] = otherProperty + 'rotateY('+ degX +'deg) rotateX('+ degY +'deg)'; }; }); })(document, window);
9. Enable the nav links to switch between page section when clicked.
$('nav ul li').on('click', function(){ var elementName = $(this).attr('name'); $('.page.active').fadeOut(800).removeClass('active'); $('.page.'+elementName).delay(1000).fadeIn(1000).addClass('active'); });
This awesome jQuery plugin is developed by Thomas Podgrodzki. For more Advanced Usages, please check the demo page or visit the official website.