Cool Touch-enabled Corner Navigation with jQuery and CSS3
File Size: | 9.6 KB |
---|---|
Views Total: | 2791 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
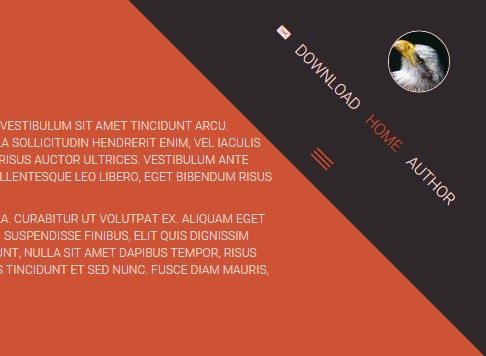
A cool touch-enabled navigation concept to create a corner menu that slides out with a subtle transition effect, depended on jQuery, touchSwipe, CSS3 transitions & transforms.
How to use it:
1. Load the Ionicons for navigation icons.
<link href="//cdnjs.cloudflare.com/ajax/libs/ionicons/1.5.2/css/ionicons.min.css" rel="stylesheet">
2. Create a corner menu.
<nav id="corner_toggle" class="top-left"> <div id="corner_toggle_container"> ... </div> </nav>
3. Style the corner menu and place it in the upper left corner of the page.
nav#corner_toggle { -webkit-transition: all 0.15s ease-in-out; -moz-transition: all 0.15s ease-in-out; -ms-transition: all 0.15s ease-in-out; -o-transition: all 0.15s ease-in-out; z-index: 1000; position: fixed; background-color: #2e282a; height: 500px; width: 2000px; top: -375px; } nav#corner_toggle.top-left { left: -1125px; -webkit-transform: rotate(-45deg); -ms-transform: rotate(-45deg); transform: rotate(-45deg); } #corner_toggle_container { width: 100%; height: 100%; } .corner_toggle_ctrl { margin: 0 auto; text-align: center; position: fixed; width: 100%; color: #cd5334; font-size: 30px; } .corner_toggle_ctrl:hover { color: #cd5334; } .corner_toggle_ctrl.top { bottom: 0; padding-bottom: 10px; } .corner_toggle_ctrl i { cursor: pointer; }
4. Load the latest version of jQuery library.
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.11.2/jquery.min.js"></script>
5. Load the jQuery touchSwipe plugin to enable touch swipe support.
<script src="//cdnjs.cloudflare.com/ajax/libs/jquery.touchswipe/1.6.4/jquery.touchSwipe.js"></script>
6. The core JavaScript to enable the corner menu.
$(document).ready(function () { var corner_toggle = $('#corner_toggle'), corner_toggle_container = $('#corner_toggle #corner_toggle_container'), corner_class = corner_toggle.attr('class'), vert_pos = 'top', horiz_pos, init_vert = '-375', init_horiz = '-1125', increased_vert = parseInt(init_vert) * 0.5, increased_horiz = parseInt(init_horiz) * 0.83; switch (corner_class) { case 'top-left': horiz_pos = 'left'; break; case 'top-right': horiz_pos = 'right'; break; } $('button#top_left_btn').click(function () { if (corner_toggle.hasClass('top-left')) { return false; } else { corner_toggle.fadeOut('fast', function () { $(this).delay(250).fadeIn('fast'); corner_toggle.css('top', ''); corner_toggle.css('right', ''); corner_toggle.removeClass('top-right'); corner_toggle.addClass('top-left'); horiz_pos = 'left'; }); } }); corner_toggle_container.click(function () { if (corner_toggle.hasClass('active')) { toggleCornerMenu('in'); } else { toggleCornerMenu('out'); } }); corner_toggle.swipe({ tap: function (event, target) { if ($(this).hasClass('active')) { toggleCornerMenu('in'); } else { toggleCornerMenu('out'); } }, swipe: function (event, direction, distance, duration, fingerCount) { switch (corner_class) { case 'top-left': if (direction == 'down' || direction == 'right' && !$(this).hasClass('active')) { toggleCornerMenu('out'); } if (direction == 'up' || direction == 'left' && $(this).hasClass('active')) { toggleCornerMenu('in'); } break; case 'top-right': if (direction == 'down' || direction == 'left' && !$(this).hasClass('active')) { toggleCornerMenu('out'); } if (direction == 'up' || direction == 'right' && $(this).hasClass('active')) { toggleCornerMenu('in'); } break; } }, threshold: 0 }); var toggleCornerMenu = function (action) { switch (action) { case 'in': corner_toggle.css(vert_pos, init_vert + 'px'); corner_toggle.css(horiz_pos, init_horiz + 'px'); corner_toggle.toggleClass('active'); break; case 'out': corner_toggle.css(vert_pos, increased_vert + 'px'); corner_toggle.css(horiz_pos, increased_horiz + 'px'); corner_toggle.toggleClass('active'); break; } }; });
This awesome jQuery plugin is developed by jamesgray. For more Advanced Usages, please check the demo page or visit the official website.