Dynamic Right Click Menu Plugin With jQuery - Context Menu
File Size: | 20.3 KB |
---|---|
Views Total: | 4927 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
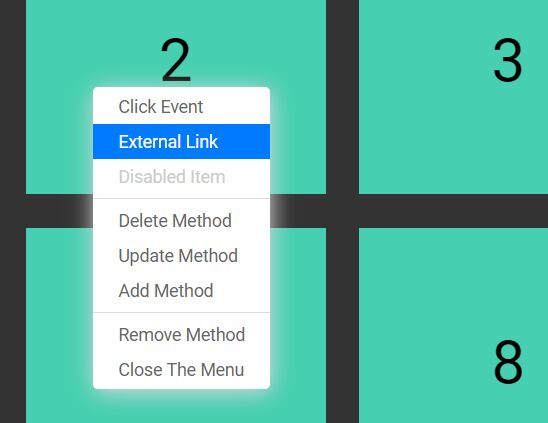
A lightweight and cross-browser jQuery plugin to dynamically render a custom, clean context menu triggered by right click.
The context menu can be attached to the whole document or a DOM element you specify. You can also specify the function (eg. click event) for each menu item.
The plugin also supports dynamic menu creation, deleting, and updating.
How to use it:
1. Add jQuery library and the context menu plugin's files to the html page.
<link rel="stylesheet" href="dist/contextmenu.css"> <script src="https://code.jquery.com/jquery-1.12.4.min.js" integrity="sha384-nvAa0+6Qg9clwYCGGPpDQLVpLNn0fRaROjHqs13t4Ggj3Ez50XnGQqc/r8MhnRDZ" crossorigin="anonymous"> </script> <script src="dist/contextmenu.js"></script>
2. Create an array of item objects as follows:
const myMenu = [{ "name":"Item 1", "func":"customFunction()", "link":null, "disable":false }, { "name":"Item 2", "link":"https://www.jqueryscript.net", "disable":false }, { "name":"Disabled", "disable":true }, { "name":"-" // separator }, ... ]
3. Initialize the plugin and attach the context menu to a specific element.
const menu= new Contextmenu({ // menu id name:"menu", // container element // default: 'body' wrapper:".wrapper", // trigger element // default: 'body' trigger: ".item", item: myMenu });
4. Decide whether to open the links in a new window.
const menu= new Contextmenu({ target : "_blank" });
5. Execute a function before opening the context menu.
const menu= new Contextmenu({ beforeFunc: function () { // do something } });
6. Add a new item to the context menu.
const menu= new Contextmenu(); menu.add({ index: 3, name: "Item Name", func: "", link: null, disable: false });
7. Remove a specific item from the context menu.
menu.del(3);
8. Update the options of a specific menu item.
menu.update({ index: 3, name: "New Name", func: "", link: null, disable: false });
9. Destroy the plugin and remove the context menu from DOM.
menu.destroy();
This awesome jQuery plugin is developed by shulkme. For more Advanced Usages, please check the demo page or visit the official website.