One Page Scrolling Navigation with jQuery and CSS3
File Size: | 2.67 KB |
---|---|
Views Total: | 6978 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
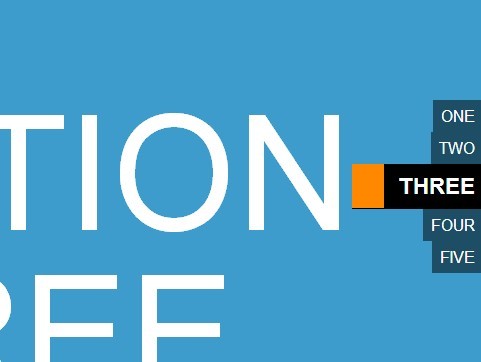
Scroll Nav is a jQuery plugin designed for one page scrolling website that generates a side navigation to indicate which page section you stay in. Also provides a scroll to functionality which allows you to scroll through different sections of your website.
How to use it:
1. Create a simple one page scrolling layout as follows.
<section id="section-one" class="section scroll-item" title="One"> <div class="inner"> <h2>Section One</h2> </div> </section> <section id="section-two" class="section scroll-item" title="Two"> <div class="inner"> <h2>Section Two</h2> </div> </section> <section id="section-three" class="section scroll-item" title="Three"> <div class="inner"> <h2>Section Three</h2> </div> </section> <section id="section-four" class="section scroll-item" title="Four"> <div class="inner"> <h2>Section Four</h2> </div> </section> <section id="section-five" class="section scroll-item" title="Five"> <div class="inner"> <h2>Section Five</h2> </div> </section>
2. The CSS styles for the one page scrolling laytout.
html, body { margin: 0; padding: 0; min-height: 100%; vertical-align: baseline; } .inner { width: 62.5%; margin: 0 auto; padding-top: 20%; } .section { -webkit-box-sizing: border-box; -moz-box-sizing: border-box; box-sizing: border-box; height: 400px; } #section-one { background: #73d2e5; } #section-two { background: #57b8d9; } #section-three { background: #3d9ccc; } #section-four { background: #2680bf; } #section-five { background: #1262b2; }
3. Create a side navigation for the one page scrolling layout.
<nav id="section-menu"> <ul> </ul> </nav>
4. The CSS for the side navigation.
#section-menu { position: fixed; top: 50%; right: 0; z-index: 3000; text-transform: uppercase; -webkit-transition: all ease 0.1s; transition: all ease 0.1s; } #section-menu.freeze { right: -112px; } .touch #section-menu { display: none; } #section-menu ul { margin: 0; padding: 0; list-style: none; } #section-menu li { } #section-menu a { float: right; clear: both; display: block; height: 2em; line-height: 2em; text-decoration: none; padding: 0 8px; background: rgba(0,0,0,0.5); color: #fff; white-space: nowrap; -webkit-transition: all ease 0.1s; transition: all ease 0.1s; } #section-menu.freeze a { float: none; width: 128px; } #section-menu a:hover, #section-menu a:focus { background: rgba(0,0,0,0.75); font-size: 1.2em; } #section-menu a span { display: none; font-weight: 700; position: relative; width: 32px; left: -8px; text-align: center; background: rgba(0,0,0,0.1); } #section-menu a.active { background: #000; font-size: 1.4em; font-weight: 700; } #section-menu.freeze a span { display: inline-block; } #section-menu a.active span { display: inline-block; background: #000; } #section-menu a.active span:before { content: ''; position: absolute; width: 100%; height: 100%; left: 0; background: #f80; } #section-menu.freeze a.active span:before { display: none; }
5. Include the latest version of jQuery library in the web page.
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
6. The jQuery script to enable the side navigation.
jQuery(function($) { var html = $('html'); var viewport = $(window); var viewportHeight = viewport.height(); var scrollMenu = $('#section-menu'); var timeout = null; function menuFreeze() { if (timeout !== null) { scrollMenu.removeClass('freeze'); clearTimeout(timeout); } timeout = setTimeout(function() { scrollMenu.addClass('freeze'); }, 2000); } scrollMenu.mouseover(menuFreeze); /* ========================================================================== Build the Scroll Menu based on Sections .scroll-item ========================================================================== */ var sectionsHeight = {}, viewportheight, i = 0; var scrollItem = $('.scroll-item'); var bannerHeight; function sectionListen() { viewportHeight = viewport.height(); bannerHeight = (viewportHeight); $('.section').addClass('resize').css('height', bannerHeight); scrollItem.each(function(){ sectionsHeight[this.title] = $(this).offset().top; }); } sectionListen(); viewport.resize(sectionListen); viewport.bind('orientationchange', function() { sectionListen(); }); var count = 0; scrollItem.each(function(){ var anchor = $(this).attr('id'); var title = $(this).attr('title'); count ++; $('#section-menu ul').append('<li><a id="nav_' + title + '" href="#' + anchor + '"><span>' + count + '</span> ' + title + '</a></li>'); }); function menuListen() { var pos = $(this).scrollTop(); pos = pos + viewportHeight * 0.625; for(i in sectionsHeight){ if(sectionsHeight[i] < pos) { $('#section-menu a').removeClass('active'); $('#section-menu a#nav_' + i).addClass('active');; var newHash = '#' + $('.scroll-item[title="' + i + '"]').attr('id'); if(history.pushState) { history.pushState(null, null, newHash); } else { location.hash = newHash; } } else { $('#section-menu a#nav_' + i).removeClass('active'); if (pos < viewportHeight - 72) { history.pushState(null, null, ' '); } } } } scrollMenu.css('margin-top', scrollMenu.height() / 2 * -1); /* ========================================================================== Smooth Scroll for Anchor Links and URL refresh ========================================================================== */ scrollMenu.find('a').click(function() { var href = $.attr(this, 'href'); $('html').animate({ scrollTop: $(href).offset().top }, 500, function() { window.location.hash = href; }); return false; }); /* ========================================================================== Fire functions on Scroll Event ========================================================================== */ function scrollHandler() { menuListen(); menuFreeze(); } scrollHandler(); viewport.on('scroll', function() { scrollHandler(); //window.requestAnimationFrame(scrollHandler); }); });
This awesome jQuery plugin is developed by zutrinken. For more Advanced Usages, please check the demo page or visit the official website.