Responsive Off-Canvas Slide Menu with jQuery and CSS3
File Size: | 3.58 KB |
---|---|
Views Total: | 4222 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
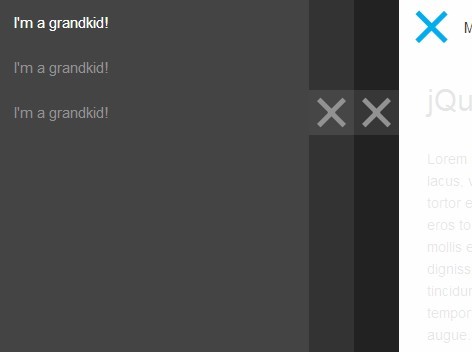
Yet another jQuery & CSS3 responsive off-canvas sliding menu system that supports 3-tier sub-menus based on nested Html lists. As you click the menu button, a vertical sidebar menu slides out from the left side of your browser window with an overlay covering your main content.
How to use it:
1. Create a toggle button to open the off-canvas menu.
<div id="menu-toggle"> <div id="menu-icon"> <span></span> <span></span> <span></span> <span></span> <span></span> <span></span> </div> Menu </div>
2. Create an overlay for the main content that will focus your visitors on the off-canvas menu.
<div id="menu-overlay"></div>
3. Create the Html for a multi-level off-canvas menu using nested Html unordered lists.
<ul id="menu"> <li><a href="#">Visit My Site</a></li> <li><a href="#">No children...</a></li> <li><a href="#">Hey - this one has some kids!</a> <ul> <li><a href="#">I'm a kid!</a></li> <li><a href="#">I'm a kid!</a></li> <li><a href="#">Got kids of my own...</a> <ul> <li><a href="#">I'm a grandkid!</a></li> <li><a href="#">I'm a grandkid!</a></li> <li><a href="#">I'm a grandkid!</a></li> </ul> </li> </ul> </li> </ul>
4. Wrap the main content in a container with unique ID of 'page-content'
<div id="page-content"> Your main content goes here </div>
5. The CSS/CSS3 rules to style the off-canvas menu.
#menu-toggle { position: fixed; padding: 20px 20px 20px 65px; width: 100%; left: 0; top: 0; background: rgba(255,255,255,.9); color: #444444; z-index: 5; } #menu-toggle.open { left: 400px; } #menu-icon { width: 45px; height: 35px; position: absolute; left: 10px; top: 10px; -webkit-transform: rotate(0deg); -moz-transform: rotate(0deg); -o-transform: rotate(0deg); transform: rotate(0deg); -webkit-transition: .5s ease-in-out; -moz-transition: .5s ease-in-out; -o-transition: .5s ease-in-out; transition: .5s ease-in-out; cursor: pointer; } #menu-icon span { display: block; position: absolute; height: 5px; width: 50%; background: #00aeef; opacity: 1; -webkit-transform: rotate(0deg); -moz-transform: rotate(0deg); -o-transform: rotate(0deg); transform: rotate(0deg); -webkit-transition: .25s ease-in-out; -moz-transition: .25s ease-in-out; -o-transition: .25s ease-in-out; transition: .25s ease-in-out; } #menu-icon span:nth-child(even) { left: 50%; } #menu-icon span:nth-child(odd) { left: 0px; } #menu-icon span:nth-child(1), #menu-icon span:nth-child(2) { top: 5px; } #menu-icon span:nth-child(3), #menu-icon span:nth-child(4) { top: 15px; } #menu-icon span:nth-child(5), #menu-icon span:nth-child(6) { top: 25px; } #menu-icon.open span:nth-child(1), #menu-icon.open span:nth-child(6) { -webkit-transform: rotate(45deg); -moz-transform: rotate(45deg); -o-transform: rotate(45deg); transform: rotate(45deg); } #menu-icon.open span:nth-child(2), #menu-icon.open span:nth-child(5) { -webkit-transform: rotate(-45deg); -moz-transform: rotate(-45deg); -o-transform: rotate(-45deg); transform: rotate(-45deg); } #menu-icon.open span:nth-child(1) { left: 5px; top: 8px; } #menu-icon.open span:nth-child(2) { left: calc(50% - 5px); top: 8px; } #menu-icon.open span:nth-child(3) { left: -50%; opacity: 0; } #menu-icon.open span:nth-child(4) { left: 100%; opacity: 0; } #menu-icon.open span:nth-child(5) { left: 5px; top: 21px; } #menu-icon.open span:nth-child(6) { left: calc(50% - 5px); top: 21px; } /* MENU STYLES */ ul#menu, #menu ul { list-style-type: none; padding: 0; margin: 0; } #menu { background: #222222; width: 400px; position: fixed; top: 0; bottom: 0; left: -400px; z-index: 4; } #menu li { position: relative; } #menu.open { left: 0; } #menu a { display: block; padding: 15px; text-decoration: none; color: #929292; } #menu a:hover { color: white; } #menu > .parent > ul { position: fixed; top: 0; bottom: 0; left: -100%; width: 355px; } #menu > .parent > ul > .parent > ul { position: fixed; top: 0; bottom: 0; left: -100%; width: 310px; } #menu > .parent.open > ul { left: 0; background: #333333; } #menu > .parent.open > ul > .parent.open > ul { left: 0; background: #444444; } .submenu-toggle { position: absolute; right: 0px; top: 0px; cursor: pointer; width: 45px; height: 45px; text-indent: -5000px; overflow: hidden; } .submenu-toggle.open { background: rgba(255,255,255,.1); height: 100%; z-index: 5000; top: 0; } .submenu-toggle:before, .submenu-toggle:after { -webkit-transition: .5s ease-in-out; } .submenu-toggle:before { content: ''; display: block; position: absolute; height: 16px; width: 16px; top: 12px; right: 15px; border-top: 5px solid #929292; border-right: 5px solid #929292; -webkit-transform: rotate(45deg); } .submenu-toggle:after { content: ''; display: block; position: absolute; height: 16px; width: 16px; top: 12px; left: 50px; border-top: 5px solid #929292; border-right: 5px solid #929292; -webkit-transform: rotate(-135deg); } .submenu-toggle:hover:before, .submenu-toggle:hover:after { border-color: white; } .submenu-toggle.open:before { right: 23px; } .submenu-toggle.open:after { left: 23px; } #menu-overlay { position: fixed; width: 100%; background: red; top: 55px; bottom: 0; left: 0; z-index: 0; background: rgba(255,255,255,.0); } #menu-overlay.open { z-index: 3; background: rgba(255,255,255,.9); }
6. Include the latest version of jQuery javascript library at the bottom of your page.
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.11.0/jquery.min.js"></script>
7. The jQuery script to enable the off-canvas menu.
$(document).ready(function(){ // NAVIGATION MENU // menu icon states, opening main nav $('#menu-icon').click(function(){ $(this).toggleClass('open'); $('#menu,#menu-toggle,#page-content,#menu-overlay').toggleClass('open'); $('#menu li,.submenu-toggle').removeClass('open'); }); // clicking on overlay closes menu $('#menu-overlay').click(function(){ $('*').removeClass('open'); }); // add child menu toggles and parent class $('#menu li').has('ul').addClass('parent').prepend('<div class="submenu-toggle">open</div>'); // toggle child menus $('.submenu-toggle').click(function(){ var currentToggle=$(this); currentToggle.parent().toggleClass('open'); currentToggle.toggleClass('open'); }); });
This awesome jQuery plugin is developed by designcouch. For more Advanced Usages, please check the demo page or visit the official website.