Animated Sticky Navbar With jQuery And CSS3
File Size: | 2.97 KB |
---|---|
Views Total: | 6858 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
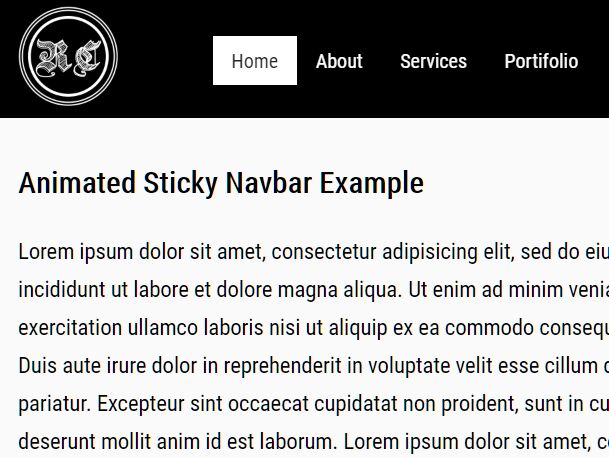
A modern site navigation that collapses a fullscreen hero header into a sticky navigation bar on scroll bar.
Built with JavaScript (jQuery) and CSS/CSS3. Ideal for landing pages or single page web applications.
See It In Action:
How to use it:
1. Add the sticky navigation bar into your hero header as follows:
<header> <img src="bg.jpg" class="banner"> <a href="#" class="logo"> <img src="logo.png"/> </a> <nav> <ul> <li> <a href="#" class="active">Home</a> </li> <li> <a href="#">About</a> </li> <li> <a href="#">Services</a> </li> <li> <a href="#">Portifolio</a> </li> <li> <a href="#">Team</a> </li> <li> <a href="#">Contact Us</a> </li> </ul> </nav> </header>
2. The CSS styles for the hero header.
header{ position: fixed; top: 0; left: 0; height: 100vh; width: 100%; background: #000; transition: 1s; padding: 0 100px; box-sizing: border-box; } header.scrolled{ height: 100px; } header img.banner{ object-fit: cover; position: absolute; top: 0; left: 0; height: 100%; width: 100%; transition: 1s; } header.scrolled img.banner{ opacity: 0; } header .logo{ position: absolute; top: calc(50% - 150px); left: calc(50% - 150px); transition: 1s; } header.scrolled .logo{ position: relative; top: 10px; left: 0; } header .logo img { width: 300px; transition: 1s; } header.scrolled .logo img { width: 80px; }
3. The CSS styles for sticky header navbar.
nav{ position: relative; float: right; } nav ul{ margin: 0; padding: 40px 0; display: flex; transition: 1s; opacity: 0; visibility: hidden; transform: translateX(100px); } header.scrolled nav ul{ opacity: 1; visibility: visible; transform: translateX(0); } nav ul li{ list-style: none; } nav ul li a { color: #fff; padding: 10px 15px; text-decoration: none; transition: 1s; } nav ul li a.active{ background: #fff; color:#262626; } nav ul li a:hover{ border-bottom: 2px solid white; }
4. The core JavaScript (jQuery script) to switch between CSS classes on page scroll. Copy and insert the following snippets after you load the latest jQuery library. That's it.
<script src="/path/to/cdn/jquery.slim.min.js"></script>
$(window).scroll(function(){ var scroll = $(window).scrollTop(); if (scroll > 0){ $("header").addClass('scrolled'); } else{ $("header").removeClass('scrolled'); } })
This awesome jQuery plugin is developed by rodcordeiro. For more Advanced Usages, please check the demo page or visit the official website.