Convert Bootstrap Navbar Into Off-canvas Navigation
File Size: | 2.95 KB |
---|---|
Views Total: | 1828 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
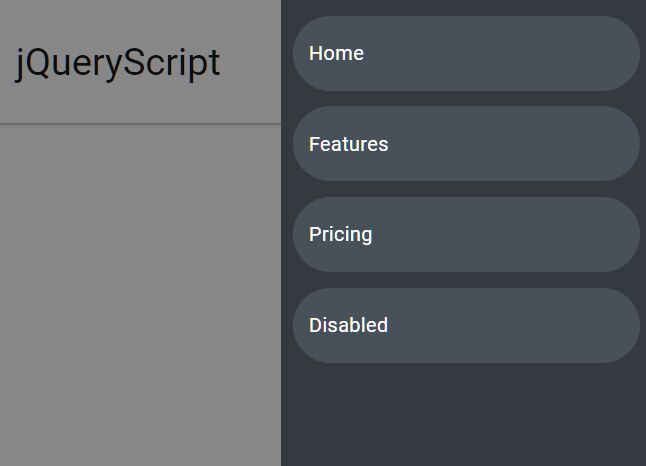
The native Responsive Behaviors of Bootstrap navbar component is boring.
This is a jQuery & CSS alternative that collapses the Bootstrap 4 navbar component into a mobile-friendly sidebar drawer (also called off-canvas navigation) when the screen size reaches a breakpoint defined in the media queries.
How to use it:
1. Add a fullcreen overlay to the Bootstrap navbar as follows:
<nav class="navbar navbar-expand-lg navbar-light bg-light"> <a class="navbar-brand" href="#">Navbar</a> <button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNavAltMarkup" aria-controls="navbarNavAltMarkup" aria-expanded="false" aria-label="Toggle navigation"> <span class="navbar-toggler-icon"></span> </button> <span class="collapse-bg"></span> <div class="collapse navbar-collapse" id="navbarNavAltMarkup"> <ul class="navbar-nav"> <li class="nav-item active"> <a class="nav-link" href="#">Home <span class="sr-only">(current)</span></a> </li> <li class="nav-item"> <a class="nav-link" href="#">Features</a> </li> <li class="nav-item"> <a class="nav-link" href="#">Pricing</a> </li> <li class="nav-item"> <a class="nav-link disabled" href="#" tabindex="-1" aria-disabled="true">Disabled</a> </li> </ul> </div> </nav>
.collapse-bg { position: fixed; display: none; }
2. Convert the collapsed navbar into an off-canvas navigation when the screen size is smaller than 992px.
@media (max-width: 992px) { .navbar-collapse { background: #343a40; position: fixed; right: -250px; top: 0; height: 100vh; width: 235px; z-index: 999; margin: 0 !important; -webkit-transition: right 0.3s linear 0s; -moz-transition: right 0.3s linear 0s; -ms-transition: right 0.3s linear 0s; -o-transition: right 0.3s linear 0s; transition: right 0.3s linear 0s; } .navbar-collapse a { color: #fff !important; } .navbar-collapse.show { right: 0; top: 0; -webkit-transition: right 0.3s linear 0s; -moz-transition: right 0.3s linear 0s; -ms-transition: right 0.3s linear 0s; -o-transition: right 0.3s linear 0s; transition: right 0.3s linear 0s; } .collapse-bg { display: none; position: fixed; background: #00000078; width: 100%; height: 200%; z-index: 888; right: 0; top: 0; -webkit-transition: right 0.3s linear 0s; -moz-transition: right 0.3s linear 0s; -ms-transition: right 0.3s linear 0s; -o-transition: right 0.3s linear 0s; transition: right 0.3s linear 0s; } .nav-item { background: #48515a; border-radius: 1.5rem; padding: 0 10px; margin: 10px 5px 0px 5px; } .navbar-nav { padding-left: 3px !important; } }
3. The main JavaScript to show the background overlay when the off-canvas navigation is toggled.
$(".navbar-toggler").click(function () { if ($(".navbar-toggler").hasClass("collapsed")) { $(".collapse-bg").css({ display: 'none' }); } else { $(".collapse-bg").css({ display: 'block' }); } }); // click the overlay to hide the drawer $(".collapse-bg").click(function () { $(".navbar-toggler").removeClass("collapsed"); $(".navbar-collapse").removeClass("show"); $(".collapse-bg").css({ display: 'none' }); });
This awesome jQuery plugin is developed by DabbaghDev. For more Advanced Usages, please check the demo page or visit the official website.