Auto Add Table Of Contents To Long Document Using jQuery
File Size: | 3.65 KB |
---|---|
Views Total: | 2207 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
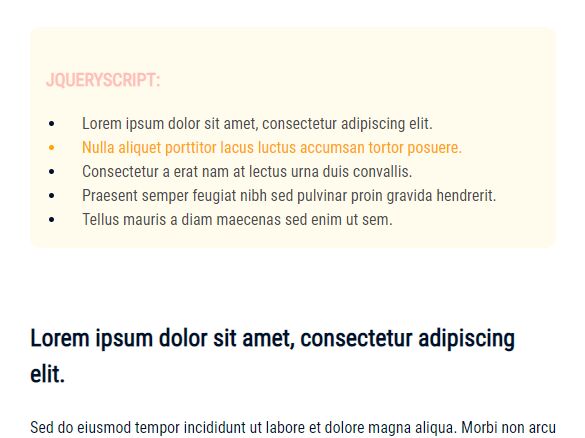
A simple and small jQuery script that dynamically generates a table of contents to easily and quickly navigate between sections within the long document.
The script automatically generates a list based navigation that exacts the headings and builds anchors & IDs from a given heading element.
Clicking the anchor links will smoothly scroll through the page sections using the jQuery animate()
method.
How to use it:
1. Split your long post/article to several sections using a heading element.
<h3>Section One</h3> <p>Content One</p> <h3>Section Two</h3> <p>Content Two</p> <h3>Section Three</h3> <p>Content Three</p> ...
2. Include the latest version of jQuery library on the page.
<script src="https://code.jquery.com/jquery-3.3.1.min.js" integrity="sha384-tsQFqpEReu7ZLhBV2VZlAu7zcOV+rXbYlF2cqB8txI/8aZajjp4Bqd+V6D5IgvKT" crossorigin="anonymous"> </script>
3. The main jQuery script to generate the table of contents.
var toc = $('<ul></ul>').addClass("toc"); var el, title, link; $("article h3").each(function() { // Prepare the heading to become a link. Remove any special characters, replace spaces with dashes, change to lowercase var hyphenated = $(this).text().replace(/[^a-zA-Z0-9 ]/g, '') .replace(/\s/g,'-').toLowerCase(); // Convert the heading to its link version $(this).attr('id', hyphenated); // Create variables for text and link version el = $(this); title = el.text(); link = "#" + el.attr("id"); // Create a list item using the text and link newLine = "<li>" + "<a href='" + link + "'>" + title + "</a>" + "</li>"; // Add list item to table of contents toc.append(newLine); }); // Add heading to table of contents section toc.prepend('<h4>Table Of Contents:</h4>'); $('article').prepend(toc);
4. Enable the smooth scroll functionality on the table of contents.
toc.find('a').on('click', function () { var $el = $(this) , id = $el.attr('href'); $('html, body').animate({ scrollTop: $(id).offset().top }, 500); return false; });
5. Apply your own styles to the table of content.
.toc { padding: 1em; border-radius: 10px; background-color: rgba(255, 233, 138, 0.15); } .toc h4 { font-size: 18px; font-weight: 700; margin-bottom: 1em; text-transform: uppercase; color: rgba(255,132,132, 0.5); } .toc li { padding-left: 1em; margin-left: 1.25em; } .toc li a { text-decoration: none; color: #4a4a4a; } .toc li:hover, .toc li:hover a { color: orange; }
This awesome jQuery plugin is developed by von_designs. For more Advanced Usages, please check the demo page or visit the official website.