Fully Customizable Organisational Chart Plugin With jQuery - OrgChart
File Size: | 1.2 MB |
---|---|
Views Total: | 131322 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
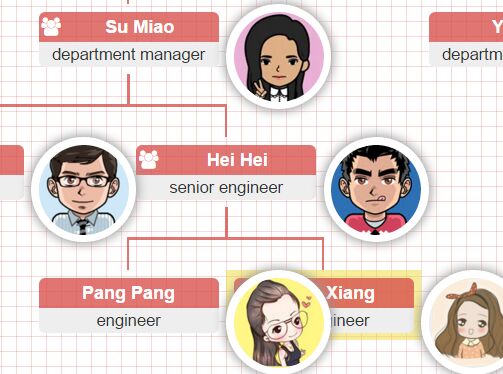
OrgChart is a simple, flexible and highly customizable organization chart plugin for presenting the structure of your organization and the relationships and relative ranks of its parts and positions/jobs in an elegant way.
Installation:
# NPM $ npm install orgchart --save # Bower $ bower install orgchart --save
Features:
- Supports both local data and remote data (JSON).
- Ajax enabled.
- On-demand data loading.
- Fully customizable structure & nodes.
- CSS3 based smooth transition effects.
- Expandable and collapsible.
- Avatars supported as well.
Basic usage:
1. Load Font Awesome (OPTIONAL) and the jQuery OrgChart plugin's style sheet in the head section of your document.
<link rel="stylesheet" href="font-awesome.min.css"> <link rel="stylesheet" href="css/jquery.orgchart.css">
2. Create a container for your organization chart.
<div id="chart-container"></div>
3. Load jQuery library and other required resources at the end of the document so the page loads faster.
<script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/js/jquery.orgchart.js"></script>
4. Prepare your data following the structure like this.
var datascource = { 'id': 'rootNode', // It's a optional property which will be used as id attribute of node // and data-parent attribute, which contains the id of the parent node 'collapsed': true, // By default, the children nodes of current node is hidden. 'className': 'top-level', // It's a optional property which will be used as className attribute of node. 'nodeTitlePro': 'Lao Lao', 'nodeContentPro': 'general manager', 'relationship': relationshipValue, // Note: when you activate ondemand loading nodes feature, // you should use json datsource (local or remote) and set this property. // This property implies that whether this node has parent node, siblings nodes or children nodes. // relationshipValue is a string composed of three "0/1" identifier. // First character stands for wether current node has parent node; // Scond character stands for wether current node has siblings nodes; // Third character stands for wether current node has children node. 'children': [ // The property stands for nested nodes. { 'nodeTitlePro': 'Bo Miao', 'nodeContentPro': 'department manager', 'relationship': '110' }, { 'nodeTitlePro': 'Su Miao', 'nodeContentPro': 'department manager', 'relationship': '111', 'children': [ { 'nodeTitlePro': 'Tie Hua', 'nodeContentPro': 'senior engineer', 'relationship': '110' }, { 'nodeTitlePro': 'Hei Hei', 'nodeContentPro': 'senior engineer', 'relationship': '110' } ] }, { 'nodeTitlePro': 'Yu Jie', 'nodeContentPro': 'department manager', 'relationship': '110' } ], 'otherPro': anyValue };
5. The JavaScript to render a basic organization chart inside the container you just created.
$('#chart-container').orgchart({ 'data' : datascource, 'depth': 2, 'nodeTitle': 'name', 'nodeContent': 'title' });
6. Load data from a remote JSON file via AJAX request.
$('#chart-container').orgchart({ 'data' : '/path/to/your/data', 'visibleLevel': 2, 'nodeTitle': 'name', 'nodeContent': 'title' });
7. Dynamically load data into the organization chart on demand.
var datasource = {...} $('#chart-container').orgchart({ 'data' : datascource, 'nodeTitle': 'name', 'nodeContent': 'title', 'nodeId': 'id' });
8. All default options.
{ 'icons': { 'theme': 'oci', 'parentNode': 'oci-menu', 'expandToUp': 'oci-chevron-up', 'collapseToDown': 'oci-chevron-down', 'collapseToLeft': 'oci-chevron-left', 'expandToRight': 'oci-chevron-right', 'collapsed': 'oci-plus-square', 'expanded': 'oci-minus-square', 'spinner': 'oci-spinner' }, 'nodeTitle': 'name', 'nodeId': 'id', 'toggleSiblingsResp': false, 'visibleLevel': 999, 'chartClass': '', 'exportButton': false, 'exportButtonName': 'Export', 'exportFilename': 'OrgChart', 'exportFileextension': 'png', 'draggable': false, 'direction': 't2b', 'pan': false, 'zoom': false, 'zoominLimit': 7, 'zoomoutLimit': 0.5 };
9. API methods.
// Adds parent node(actullay it's always root node) for current orgchart. instance.addParent(data) // Adds the ancestors for current orgchart. addAncestors(data, parentId) // Adds the descendants for specified parent node. addDescendants(data, $parent) // Adds sibling nodes for designated node. addSiblings($node, data) // Adds child nodes for designed node. addChildren($node, data) // Removes the designated node and its descedant nodes. removeNodes($node) // Gets the hierarchy relationships of orgchart for further processing. // For example, after editing the orgchart, you could send the returned value of this method to server-side and save the new state of orghcart. getHierarchy(includeNodeData) // Hides the parent node of any specific node(.node element). hideParent($node) // Shows the parent node of any specific node(.node element) showParent($node) // Hides the children of any specific node(.node element) hideChildren($node) // Shows the children of any specific node(.node element), if it has. showChildren($node) // Hides the siblings of any specific node(.node element), if it has. hideSiblings($node, direction) // Shows the siblings of any specific node(.node element), if it has. showSiblings($node, direction) // Returns the display state of related node of the specified node. getNodeState($node, relation) // Returns the nodes related to the specified node. getRelatedNodes($node, relation) // returns the parent node getParent($node) // returns the sibling nodes getSiblings($node) // returns the child nodes getChildren($node) // Set the scale setChartScale($chart, newScale) // Exports the current orgchart as png or pdf file. export(exportFilename, exportFileextension)
Changelog:
v4.0.1 (2023-12-22)
- update the demo of compact node chart
v4.0.0 (2023-12-17)
- remove the option "ajaxURL"
- remove the events "load-[relation].orgchart"
- add new method "addAncestors(data, parentId)"
- add new method "addDescendants(data, $parent)"
v3.8.2 (2023-12-01)
- abandon the optional URL string of option "data" and make enough space for users to build up their ajax requests
v3.8.1 (2023-11-26)
- bug-fix: compact nodes can not be opened after collapsing their siblings
v3.8.0 (2023-06-22)
- Users can use data prop "compact" or option "compact" to build up the compact layout.
v3.7.0 (2023-05-10)
- users are allowed to replace built-in icons with Font Awesome icons(or other icons).
v3.6.0 (2023-04-02)
- You can use crossorigin="anonymous" setting to export the charts with nodes containing pictures.
v3.5.0 (2023-02-23)
- Users are allowed to make a certain branch of the chart to be displayed as vertical by data.
v3.4.0 (2023-02-22)
- Users are allowed to drag&drop in the hybrid(horizontal + vertical) chart.
v3.2.0 (2023-01-31)
- refactor
v3.1.4 (2022-12-18)
- fixed bug: drag & drop not working on Firefox
v3.1.3 (2022-11-16)
- position node in specific level
v3.1.2 (2022-11-13)
- expose methods -- getParent(), getSiblings(), getChildren()
v3.1.1 (2021-02-20)
- Adjust the position of export button
v3.1.0 (2021-02-18)
- Allow users to edit the hybrid chart
v3.0.0 (2021-02-15)
- Refactor orgchart with nested ul
v2.2.0 (2021-01-07)
- Added option to change exportButtonName
- Added option to getHierarchy to include nodeData
- Add events for ajax insert, show and hide
2020-01-21
- v2.1.10: add oci icons; remove font-awesome dependency
2019-10-11
- v2.1.9: fix: missing folder dist
2019-10-09
- v2.1.8 feat: update html2canvas
2019-10-06
- v2.1.7: Updated
2019-08-20
- v2.1.5: Update basic styles
2018-08-18
- v2.1.4: Upgrade unit testing based on gulp 4
2018-08-14
- v2.1.3: Fixed drag & drop not working on Firefox
2018-08-12
- v2.1.2: add data property nodeData for every node
2018-01-20
- v2.1.0: Refactor the core methods buildHierarchy()
2018-01-20
- v2.1.0: Refactor the core methods buildHierarchy()
2018-01-12
- v2.0.16: Add support for drag-and-drop of nodes on touch devices
2018-01-02
- v2.0.15: update
2017-12-21
- v2.0.14: refactor the method getHierarchy()
2017-12-17
- v2.0.12: fix-bug: drag&drop broken;extract out nested callback handler for tansitionend event
2017-12-13
- v2.0.11: fix-bug: Nodes alignement
2017-12-12
- v2.0.10: remove all :visible selector from the js source code; fix-bug: conflict between depth and verticalDepth; refactor the method init(); refactor the method createNode()
2017-11-24
- v2.0.9: rename nodedropped.orgchart to nodedrop.orgchart
2017-11-08
- v2.0.8: improve ccs performance
2017-11-06
- v2.0.7
2017-11-05
- fix: call ajaxSettings.beforeSend when loading chart
2017-11-02
- update version to 2.0.5
- Adjust CSS
2017-07-15
- expose the new option nodeTemplate
2017-07-13
- fix bug: setOptions()
2017-07-11
- provid setOptions() method
2017-07-08
- fix the bug of init method
2017-07-07
- fix the bug of ajax-datasource example
- fix(bug): refactor the examples based on new OrgChart class
2017-07-04
- fix(events): trigger init event on orgchart div
2017-07-03
- add initCompleted callback
2017-04-14
- expose setChartScale() method
2017-03-06
- Refactor the method showChildren
2017-03-05
- Users are allowed to set a zoom-in&zoom-out limit
2017-02-09
- Fix export button default click event
2016-10-13
- Allow functions in AjaxURLS option
- CSS update
- v1.2.6
2016-10-09
- node will not be selected when users expand/collapse the nodes
2016-09-22
- v1.2.3: Enable option zoom on mobile device
2016-09-21
- Fix-bug: resolve the collision between option direction and draggable
2016-09-20
- Fix-bug: if orgchart has been transformed, the ghost image is out of shape when drag&drop
2016-09-12
- js update
2016-08-30
- Fix-bug: drag and drop in IE is not working
2016-07-29
- remove the option nodeChildren
2016-07-16
- JS & CSS update
2016-06-28
- append option dropCriteria
2016-06-27
- fix-bug: export doesn't work well when chart-container has overflow:hidden
2016-06-24
- split option panzoom into pan and zoom
2016-06-23
- v1.0.9
2016-06-14
- set dataType to json for all ajax
2016-05-23
- added a new options: 'panzoom'
2016-05-11
- added a new options: 'pan'
2016-05-07
- bugfix: GetHierarchy method doesn't work properly when draggable option is on
2016-04-27
- fix-bug: drag&drop feature doesn't work in FireFox
2016-04-26
- bugfix
2016-04-22
- JS update
2016-04-14
- refactor the function buildJsonDS()
2016-04-09
- refactor the method addParent()
2016-03-31
- reduce plugin size.
2016-03-18
- refactor the internal structure of orgchart plugin
2016-03-06
- bugfix
This awesome jQuery plugin is developed by dabeng. For more Advanced Usages, please check the demo page or visit the official website.