Nice Tags Manager with jQuery and Bootstrap - Bootstrap Tags Input
File Size: | 186 KB |
---|---|
Views Total: | 27948 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
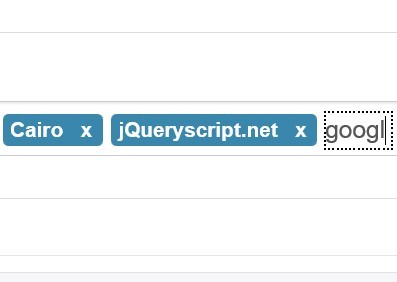
Bootstrap Tags Input is a jQuery plugin that allows you to add, remove, and categorize tags in Bootstrap-powered projects. Compatible with Bootstrap 5/4/3/2.
See also:
- Versatile jQuery Tags Input Plugin - Tag Handler
- jQuery Tags Manager with Twitter Bootstrap - tagmanager
- Bootstrap 4 Tag Input Plugin With jQuery - Tagsinput.js
- jQuery Tag & Token Input Plugin For Bootstrap - Bootstrap Tokenfield
How to use it:
1. Include jQuery library and the jQuery Bootstrap Tags Input plugin on the web page.
<!-- jQuery is required --> <script src="/path/to/cdn/jquery.min.js"></script> <!-- Bootstrap Tags Input Plugin --> <link rel="stylesheet" href="/path/to/dist/bootstrap-tagsinput.css" /> <script src="/path/to/bootstrap-tagsinput.min.js"></script>
2. Include the latest Twitter Bootstrap framework on the page.
<link rel="stylesheet" href="/path/to/cdn/bootstrap.min.css" /> <script src="/path/to/cdn/bootstrap.min.js"></script>
3. Create a input field for the tags manager and auto-init the plugin using the data-role="tagsinput"
attribute.
<input type="text" value="Amsterdam,Washington,Sydney,Beijing,Cairo" data-role="tagsinput" />
// Required For Bootstrap 5 & 4 $('input').tagsinput({ tagClass: 'badge bg-info', });
4. The plugin also works with select
elements.
<select multiple data-role="tagsinput"> <option value="Amsterdam">Amsterdam</option> <option value="Washington">Washington</option> <option value="Sydney">Sydney</option> <option value="Beijing">Beijing</option> <option value="Cairo">Cairo</option> </select>
5. Integrate the plugin with the typeahead.js autocomplete library.
var citynames = new Bloodhound({ datumTokenizer: Bloodhound.tokenizers.obj.whitespace('name'), queryTokenizer: Bloodhound.tokenizers.whitespace, prefetch: { url: 'assets/citynames.json', filter: function(list) { return $.map(list, function(cityname) { return { name: cityname }; }); } } }); citynames.initialize(); $('input').tagsinput({ typeaheadjs: { name: 'citynames', displayKey: 'name', valueKey: 'name', source: citynames.ttAdapter() } });
6. Categorize tags using custom label/badge classes.
var cities = new Bloodhound({ datumTokenizer: Bloodhound.tokenizers.obj.whitespace('text'), queryTokenizer: Bloodhound.tokenizers.whitespace, prefetch: 'assets/cities.json' }); cities.initialize(); var elt = $('input'); elt.tagsinput({ tagClass: function(item) { switch (item.continent) { case 'Europe' : return 'badge badge-primary'; case 'America' : return 'badge badge-danger badge-important'; case 'Australia': return 'badge badge-success'; case 'Africa' : return 'badge badge-default'; case 'Asia' : return 'badge badge-warning'; } }, itemValue: 'value', itemText: 'text', typeaheadjs: { name: 'cities', displayKey: 'text', source: cities.ttAdapter() } });
7. All default plugin options.
$('input').tagsinput({ tagClass: function(item) { // 'badge bg-info' for BS 5 & 4 return 'label label-info'; }, focusClass: 'focus', itemValue: function(item) { return item ? item.toString() : item; }, itemText: function(item) { return this.itemValue(item); }, itemTitle: function(item) { return null; }, // allow to add tags which are not returned by typeahead's source freeInput: true, addOnBlur: true, maxTags: undefined, maxChars: undefined, // ENTER and comma confirmKeys: [13, 44], delimiter: ',', delimiterRegex: null, cancelConfirmKeysOnEmpty: false, onTagExists: function(item, $tag) { $tag.hide().fadeIn(); }, // remove all whitespace around tags trimValue: false, allowDuplicates: false, riggerChanget: true });
8. API methods.
// add tag(s) $('input').tagsinput('add', 'Another Tag'); $('input').tagsinput('add', { id: 10, text: 'Another Tag'}); // remove tag(s) $('input').tagsinput('remove', 'some tag'); $('input').tagsinput('remove', { id: 1, text: 'some tag' }); // remove all tags $('input').tagsinput('removeAll'); // set focus on the tags input $('input').tagsinput('focus'); // refresh the tags input $('input').tagsinput('refresh'); // destroy the instance $('input').tagsinput('destroy');
9. Events.
$('input').on('itemAddedOnInit', function(event) { // event.item: contains the item }); $('input').on('beforeItemAdd', function(event) { // event.item: contains the item // event.cancel: set to true to prevent the item getting added }); $('input').on('itemAdded', function(event) { // event.item: contains the item }); $('input').on('beforeItemRemove', function(event) { // event.item: contains the item // event.cancel: set to true to prevent the item getting removed }); $('input').on('itemRemoved', function(event) { // event.item: contains the item });
Changelog:
2023-06-20
- Added Bootstrap 5/4 usages.
v0.8.0 (2022-04-11)
- New version
v0.7.1 (2015-11-11)
- allowDuplicates not working
- tag text appears when typeahead input looses focus
- Remove duplicate method removeAll in manual
v0.7.0 (2015-11-09)
- .tt-menu etc. styles should be included in bootstrap-tagsinput.css by default
- Comma character carried over to new tag input when used as separator
- Emails in multi select are being duplicated
- The 'itemAdded' Event run on Load the Page!
v0.6.1 (2015-11-03)
- Updated uglify to fix source maps generation issue
v0.5.1 (2015-03-26)
- update.
v0.4.2 (2014-08-01)
- Fix typeahead when using Bootstrap 3
v0.3.14 (2014-07-31)
- Renamed itemKey to valueKey
v0.3.14 (2014-07-27)
- Bugs fixed.
v0.3.10 (2014-07-17)
- update.
v0.3.9 (2013-10-15)
- Fixed Type ahead stops when entering second character
v0.3.8 (2013-10-15)
- Add support for placeholder
- ie 8 compatibility, replace indexOf method
v0.3.7 (2013-10-15)
- fixed: flash when duplicate is entered
v0.3.6 (2013-10-06)
- Only add existing value as tags when using strings as tags
- Implemented confirmKeys option
This awesome jQuery plugin is developed by bootstrap-tagsinput. For more Advanced Usages, please check the demo page or visit the official website.