jQuery Autocomplete Plugin By Twitter - typeahead.js
File Size: | 147 KB |
---|---|
Views Total: | 11786 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
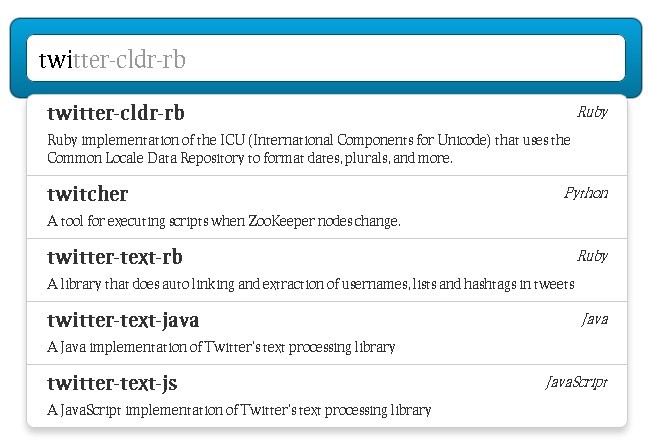
A robust, flexible, smart, fast autocomplete/typeahead library inspired by Twitter's autocomplete search functionality.
The plugin also provides an advanced suggestion engine named bloodhound.js which offers advanced functionalities such as prefetching, intelligent caching, fast lookups, and backfilling with remote data.
Main Features:
- Displays suggestions to end-users as they type
- Shows top suggestion as a hint (i.e. background text)
- Works with hardcoded data as well as remote data
- Rate-limits network requests to lighten the load
- Allows for suggestions to be drawn from multiple datasets
- Supports customized templates for suggestions
- Plays nice with RTL languages and input method editors
You might also like:
- jQuery Ajax Autocomplete Plugin For Input Fields - Autocomplete
- Tag input & Autocomplete Plugin - TextExt
Basic Usage:
1. Include the typeahead.js plugin and other required resources on the webpage.
<script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/dist/bloodhound.min.js"></script> <script src="/path/to/dist/typeahead.jquery.min.js"></script> <!-- OR --> <script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/dist/typeahead.bundle.min.js"></script>
2. Create an input field on which the typeahead.js plugin will be enabled.
<div class="demo"> <input class="typeahead" type="text" placeholder="countries"> </div>
3. Initialize the plugin on the input field and define the data sources as follows:
var myData = ['Alabama', 'Alaska', 'Arizona']; var substringMatcher = function(strs) { return function findMatches(q, cb) { var matches, substringRegex; // an array that will be populated with substring matches matches = []; // regex used to determine if a string contains the substring `q` substrRegex = new RegExp(q, 'i'); // iterate through the pool of strings and for any string that // contains the substring `q`, add it to the `matches` array $.each(strs, function(i, str) { if (substrRegex.test(str)) { matches.push(str); } }); cb(matches); }; }; $('#.typeahead').typeahead({ // options here }, { name: 'myData', source: substringMatcher(myData) });
4. With bloodhound.js suggestion engine.
var myData = new Bloodhound({ datumTokenizer: Bloodhound.tokenizers.whitespace, queryTokenizer: Bloodhound.tokenizers.whitespace, local: myData }); $('#bloodhound .typeahead').typeahead({ // options }, { name: 'myData', source: myData });
5. Possible options & dataset settings for typeahead.js.
$('#.typeahead').typeahead({ // highlights search queries highlight: false, // shows/hides hint hint: true. // minimum character length to trigger the suggestion list minLength: 1, // default CSS classes classNames: { wrapper: "twitter-typeahead", input: "tt-input", hint: "tt-hint", menu: "tt-menu", dataset: "tt-dataset", suggestion: "tt-suggestion", selectable: "tt-selectable", empty: "tt-empty", open: "tt-open", cursor: "tt-cursor", highlight: "tt-highlight" } }, { // data source // a function with the signature (query, syncResults, asyncResults) source: data // if the source function expects 3 arguments, async will be set to true async: false // the name of the dataset name: '', // specify the maximum number of suggestions to display limit: 5, // either a key string or a function that transforms a suggestion object into a string display: null, // custom rendering template. templates: { notFound: null, empty: null, pending: null, header: null, footer: null, suggestion: null } });
6. Possible options for bloodhound.js.
new Bloodhound({ // A function with the signature (datum) that transforms a datum into an array of string tokens. Required. datumTokenizer: function(datum) {}, // A function with the signature (query) that transforms a query into an array of string tokens. Required. queryTokenizer: function(datum) {}, // If set to false, the Bloodhound instance will not be implicitly initialized by the constructor function. initialize: true, // return a unique id identify: JSON.stringify, // If the number of datums provided from the internal search index is less than sufficient, remote will be used to backfill search requests triggered by calling #search. sufficient: 5 // A compare function used to sort data returned from the internal search index. sorter: function(){}, // An array of data or a function that returns an array of data. The data will be added to the internal search index when #initialize is called. local: [], // Can be a URL to a JSON file containing an array of data or, if more configurability is needed, a prefetch options hash. prefetch: '', // Can be a URL to fetch data from when the data provided by the internal search index is insufficient or, if more configurability is needed, a remote options hash. remote: '' });
Changelog:
v0.11.1 (2015-04-28)
- update.
v0.10.4 (2014-07-14)
- update.
v0.10.3 (2014-07-12)
- Bloodhound#clearPrefecthCache now works with cache keys that contain regex characters.
- Prevent outdated network requests from being sent.
- Add support to object tokenizers for multiple property tokenization.
- Fix broken jQuery#typeahead('val') method.
- Remove disabled attribute from the hint input control.
- Add tt-highlight class to highlighted text.
- Handle non-string types that are passed to jQuery#typeahead('val', val).
v0.10.2 (2014-03-12)
- update
v0.10.1 (2014-02-10)
- hot fixes
v0.10.0 (2014-02-02)
- update to the latest version.
v0.9.3 (2013-06-26)
- Ensure cursor visibility in menus with overflow.
- Fixed bug that led to the menu staying open when it should have been closed.
- Private browsing in Safari no longer breaks prefetch.
- Pressing tab while a suggestion is highlighted now results in a selection.
- Dataset name is now passed as an argument for typeahead:selected event.
v0.9.2 (2013-04-15)
- Prefetch usage no longer breaks when cookies are disabled.
- Precompiled templates are now wrapped in the appropriate DOM element.
v0.9.1 (2013-04-01)
- Multiple requests no longer get sent for a query when datasets share a remote source.
- Datasets now support precompiled templates.
- Cached remote suggestions now get rendered immediately.
- Added typeahead:autocompleted event.
- Added a plugin method for programmatically setting the query. Experimental.
- Added minLength option for datasets. Experimental.
- Prefetch objects now support thumbprint option. Experimental.
v0.9.0 (2013-03-24)
- Implemented the triggering of custom events.
- Got rid of typeahead.css and now apply styling through JavaScript.
- Made the API more flexible and addressed a handful of remote issues by rewriting the transport component.
- Added support for dataset headers and footers.
- No longer cache unnamed datasets.
- Made the key name of the value property configurable.
- Input values set before initialization of typeaheads are now respected.
- Fixed an input value/hint casing bug.
This awesome jQuery plugin is developed by twitter. For more Advanced Usages, please check the demo page or visit the official website.