jQuery Plugin For Easy Client Side Form Validation - bValidator
File Size: | 32.9 KB |
---|---|
Views Total: | 12191 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
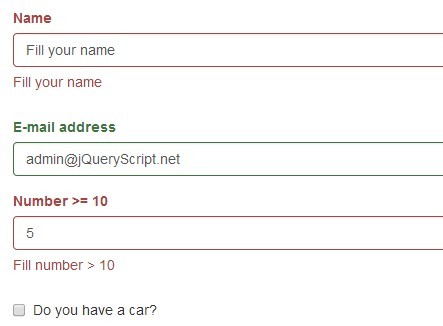
bValidator is a simple jQuery plugin for executing client side validation as you submit a form, displaying error & hint messages next to the invalid inputs.
How to use it:
1. Include the jQuery Javascript library and the jQuery bValidator plugin at the end of your document.
<script src="//code.jquery.com/jquery-1.11.1.min.js"></script> <script src="bvalidator.jquery.js"></script>
2. All you have to do is add custom HTML5 data-*
attributes to your form controls. The plugin comes with a lot of pre-defined validation rules as shown below. You can also create your own rules using regular expression.
data-bvStrict="email":
email address validationdata-bvStrict="phone":
phone number validation. 9 digits.data-bvStrict="number":
number validation.data-bvStrict="number:1":
number > 1data-bvStrict="number::10":
number < 10data-bvStrict="number:1:10":
1 < number <10data-bvStrict="notEmpty":
input can't be emptydata-bvStrict="empty":
input has to be emptydata-bvStrict="string":
input has to be stringsdata-bvStrict="date:format":
date format validationdata-bvStrict="checked":
checkbox has to be checkeddata-bvStrict="reg:^[\d]{2,3}$":
validate input by regular expressiondata-bvSwitch:
placeholderdata-bvAppend:
Add suffix to a valuedata-bvPrepend:
Add prefix to a valuedata-bvEmpty:
Sets value to a character, if input is empty.data-bvTransform:
Erasing spaces from value
<form> <div class="form-row"> <input type="text" data-bvStrict="notEmpty" data-bvSwitch="Fill your name"> <div class="help-block error-message">Fill your name</div> </div> <div class="form-row"> <input type="text" data-bvStrict="email" data-bvEmpty="@"> <div class="help-block error-message">Fill valid e-mail address</div> </div> <div class="form-row"> <input type="password" data-bvStrict="reg:^.{5,}"> <div class="help-block error-message">Password must have at least 5 letters</div> </div> ... </form>
3. Add some CSS to hide the error message on initialization and to style the input fields based on the validation status.
form .help-block.error-message { color: #a94442 } form .help-block.error-message.error { display: block } form .help-block.valid-message { color: #3c763d } form .row.error .error-message { display: block } form .row.error input { border-color: #a94442 } form .row.valid .valid-message { display: block } form .row.valid input { border-color: #3c763d } form input.error { border-color: #a94442 } form input.valid { border-color: #3c763d } form label.error { color: #a94442 } form label.valid { color: #3c763d }
4. Call the plugin to initialize the form validator.
$('#form-row').bValidator();
5. Available options.
// Selectors errorClass: 'error', errorMessageClass: 'error-message', validClass: 'valid', rowClass: 'row', // Hide error after focusing input onFocusHideError: false, // If true, add extra on key up validation onKeyUpValidate: false, // Other options: 'direct' domType: 'row', // Callback functions onValid: function() {}, onInvalid: function() {}, beforeSubmit: function() {}, onSubmitFail: function() {}, onFocusIn: function() {}, onFocusOut: function() {}, onKeyUp: function() {}
6. Add custom validation rules using validation()
method.
jQuery.bValidator.validation('rule_name', function(value, args, elem) { return true/false; });
This awesome jQuery plugin is developed by jBenes. For more Advanced Usages, please check the demo page or visit the official website.