Mobile-friendly Drawer Navigation JavaScript Library - hy-drawer
File Size: | 184 KB |
---|---|
Views Total: | 10331 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
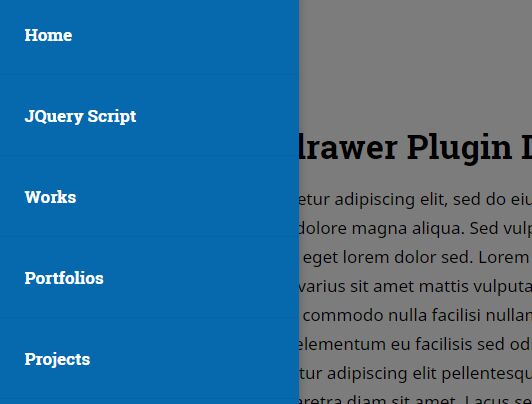
hy-drawer is an easy, performant drawer navigation JavaScript library which enables a trigger element to toggle a mobile app-style off-canvas menu for modern cross-platform web design. Can be implemented as jQuery plugin, Vanilla JavaScript class or Web Component.
How to use it:
1. In this post, we're going to implement the hy-drawer library as jQuery plugin. So you first need to load the latest jQuery library in the document.
<script src="https://code.jquery.com/jquery-3.2.1.slim.min.js" integrity="sha384-KJ3o2DKtIkvYIK3UENzmM7KCkRr/rE9/Qpg6aAZGJwFDMVNA/GpGFF93hXpG5KkN" crossorigin="anonymous"> </script>
2. Load the hy-drawer plugin's JavaScript and Stylesheet in the document.
<link href="/dist/jquer/style.css" rel="stylesheet"> <script src="/dist/jquer/hy-drawer.js"></script>
3. Create the drawer navigation from a normal nav list.
<aside id="drawerEl"> <ul> <li><a href="#">Home</a></li> <li><a href="#">JQuery Script</a></li> <li><a href="#">Works</a></li> <li><a href="#">Portfolios</a></li> <li><a href="#">Projects</a></li> <li><a href="#">Contact</a></li> <li><a href="#">About</a></li> </ul> </aside>
4. Create a hamburger menu toggle button inside your main content.
<a id="menuEl" href="#drawerEl"><span class="sr-only">Menu</span></a>
5. Enable the hamburger icon to open the drawer navigation.
$('#drawerEl').drawer({ // options here }) $('#menuEl').click(function (e) { e.preventDefault(); $('#drawerEl').drawer('toggle'); });
6. All possible plugins with default options.
$('#drawerEl').drawer({ // is opened on page load? opened: false, // alignment of the drawer navigation // left, right align: 'left', // the range in pixels from either left or right side of the screen (depending on alignment) from within which the drawer can be drawn range: [0, 100], // is in 'persistent' state? // true = drawer can't be moved with touch events persistent: false, // true = call preventDefault on every (touch-)move event, effectively preventing scrolling while sliding the drawer preventDefault: false, // in pixels threshold: 10, // allows the drawer to be pulled with the mouse mouseEvents: false })
6. You can also pass the options via data-OPTIONNAME
as follows:
<aside id="drawerEl" data-prevent-default="true" data-mouse-events="true"> <ul> <li><a href="#">Home</a></li> <li><a href="#">JQuery Script</a></li> <li><a href="#">Works</a></li> <li><a href="#">Portfolios</a></li> <li><a href="#">Projects</a></li> <li><a href="#">Contact</a></li> <li><a href="#">About</a></li> </ul> </aside>
7. API methods.
// toggle the drawer navigation $('#drawerEl').drawer('toggle'); // open the drawer navigation $('#drawerEl').drawer('open'); // close the drawer navigation $('#drawerEl').drawer('close');
8. Event handlers.
$('#drawerEl').drawer({ // options here }) .on('transitioned.hy.drawer', function(e) { // fired after a completed transitioning to the new opened state }); $('#drawerEl').drawer({ // options here }) .on('hy-drawer-init', function(e) { // fired after the navigation has been initialized }); $('#drawerEl').drawer({ // options here }) .on('hy-drawer-slidestart', function(e) { // fired when the user starts sliding the drawer }); $('#drawerEl').drawer({ // options here }) .on('hy-drawer-slideend', function(e) { // when the user stops sliding the drawer });
Changelog:
2018-09-01
- 1.0.0-pre.25
This awesome jQuery plugin is developed by qwtel. For more Advanced Usages, please check the demo page or visit the official website.