Basic Responsive Header Dropdown Menu With jQuery And CSS3
File Size: | 3.32 KB |
---|---|
Views Total: | 4499 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
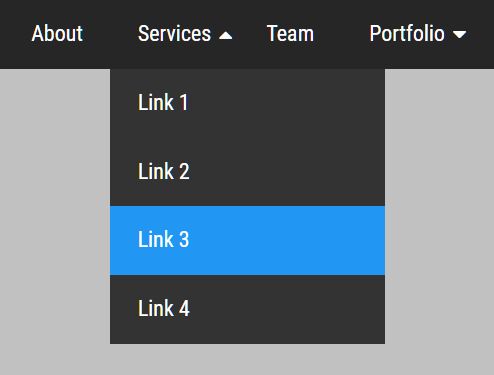
A responsive dropdown menu for header navigation that utilizes CSS3 media queries to detect the screen size and collapses horizontal menu items into a fullscreen sliding toggle menu when the maximum screen width is reached.
How to use it:
1. Create a multi-level header navigation from a nested nav list as follows:
<header> <div class="logo">YOUR LOGO HERE</div> <nav> <ul> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li class="sub-menu"><a href="#">Services</a> <ul> <li><a href="#">Link 1</a></li> <li><a href="#">Link 2</a></li> <li><a href="#">Link 3</a></li> <li><a href="#">Link 4</a></li> </ul> </li> <li><a href="#">Team</a></li> <li class="sub-menu"><a href="#">Portfolio</a> <ul> <li><a href="#">Link 1</a></li> <li><a href="#">Link 2</a></li> <li><a href="#">Link 3</a></li> <li><a href="#">Link 4</a></li> </ul> </li> <li><a href="#">Contact</a></li> </ul> </nav> <div class="menu-toggle"> Menu Toggler Here </div> </header>
2. The CSS styles for the regular dropdown menu.
/* Header */ header { position: absolute; top: 0; left: 0; padding: 0 100px; background: #262626; width: 100%; box-sizing: border-box; } /* LOGO */ header .logo { color: #FFF; height: 50px; line-height: 50px; font-size: 24px; float: left; font-weight: bold; } /* Dropdown Nav */ header nav { float: right; } /* UL */ header nav ul { margin: 0; padding: 0; display: flex; } /* Nav Items */ header nav ul li { list-style: none; position: relative; } /* Sub-menu */ header nav ul li.sub-menu:before { content: '\f0d7'; font-family: fontAwesome; position: absolute; line-height: 50px; color: #FFF; right: 5px; cursor: pointer; } header nav ul li.active.sub-menu:before { content: '\f0d8'; } header nav ul li ul { position: absolute; left: 0; background: #333; display: none; } header nav ul li.active ul { display: block; } header nav ul li ul li { display: block; width: 200px; } header nav ul li a { height: 50px; line-height: 50px; padding: 0 20px; color: #FFF; text-decoration: none; display: block; } header nav ul li a:hover, header nav ul li a.active { color: #FFF; background: #2196f3; }
3. The CSS styles for the toggle menu.
/* Toggle Button */ .menu-toggle { color: #FFF; float: right; line-height: 50px; font-size: 24px; cursor: pointer; display: none; } /* Media Queries */ @media (max-width: 991px) { header { padding: 0 20px; } .menu-toggle { display: block; } header nav { position: absolute; width: 100%; height: calc(100vh - 50px); background: #333; top: 50px; left: -100%; transition: 0.5s; } header nav.active { left: 0; } header nav ul { display: block; text-align: center; } header nav ul li a { border-bottom: 1px solid rgba(0,0,0,.2); } header nav ul li.active ul { position: relative; background: #003e6f; } header nav ul li ul li { width: 100%; } }
4. The core JavaScript to enable the toggle button to open/close the menu when running on small screens.
$(document).ready(function(){ $('.menu-toggle').click(function(){ $('nav').toggleClass('active'); }); $('ul li').click(function(){ $(this).siblings().removeClass('active'); $(this).toggleClass('active'); }) })
This awesome jQuery plugin is developed by leosantosp. For more Advanced Usages, please check the demo page or visit the official website.