Create Notifications In A Specific Container - Module Notification
File Size: | 375 KB |
---|---|
Views Total: | 628 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
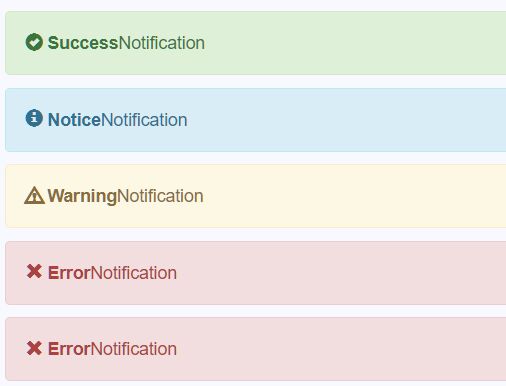
Module Notification is a JavaScript/jQuery plugin that helps you create and display notification messages in a container element you specify.
Features:
- Support notification groups.
- Custom notification icons based on Bootstrap Glyphicons.
- Custom notification template.
- Success/Warning/Notice/Error types.
- Top or bottom position.
- Auto dismisses after a timeout just like the toast.
How to use it:
1. Install and download the Module Notification plugin.
# Yarn $ yarn add module-notification # NPM $ npm install module-notification --save
2. Add references to the Module Notification plugin's JavaScript and CSS files.
<!-- Bootstrap Glyphicons are included in this file --> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/module-notification@latest/dist/module-notification.css"> <!-- jQuery library is required --> <script src="/path/to/jquery.min.js"></script>
3. Create a container element in which the notification message will display.
<div id="notiContainer"> </div>
4. Create a new module notification instance.
let myMNModule = new MNModule({ container: "#notifications" });
5. Display your notification in the container element using the pushNotif
method.
let myNotif = myMNModule.pushNotif({ title: "Notification Title", message: "Notification Message" });
6. Create a notification group. Set the greedy
option to true in case you'd like to have only one elements to display at a time.
myMNModule.createEmptyGroup({ name: "myGroup", greedy: false });
7. Default module options.
let myMNModule = new MNModule({ // target container container: "#notifications", //e.g. (number) => { console.debug("Number of notifications", number); }, onNotifsNumberChange: undefined, // or "fromBottom" direction: "fromTop" });
8. Customize the notification using the following options.
let myNotif = myMNModule.pushNotif({ // notification title title: "", // notification message message: "", // auto dismiss after 5000ms closeCond: 5000, // group name group: "common", // custom template // e.g. function(title, message) { return "<span>" + title + "</span>"; } template: undefined, // Bootstrap Glyphicons name // e.g. "ok-sign" icon: undefined, //"notice", "warning", "error", "success" type: "notice" });
This awesome jQuery plugin is developed by vadimkorr. For more Advanced Usages, please check the demo page or visit the official website.