Modern Custom Radio Buttons with jQuery and CSS3
File Size: | 2.05 MB |
---|---|
Views Total: | 3331 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
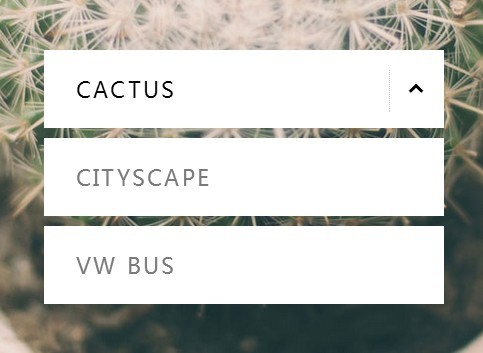
A tiny jQuery plugin that transforms your radio buttons into a modern dropdown select list with background images interaction. Great for creating an image filter that fades in/out the background images depending on the option you choose.
How to use it:
1. Create several radio buttons for an image filter.
<div class="container"> <div class="filter-wrapper"> <div class="image-filters"> <input id="filter-1" type="radio" name="radio-set"> <label for="filter-1">Filter 1</label> <input id="filter-2" type="radio" name="radio-set"> <label for="filter-2">Filter 2</label> <input id="filter-3" type="radio" name="radio-set"> <label for="filter-3">Filter 3</label> </div> </div> </div>
2. Include jQuery library and the custom radio button plugin at the end of your web page.
<script src="//ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> <script src="js/main.js"></script>
3. The required CSS styles for the plugin.
html, body { height: 100%; } body { background-size: cover; -webkit-background-size: cover; -moz-background-size: cover; -o-background-size: cover; background-repeat: no-repeat; background-attachment: fixed; background-position: center; transform: translate3d(0, 0, 0); -webkit-backface-visibility: hidden; -webkit-perspective: 1000; backface-visibility: hidden; perspective: 1000; font: 100% 'Montserrat', sans-serif; color: #7a7a7a; transition: background-image .5s ease-in; -webkit-transition: background-image .5s ease-in; -moz-transition: background-image .5s ease-in; -o-transition: background-image .5s ease-in; background-color: #fff; } a:link, a:visited { color: #7a7a7a; text-decoration: none; } @-webkit-keyframes fade-in { 0% { opacity: 0; } 50% { opacity: 0; } 100% { opacity: 1; } } @-moz-keyframes fade-in { 0% { opacity: 0; } 50% { opacity: 0; } 100% { opacity: 1; } } .fade-in { -webkit-animation-name: fade-in; -webkit-animation-duration: .6s; -webkit-animation-timing-function: ease-in; -moz-animation-name: fade-in; -moz-animation-duration: .6s; -moz-animation-timing-function: ease-in; } .container { height: 100%; min-height: 100%; text-align: center; width: 100%; display: table; } .container .filter-wrapper { display: table-cell; vertical-align: middle; } .image-filters { display: inline-block; vertical-align: top; text-align: left; position: relative; width: 400px; } .image-filters.active input:checked + label { color: #000; } .image-filters.active input:checked + label:after { content: "\f077"; display: inline-block; font-family: FontAwesome; font-style: normal; font-weight: normal; line-height: 1; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; color: #000; } .image-filters input { display: none; } .image-filters input:checked + label { z-index: 20; } .image-filters input:checked + label:after { content: "\f078"; display: inline-block; font-family: FontAwesome; font-style: normal; font-weight: normal; line-height: 1; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; text-align: center; padding: 10px 18px 15px 18px; border-left: 1px dotted #ccc; position: absolute; top: 25%; height: 55%; right: 0; font-size: .7em; } .image-filters input:not(:checked) + label:hover { background-color: #000; color: #fff; transition: all 0.3s ease-in; -webkit-transition: all 0.3s ease-in; -moz-transition: all 0.3s ease-in; -o-transition: all 0.3s ease-in; } .image-filters label { width: 100%; white-space: nowrap; overflow: hidden; text-overflow: ellipsis; top: 0; position: absolute; background: #fff; padding: 6% 8%; margin-bottom: 10px; z-index: 10; display: block; cursor: pointer; letter-spacing: .1em; text-transform: uppercase; font-size: 1.4em; } @media (max-width: 440px) { .container .filter-wrapper { vertical-align: top; padding-top: 10%; } .image-filters { width: 300px; } .image-filters input:checked + label:after { padding-top: 8px; } .image-filters label { font-size: 1.2em; }
4. Initialize the plugin with options.
$( function() { $('.image-filters').customradio({ mobileImages: true, // loads different images on mobile devices mobileSize: 640, imgsUrl: 'imgs/' // must be have the same name as the ID of the respective input/label. }); });
This awesome jQuery plugin is developed by ehayman. For more Advanced Usages, please check the demo page or visit the official website.