Real-time Form Validation & Auto Formatting Plugin - field-validator.js
File Size: | 24.8 KB |
---|---|
Views Total: | 3447 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
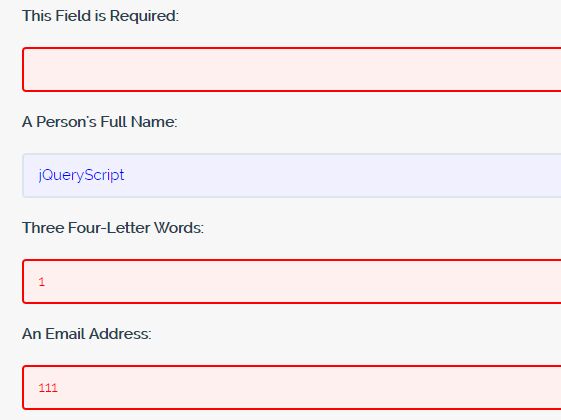
field-validator.js is a simple, powerful jQuery form validation plugin which enables you to validate and auto-format form fields on the client side.
More features:
- A set of pre-defined validators.
- Auto formats input values as typing.
- Auto validates & formats input fields when you jump to the next one.
- Custom background & styles for valid and invalid form fields.
Validators included:
- Email.
- Required.
- Credit card.
- Date and time.
- Custom validators using REGEX.
Basic usage:
1. Include the latest version of jQuery library and the jQuery field-validator.js
script on the web page.
<script src="//code.jquery.com/jquery-3.2.1.min.js"></script> <script src="field-validator.js"></script>
2. Add validations and custom validation rules to your form fields as follows:
<p> <div><label>This Field is Required:</label> <input type="text" id="req" class="form-control" required /></div> <div><label>A Person's Full Name:</label> <input type="text" class="form-control" pattern="^[a-zA-Z ,.'-]+$" /></div> <div><label>Three Four-Letter Words:</label> <input type="text" class="form-control" pattern="^[a-zA-Z]{4} [a-zA-Z]{4} [a-zA-Z]{4}$" /></div> <div><label>An Email Address:</label> <input type="text" class="form-control" data-type="email" /></div> </p> <p> <div><label>A Number:</label> <input type="text" class="form-control" pattern="^[0-9]*$" /></div> <div><label>A Single Digit:</label> <input type="text" class="form-control" pattern="^[0-9]$" /></div> <div><label>An Amount of Money:</label> <input type="text" class="form-control" data-type="money" /></div> <div><label>A Credit Card Number:</label> <input type="text" class="form-control" data-type="creditcard" data-cardicons="on" data-discover="on" data-amex="on" /></div> </p> <p> <div><label>This Field Will Become Title Case:</label> <input type="text" class="form-control" data-format="titlecase" /></div> <div><label>This Number Will Be Padded:</label> <input type="text" class="form-control" pattern="^[0-9]{1,10}$" data-pad="10" /></div> <div><label>Type a Date, Nearly Any Format Will Work:</label> <input type="text" class="form-control" data-type="date" /></div> </p>
3. Customize the background color of invalid form fields.
fieldValidator.backgroundInvalid = "rgb(255, 240, 240)";
4. Customize the CSS styles of invalid form fields.
.form-error { ... }
5. All customization options.
{ fieldSelector: ".form-control", errorSelector: ".form-error", inputDelay: 2000, animDelay: 500, colorValid: "blue", colorInvalid: "red", borderValid: undefined, borderInvalid: "red", backgroundValid: undefined, backgroundInvalid: undefined, describe: function (element, value) { function ident() { if ($(element).attr("id")) { return "#" + $(element).attr("id"); } else if ($(element).attr("name")) { return "[name=" + $(element).attr("name") + "]"; } else { return "." + $(element).attr("class").replace(/ /g, '.'); } } return "<" + $(element)[0].tagName + "/> " + ident() + (!value ? "" : ": " + $(element).val()); }, onvalid: function (callback) { if (callback) { handler = callback; } else { return handler; } } }
This awesome jQuery plugin is developed by paulwardrip. For more Advanced Usages, please check the demo page or visit the official website.