Tiny Client-side Form Validator - jQuery Poppa
File Size: | 25.7 KB |
---|---|
Views Total: | 932 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
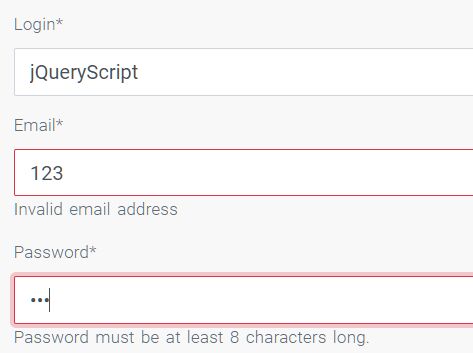
Poppa is an easy, tiny, cross-browser, client-side jQuery form validation plugin which makes it simple to validate existing form fields using built-in and custom validation rules.
Built-in validators:
- Required
- Length
- Range
- Url
- Alphanumeric
- Letters
- Regexp
More features:
- Validates form fields on submit.
- Or validates form fields when typing in the input or the field looses focus.
- Prevents your form from submitting untile all the fields are valid.
- Allows you to turn off the native autocomplete functionality.
- Allows you to disable the native HTML5 form validation.
- Custom hints and error messages.
How to use it:
1. Download and import the Poppa plugin's files into the document which has the latest jQuery library loaded.
<link rel="stylesheet" href="/path/to/validation.css" /> <script src="/path/to/jquery.min.js"></script> <script src="/path/to/poppa.js"></script>
2. Add validators to form fields and customize the hint/error messages using the following data
attributes:
<form action="" class="example"> <label>Name *</label> <input name="password" data-validation-type="letters" required> <label>Login *</label> <input name="login" type="text" data-validation-type="alphanumeric" data-validation-length="min:3,max:35" required> <label>Email *</label> <input name="email" type="text" data-validation-type="email" required> <label>Password *</label> <input name="password" data-validation-type="password" required> <label>Website</label> <input name="website" data-validation-type="url"> <label>Custom Validator</label> <input type="text" data-validation-regexp="^[A-Z]+$" required> <label>Bio</label> <textarea name="bio" data-validation-length="min:10,max:150" data-validation-hint="Describe yourself"></textarea> <label>I agree to the terms of service *</label> <input type="checkbox" data-validation-message="You have to agree to terms of service" required> <button type="submit">Submit</button> </form>
3. Enable the Poppa form validation plugin on the HTML form and done.
$(function(){ $('.example').validation(); });
4. Possible plugin options to customize the form validation plugin.
$('.example').validation({ // whether to prevents form from submitting when invalid preventDefault: true, // enable/disable autocomplete autocomplete: 'on', // prevents form from submitting when valid sendForm: true, // enable/disable live validation liveValidation: true, // enable/disable HTML5 form validation noValidate: true, // Live validation must be set to true to make this option work. disableSubmitInvalid: false, // warning message when invalid formInvalidAlertWarning: 'The form has not been completed correctly. Correct marked fields.' });
This awesome jQuery plugin is developed by eceterak. For more Advanced Usages, please check the demo page or visit the official website.