Set & Get Input/Select Values With jQuery - xVal.js
File Size: | 23.4 KB |
---|---|
Views Total: | 1036 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
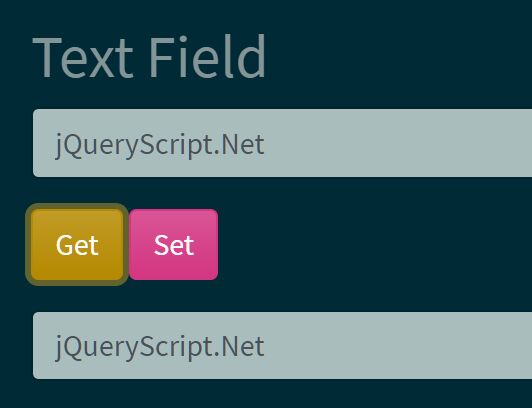
xVal.js is a small yet useful jQuery plugin that enables you to get and set the values of form elements: input field, radio button, checkbox input, single/multiple select.
How to use it:
1. Load the main script jquery.xval.js
after jQuery library and we're ready to go.
<script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/jquery.xval.js"></script>
2. Get/set the value of an input field.
<input type="text" class="text-input" name="text"> <input class="text-value" type="text">
// Get $('.text-value').xval($('.text-input').xval()); // Set $('.text-input').xval($('.text-value').xval(), { event: 'mychange', args: ['test'] } ); // event handler $('.text-input').on('mychange', function(e, ...args) { console.info('mychange text', this, this.value, ...args); });
3. Get/set the value of a group of radio buttons.
<label><input type="radio" class="radio-input" name="radio" value="1"> One</label> <label><input type="radio" class="radio-input" name="radio" value="2"> Two</label> <label><input type="radio" class="radio-input" name="radio" value="3"> Three</label> <input class="radio-value" type="text">
// Get $('.radio-value').xval($('.radio-input').xval()); // Set $('.radio-input').xval($('.radio-value').xval()); // Event handler $('.radio-input').on('change', function() { console.info('change radio', this, this.value); });
4. Get/set the value of a group of checkbox inputs.
<label><input type="checkbox" class="checkbox-input" name="checkbox" value="1"> One</label> <label><input type="checkbox" class="checkbox-input" name="checkbox" value="2"> Two</label> <label><input type="checkbox" class="checkbox-input" name="checkbox" value="3"> Three</label> <input class="checkbox-value" type="text">
// Get $('.checkbox-value').xval($('.checkbox-input').xval().join(', ')); // Set $('.checkbox-input').xval($('.checkbox-value').xval().split(/\s*\,\s*/)); // Event handler $('.checkbox-input').on('mychange', function() { console.info('mychange checkbox', this, this.value); });
5. Set/Get the value of a single select.
<select class="single-input" name="single"> <option value="1">One</option> <option value="2">Two</option> <option value="3">Three</option> </select> <input class="single-value" type="text">
// Get $('.single-value').xval($('.single-input').xval()); // Set $('.single-input').xval($('.single-value').xval()); // Event handler $('.single-input').on('change', function() { console.info('change single', this, this.value); });
6. Set/Get the value of a multiple select.
<select class="multiple-input" name="multiple" multiple> <option value="1">One</option> <option value="2">Two</option> <option value="3">Three</option> </select> <input class="multiple-value" type="text">
// Get $('.multiple-value').xval($('.multiple-input').xval().join(', ')); // Set $('.multiple-input').xval($('.multiple-value').xval().split(/\s*\,\s*/)); // Event handler $('.multiple-input').on('change', function() { console.info('change multiple', this, this.value); });
This awesome jQuery plugin is developed by nikitanickzhukov. For more Advanced Usages, please check the demo page or visit the official website.