Multi-Level Off-canvas Navigation with jQuery and CSS3 - Off-canvas
File Size: | 109 KB |
---|---|
Views Total: | 8484 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
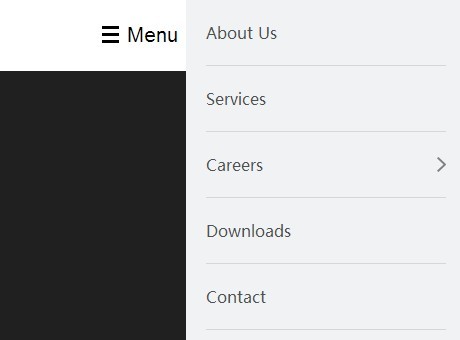
Yet another jQuery based responsive off-canvas navigation that supports multi-level menus and CSS3 powered sliding animations.
How to use it:
1. Create a button to toggle the off-canvas navigation.
<button class="button-nav-toggle"><span class="icon">☰</span> Menu</button>
2. Create a multi-level navigation menu using nested Html lists.
<div class="menu"> <nav class="nav-main"> <div class="nav-container"> <ul> <li><a href="">About Us</a></li> <li><a href="">Services</a></li> <li> <a href="#">Careers</a> <ul> <li><a href="#" class="back">Main Menu</a></li> <li class="nav-label"><strong>JOBS IN AMERICA</strong></li> <li><a href="">Accountant</a></li> <li><a href="">Budget Analyst</a></li> <li><a href="">Carpenter</a></li> <li><a href="">Desktop Publisher</a></li> <li><a href="">Electrical Engineer</a></li> <li><a href="">Financial Analyst</a></li> <li><a href="">Human Resources Specialists</a></li> <li><a href="">Loan Officer</a></li> <li><a href="">Reporter</a></li> <li class="nav-label"><strong>JOBS IN GERMANY</strong></li> <li><a href="">Budget Analyst</a></li> <li><a href="">Carpenter</a></li> <li><a href="">Electrical Engineer</a></li> <li><a href="">Financial Analyst</a></li> <li><a href="">Human Resources Specialists</a></li> <li><a href="">Loan Officer</a></li> </ul> </li> <li><a href="">Downloads</a></li> <li><a href="">Contact</a></li> </ul> </div> </nav> </div>
3. The core CSS/CSS3 snippets to style & animate the off-canvas navigation.
.button-nav-toggle { font-size: 1.3em; float: right; border: 0; background: 0 0; color: #000; outline: 0; cursor: pointer } .nav-main { transition: right .25s ease-in-out; background: #f1f2f3; position: fixed; overflow-x: hidden; width: 280px; right: -280px; top: 0; bottom: 0 } .nav-main .nav-container { transition: left .25s ease-in-out; position: relative; left: 0 } .nav-main .nav-container.show-sub { left: -280px } .nav-main .nav-container ul { margin: 0; padding: 0 20px } .nav-main .nav-container ul li { list-style: none; border-bottom: 1px solid #d5d7da } .nav-main .nav-container ul li a { text-decoration: none; display: block; padding: 20px 0; color: #4d5152; font-size: 16px } .nav-main .nav-container ul li a:hover { color: #4d5152 } .nav-main .nav-container ul li ul { display: none; width: 280px; position: absolute; right: -280px; top: 0 } .nav-main .nav-container ul li ul li { border: 0 } .nav-main .nav-container ul li ul li.nav-label { border-top: 1px solid #d5d7da; padding: 25px 0 10px } .nav-main .nav-container ul li ul li a { padding: 7px 0 15px; font-size: 14px } .nav-main .nav-container ul li ul li a.back { font-size: 16px; font-weight: 700; text-transform: uppercase; padding: 20px 25px; background: url(img/arrow-left.png) left center no-repeat } .nav-main .nav-container ul li.has-sub-nav a { background: url(img/arrow-right.png) right center no-repeat } .nav-main .nav-container ul li.has-sub-nav ul a { background: 0 0 } .nav-main .nav-container ul li.active ul { display: block } .open .nav-main { right: 0 }
4. The Javascript to active the off-canvas navigation. Make sure to load the following JS snippet after the jQuery library.
// import jQuery library $(document).ready(function(){ $(".button-nav-toggle").click(function(){ $(".main").toggleClass("open"); $(".menu").toggleClass("open"); }); $(".nav-main li:has(ul)").addClass("has-sub-nav").prepend("<div class=\"sub-toggle\"></div>"); $(".has-sub-nav a").click(function(){ $(this).parent().addClass("active"); $(".nav-container").addClass("show-sub"); }); $(".has-sub-nav .back").click(function(){ $(".nav-container").removeClass("show-sub"); $(".has-sub-nav").removeClass("active"); }); });
This awesome jQuery plugin is developed by birkmann. For more Advanced Usages, please check the demo page or visit the official website.