Minimal Form Field Validation Plugin For jQuery - Validin
File Size: | 23.2 KB |
---|---|
Views Total: | 5456 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
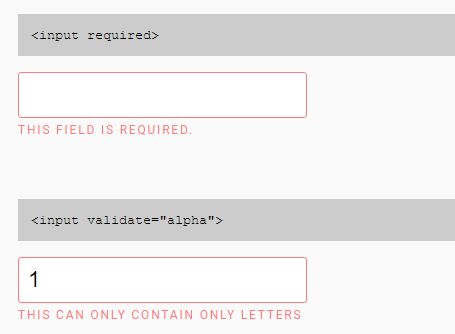
Validin is a lightweight, easy-to-use jQuery plugin used to validate various types of form fields using regular expressions.
Built-in validate rules:
- alpha: only letters
- alpha_num: letters and numbers
- alpha_space: letters
- alpha_dash: letters, underscores and hyphens
- alpha_num_dash: letters, numbers, underscores and hyphens
- number: valid whole number
- decimal: valid number
- name: valid name
- email: valid email address
- securepassword: strong password
- url: valid URL
- phone: valid phone number
- zip: valid zip code
- creditcard: valid credit card number
- min: at least %i
- ma: no more than %i
- min_length: at least %i characters long
- max_length: no more than %i characters long
- contain: contain specific strings
- match: These values have to match
How to use it:
1. To use this plugin, you first need to load the JavaScript file 'validin.js' after you've loaded jQuery library:
<script src="//code.jquery.com/jquery.min.js"></script> <script src="validin.js"></script>
2. Call the function on the target form element and the jQuery Validin is ready for use.
$('form').validin();
3. Add the validate="Rule Name"
attribute to the form fields as these:
<form> <input required> <input validate="alpha"> <input validate="alpha_num"> <input validate="alpha_space"> <input validate="alpha_dash"> <input validate="alpha_num_dash"> <input validate="number"> <input validate="decimal"> <input validate="name"> <input validate="email"> <input validate="url"> <input validate="phone"> <input validate="zip"> <input validate="creditcard"> <input validate="min:5"> <input validate="max:5"> <input validate="min_length:5"> <input validate="max_length:5"> <input class="must_match"> <input validate="match:.must_match"> <input type="submit"> </form>
3. You can also define your own validation rules using regex.
<input validate="regex:/[0-9a-z]{1,3}-\d*/i">
4. Style the error message and form fields when invalid.
input.invalid { border-color: #ff7a7a; } .validation_error { margin: .4em 0 1em; color: #ff7a7a; font-size: .7em; text-transform: uppercase; letter-spacing: .15em; }
5. Customize the default validation rules and error messages.
$('form').validin({ validation_tests: { 'alpha': { 'regex': /[a-zA-Z]*/, 'error_message': "This can only contain only letters" }, 'alpha_num': { 'regex': /[A-Z0-9]*/i, 'error_message': "This can only contain letters and numbers" }, 'alpha_space': { 'regex': /[A-Z ]*/i, 'error_message': "This can only contain letters" }, 'alpha_dash': { 'regex': /[A-Z\.\-_]*/i, 'error_message': "This can only contain letters, underscores and hyphens" }, 'alpha_num_dash': { 'regex': /[A-Z0-9\.\-_]*/i, 'error_message': "This can only contain letters, numbers, underscores and hyphens" }, 'number': { 'regex': /[\d]*/, 'error_message': "This needs to be a valid whole number" }, 'decimal': { 'regex': /(\d*\.?\d*)/, 'error_message': "This needs to be a valid number" }, 'name': { 'regex': /[A-Z\.\-'\s]*/i, 'error_message': "This needs to be a valid name" }, 'email': { 'regex': /[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,4}/i, 'error_message': "This needs to be a valid email address" }, 'url': { 'regex': /(https?|ftp):\/\/[^\s\/$.?#].[^\s]*/i, 'error_message': "This needs to be a valid URL" }, 'phone': { 'regex': /(?=.*?\d{3}( |-|.)?\d{4})((?:\+?(?:1)(?:\1|\s*?))?(?:(?:\d{3}\s*?)|(?:\((?:\d{3})\)\s*?))\1?(?:\d{3})\1?(?:\d{4})(?:\s*?(?:#|(?:ext\.?))(?:\d{1,5}))?)\b/i, 'error_message': "This needs to be a valid phone number" }, 'zip': { 'regex': /\d{5}(?:-?\d{4})?/i, 'error_message': "This needs to be a valid zip code" }, 'creditcard': { 'regex': /(?:4[0-9]{12}(?:[0-9]{3})?|5[1-5][0-9]{14}|6(?:011|5[0-9][0-9])[0-9]{12}|3[47][0-9]{13}|3(?:0[0-5]|[68][0-9])[0-9]{11}|(?:2131|1800|35\d{3})\d{11})/i, 'error_message': "This needs to be a valid credit card number" }, 'regex': { 'regex': /.*/i, 'error_message': "This is not a valid value" }, 'min': { 'regex': /.*/i, 'error_message': "This number needs to be at least %i" }, 'max': { 'regex': /.*/i, 'error_message': "This number needs to no more than %i" }, 'min_length': { 'regex': /.*/i, 'error_message': "This needs to be at least %i characters long" }, 'max_length': { 'regex': /.*/i, 'error_message': "This needs to be no more than %i characters long" }, 'match': { 'regex': /.*/i, 'error_message': "These values have to match" } }, });
6. More configuration options with default values.
$('form').validin({ // delay time in milliseconds feedback_delay: 700, // default CSS classes invalid_input_class: 'invalid', error_message_class: "validation_error", // default error messages form_error_message: "Please fix any errors in the form", required_fields_initial_error_message: "Please fill in all required fields", required_field_error_message: "This field is required", // adjust margins on the validation error messages override_input_margins: true, // additional validate rules tests: {}, // callback onValidateInput: function() {} });
Changelog:
2023-11-03
- Added addError() and removeError() functions
2023-09-17
- Re-added code that catches empty required fields even before they've gotten focus
v0.2.0 (2023-09-08)
- Added 'securepassword' validation, updated number validations to allow negative values, updated vnAttachMessage() to attach messages to labels instead of inputs, restricted validations to :visible inputs, misc. bug fixes and better scoping of variables
2022-03-01
- Improved textarea handling (allow carriage returns), scoped more variables, replaced $ jQuery references
2022-02-28
- Several reliability improvements and minor updates for less common inputs
2021-03-28
- Fixed bug that forced submit when user pressed Enter in input field
2019-01-01
- Separated default options out, changed function names to sorta namespace them, fixed errors in code, added logo svg
2017-12-14
- Added support for checkbox and radio type inputs (using new getValue() function), added error message language as configurable options, updated readme and demo page content
2017-09-16
- Fixed issue where user could submit invalid form with 'enter' key, better handling of multiple forms on one page, 'match' elements now check and flag both fields when values aren't the same, submit key gives reason for not being enabled if required fields are present, updated demo page considerably
This awesome jQuery plugin is developed by thomhines. For more Advanced Usages, please check the demo page or visit the official website.