Feature-rich Autocomplete Dropdown Plugin - jQuery ajax-combobox
File Size: | 468 KB |
---|---|
Views Total: | 888 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
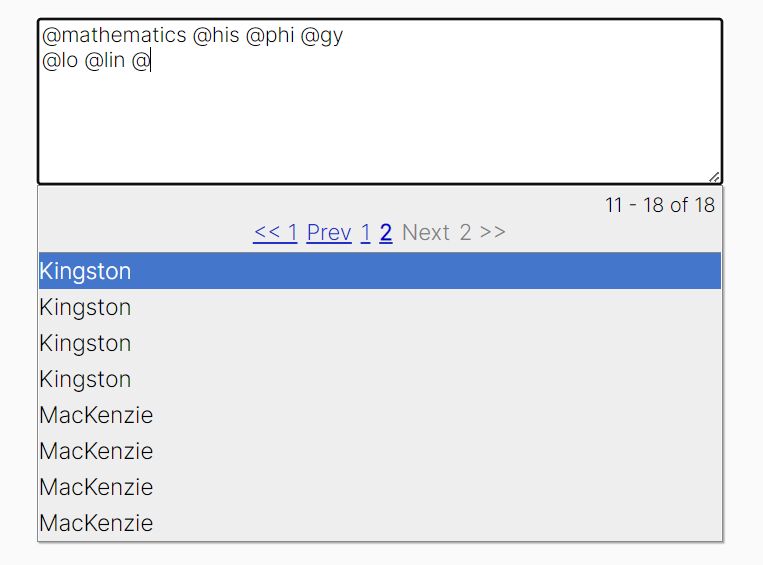
ajax-combobox is a feature-rich autocomplete dropdown plugin that uses jQuery, Ajax, PHP for adding a highly functional (pagination, filtering, sorting, keyboard navigation) autocomplete control to your text fields.
More Features:
- Fetches data from Database or JS Objects.
- Custom trigger characters (like @, #, etc) in textarea.
- Multiple languages.
- URL shorten.
How to use it:
1. To get started, load jQuery library and the ajax-combobox plugin's files in the document.
<link rel="stylesheet" href="/path/to/jquery.ajax-combobox.css" /> <script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/jquery.ajax-combobox.min.js"></script>
2. Attach the plugin to the target text field and specify the path to the PHP, which is used to fetch data from your database.
<input type="text" id="example" />
$('#example').ajaxComboBox('data.php');
<?php require_once('AjaxComboBox.php'); use myapp\AjaxComboBox; $mysql = array( 'dsn' => 'mysql:host=localhost;dbname=test;charset=utf8;port=3360', 'username' => 'root', 'password' => '' ); $sqlite = array( 'dsn' => 'sqlite:../../sample/sample.sqlite3', 'username' => '', 'password' => '' ); new AjaxComboBox($sqlite);
3. Or fetch data from an array of JS objects.
var data = [ {id:'A001',name:'Adams', post:'Sales', position:'The rank and file'}, {id:'A002',name:'Darling', post:'Sales', position:'The rank and file'}, {id:'A003',name:'Kingston', post:'General Affairs',position:'Chief clerk'}, {id:'A004',name:'Darling', post:'General Affairs',position:'Section chief'}, {id:'A005',name:'Adams', post:'Personnel', position:'The rank and file'}, {id:'A006',name:'Kingston', post:'Sales', position:'Director'}, {id:'A007',name:'Kingston', post:'Sales', position:'Section chief'}, {id:'A008',name:'Darling', post:'Personnel', position:'Chief'}, {id:'A009',name:'Adams', post:'Personnel', position:'Chief'}, {id:'A010',name:'Adams', post:'General Affairs',position:'The rank and file'}, {id:'A011',name:'Darling', post:'General Affairs',position:'The rank and file'}, {id:'A012',name:'Kingston', post:'Sales', position:'The rank and file'}, {id:'A013',name:'MacKenzie',post:'Sales', position:'Chief clerk'}, {id:'A014',name:'Darling', post:'Sales', position:'Vice-chief'}, {id:'A015',name:'MacKenzie',post:'General Affairs',position:'Vice-chief'}, {id:'A016',name:'Kingston', post:'Personnel', position:'Director'}, {id:'A017',name:'MacKenzie',post:'Personnel', position:'Section chief'}, {id:'A018',name:'MacKenzie',post:'Sales', position:'Chief'} ];
3. Or fetch data from an array of JS objects.
var data = [ {id:'A001',name:'Adams', post:'Sales', position:'The rank and file'}, {id:'A002',name:'Darling', post:'Sales', position:'The rank and file'}, {id:'A003',name:'Kingston', post:'General Affairs',position:'Chief clerk'}, {id:'A004',name:'Darling', post:'General Affairs',position:'Section chief'}, {id:'A005',name:'Adams', post:'Personnel', position:'The rank and file'}, {id:'A006',name:'Kingston', post:'Sales', position:'Director'}, {id:'A007',name:'Kingston', post:'Sales', position:'Section chief'}, {id:'A008',name:'Darling', post:'Personnel', position:'Chief'}, {id:'A009',name:'Adams', post:'Personnel', position:'Chief'}, {id:'A010',name:'Adams', post:'General Affairs',position:'The rank and file'}, {id:'A011',name:'Darling', post:'General Affairs',position:'The rank and file'}, {id:'A012',name:'Kingston', post:'Sales', position:'The rank and file'}, {id:'A013',name:'MacKenzie',post:'Sales', position:'Chief clerk'}, {id:'A014',name:'Darling', post:'Sales', position:'Vice-chief'}, {id:'A015',name:'MacKenzie',post:'General Affairs',position:'Vice-chief'}, {id:'A016',name:'Kingston', post:'Personnel', position:'Director'}, {id:'A017',name:'MacKenzie',post:'Personnel', position:'Section chief'}, {id:'A018',name:'MacKenzie',post:'Sales', position:'Chief'} ];
$('#example').ajaxComboBox(Data);
4. Display extra info when hovering over entries.
$('#example').ajaxComboBox(Data,{ sub_info: true, sub_as: { id: 'Employer ID', post: 'Post', position: 'Position' }, select_only: true, init_record: 'A009', primary_key: 'id', });
5. Attach the plugin to a textarea and specify the trigger characters as follows. Ideal for #tag & @mention autocomplete.
$('#example').ajaxComboBox(Data,{ plugin_type: 'textarea', tags: [ { pattern: ['@', ''] } ] });
6. All default options.
$('#example').ajaxComboBox(Data,{ // data source source: source, // en, es, pt-br and ja lang: 'en', // 'combobox', 'simple', 'textarea' plugin_type: 'combobox', // value of primary-key for initial value init_record: false, // database settings db_table: 'tbl', field: 'name', and_or: 'AND', // search field search_field: 'name', // number of entries per page per_page: 10, // number of pagination links navi_num: 5, // primary key primary_key: 'id', // 'name DESC', ['name ASC', 'age DESC'] order_by: 'name', // dropdown icon button_img: '<svg class="octicon octicon-chevron-down" viewBox="0 0 10 16" version="1.1" aria-hidden="true"><path fill-rule="evenodd" d="M5 11L0 6l1.5-1.5L5 8.25 8.5 4.5 10 6z"></path></svg>', // event to fire after selection bind_to: false, // enable simple navigation navi_simple: false, // Sub-info sub_info: false, sub_as: {}, show_field: '', hide_field: '', // Select-only mode select_only: false, // enable tags tags: { pattern: null, space: [true, true], db_table: 'tbl', field: 'name', order_by: 'name', search_field: 'name', sub_info: false, sub_as: {}, show_field: '', hide_field: '', }, // Shorten URL service shorten_btn: false, shorten_src: 'dist/bitly.php', shorten_min: 20, shorten_reg: false });
This awesome jQuery plugin is developed by sutara79. For more Advanced Usages, please check the demo page or visit the official website.