Form Generator With JSON Schema - jQuery jsForm
File Size: | 353 KB |
---|---|
Views Total: | 2741 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
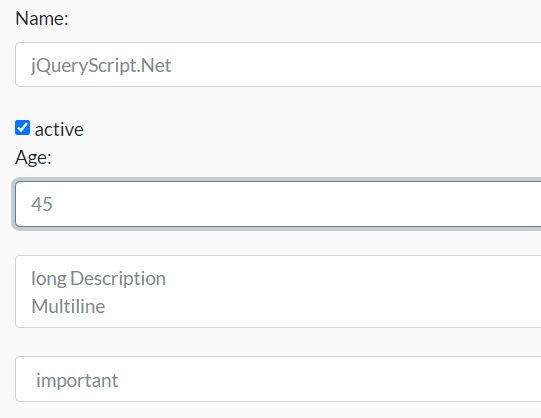
jsForm is a feature-rich JSON To Form generator that takes data from any JS objects and populates the form field with that data.
More Features:
- Form Validation.
- Data formatting.
- AJAX form submitting.
See Also:
Basic Usage:
1. Include both jQuery library and the jsForm plugin's script on the page.
<!-- jQuery --> <script src="/path/to/cdn/jquery.min.js"></script> <!-- Core --> <script src="/path/to/src/jquery.jsForm.js"></script> <!-- OPTIONAL. UI Controls and Field validation --> <script src="/path/to/src/jquery.jsForm.controls.js"></script>
2. Define your form data in a JS object as follows:
var jsonData = { name: "jQueryScript", // standard inputs props: { active: true, // checkbox groups: [{ name: "Base", priority: 0, tasks: [{ id:0, name: "base task1", priority: 1}, {id:1,name: "base task0", priority: 0}, {id:2,name: "base task2", priority: 2}] }, { name: "Important", priority: 2, tasks: [{id:0, name: "imp task1", priority: 1}, {id:1,name: "imp task0", priority: 0}] }, { name: "Other", priority: 1, tasks: [{id:0, name: "other", priority: 1}] }] }, state: "VISIBLE" // selects (enums) };
3. Initialize the plugin and fill the HTML form with the data you just defined.
<div id="details"> Name: <input name="data.name"/><br/> <input type="checkbox" name="data.props.active"/> active<br/> <select name="data.state"> <option value="VISIBLE">visible</option> <option value="IMPORTANT">important</option> <option value="HIDDEN">hidden</option> </select> <div class="collection sort" data-field="data.props.groups" data-sort="priority"> <fieldset> <legend>Group: <span class="field">groups.name</span></legend> <ul class="collection sort reorder" data-field="groups.tasks" data-sort="priority"> <li><input name="tasks.name"/></li> </ul> <button class="sortUp">up</button> <button class="sortDown">down</button> </fieldset> </div> </div>
$("#details").jsForm({ data:jsonData });
4. Available plugin options.
$("#details").jsForm({ /** * enable form control rendering (if jsForm.controls is available) and validation */ controls: true, /** * the object used to fill/collect data */ data: null, /** * the prefix used to annotate the input fields */ prefix: "data", /** * set to null to discourage the tracking of "changed" fields. * Disabling this will increase performance, but disabled the "changed" functionality. * This will add the given css class to changed fields. */ trackChanges: "changed", /** * set to false to only validate visible fields. * This is discouraged especially when you have tabs or similar elements in your form. */ validateHidden: true, /** * skip empty values when getting an object */ skipEmpty: false, /** * an object with callback functions that act as renderer for data fields (class=object). * ie. { infoRender: function(data){return data.id + ": " + data.name} } */ renderer: null, /** * an object with callback functions that act as pre-processors for data fields (class=object). * ie. { idFilter: function(data){return data.id} } */ processors: null, /** * dataHandler will be called for each field filled. */ dataHandler: null, /*{ serialize: function(val, field, obj) { if(field.hasClass("reverse")) return val.reverse(); }, deserialize: function(val, field, obj) { if(field.hasClass("reverse")) return val.reverse(); } }*/ /** * optional array of elements that should be connected with the form. This * allows the splitting of the form into different parts of the dom. */ connect: null, /** * The class used when calling preventEditing. This will replace all * inputs with a span with the given field */ viewClass: "jsfValue" });
5. API methods.
// Deserialize the object based on the form $("#details").jsForm("get"); // Get the data object $("#details").jsForm("getData"); // Prevent editing $("#details").jsForm("preventEditing", [true|false]); // Fill the form $("#details").jsForm("fill", data); // Clear the form $("#details").jsForm("clear"); // Reset the form $("#details").jsForm("reset"); // Check if the form has been changed $("#details").jsForm("changed"); // Compare data $("#details").jsForm("equals", data[, "idField"]);
Changelog:
v1.5.5 (2023-07-22)
- Update
v1.5.4 (2021-06-27)
- Update to 1.5.4 with luxon support
v1.5.2 (2021-06-24)
- add boolean handling
v1.5.1 (2021-06-03)
- fix issue with collections
This awesome jQuery plugin is developed by corinis. For more Advanced Usages, please check the demo page or visit the official website.