Animated Off-canvas Side Menu using jQuery and CSS3 Transitions
File Size: | 2.06 KB |
---|---|
Views Total: | 15353 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
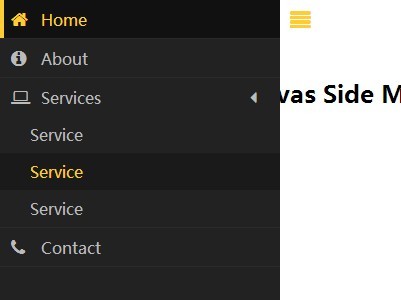
A mobile App style off-canvas navigation menu using CSS3 transitions and a little bit of JavaScript (jQuery).
How to use it:
1. Include the Font Awesome icon font for the navigation icons.
<link href="//netdna.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet" >
2. Create a vertical side menu from a nested nav
list.
<nav id="menu" class="left"> <ul> <li><a href="#" class="active"><i class="fa fa-home"></i>Home</a></li> <li><a href="#"><i class="fa fa-info-circle"></i>About</a></li> <li> <a href="#"><i class="fa fa-laptop"></i>Services <i class="fa fa-caret-down"></i></a> <ul> <li><a href="#">Service</a></li> <li><a href="#">Service</a></li> <li><a href="#">Service</a></li> </ul> </li> <li><a href="#"><i class="fa fa-phone"></i>Contact</a></li> </ul> <a href="#" id="showmenu"> <i class="fa fa-align-justify"></i> </a> </nav>
3. The core CSS styles for the side menu.
#menu { position: fixed; background-color: #222; height: 100%; z-index: 10; width: 280px; color: #bbb; top: 0; -webkit-transition: all 0.3s ease; -moz-transition: all 0.3s ease; transition: all 0.3s ease; opacity: 1; } #menu ul { list-style: none; margin-top: 0; padding: 0 } #menu ul li { border-bottom: 1px solid #2a2a2a; } #menu>ul>li>a { border-left: 4px solid #222; } #menu ul li a { color: inherit; font-size: 16px; display: block; padding: 8px 0 8px 7px; text-decoration: none; -webkit-transition: all 0.3s ease; -moz-transition: all 0.3s ease; transition: all 0.3s ease; font-weight: 600; } #menu ul a i { margin-right: 10px; font-size: 18px; margin-top: 3px; width: 20px; } #menu ul a i[class*='fa-caret'] { float: right; } #menu ul a:hover, #menu ul a.active { background-color: #111; border-left-color: #FFCC33; color: #FFCC33; } #menu ul a:hover i:first-child { color: #FFCC33; }
4. The CSS to style the sub menus.
#menu ul li a.active+ul { display: block } #menu ul li ul { margin-top: 0; display: none; } #menu ul li ul li { border-bottom: none; } #menu ul li ul li a { padding-left: 30px; } #menu ul li ul li a:hover { background-color: #1A1A1A; }
5. The CSS to turn the side menu into an off-canvas navigation that is toggleable via JavaScript.
.left { left: -280px; } .show { left: 0; }
6. Style & position the toggle button whatever you like.
#showmenu { margin-left: 100%; position: absolute; top: 0; padding: 6px 10px 7px 10px; font-size: 1.3em; color: #FFCC33; -webkit-transition: all 0.3s ease; -moz-transition: all 0.3s ease; transition: all 0.3s ease; }
7. The JavaScript to active the off-canvas side menu. Make sure to place the following JS snippets after you have jQuery library included.
$("#showmenu").click(function(e){ e.preventDefault(); $("#menu").toggleClass("show"); }); $("#menu a").click(function(event){ event.preventDefault(); if($(this).next('ul').length){ $(this).next().toggle('fast'); $(this).children('i:last-child').toggleClass('fa-caret-down fa-caret-left'); } });
This awesome jQuery plugin is developed by romanll. For more Advanced Usages, please check the demo page or visit the official website.